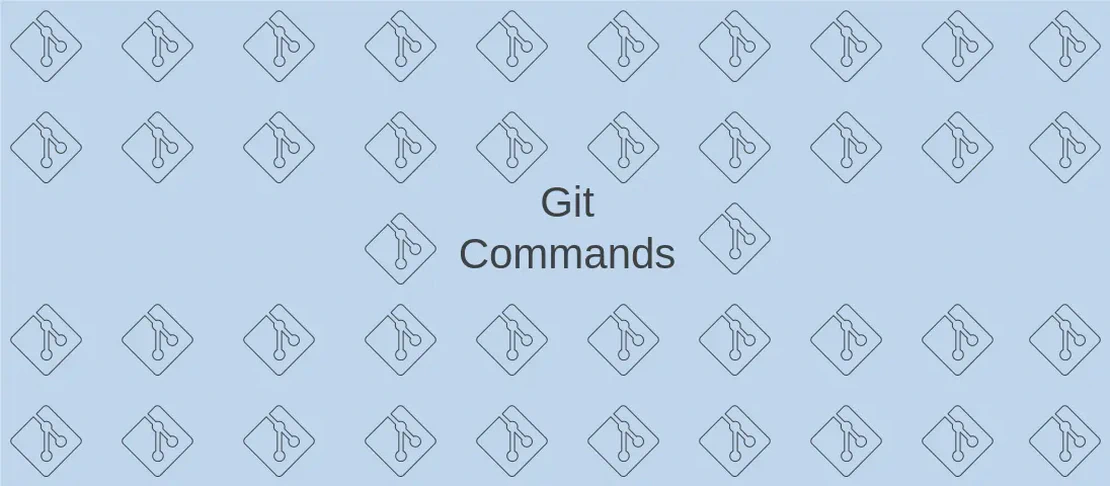
How to use the command 'git-grep' (with examples)
Git-grep is a command in Git that allows users to search for specific strings inside files anywhere in a repository’s history. It functions similarly to the regular Unix command ‘grep’, but it has the added advantage of being able to search through the entire history of a project.
Use case 1: Search for a string in tracked files
Code:
git grep search_string
Motivation:
When working on a project, it is often necessary to search for specific strings within tracked files. This can be useful for finding particular functions, variable names, or debugging statements. By using the ‘git grep’ command, you can easily filter through the tracked files in your repository and locate the desired string.
Explanation:
git grep
: The command used to initiate the search.search_string
: The string that you want to search for.
Example output:
filename1: This line contains the search_string
filename2: No matches found
filename3: Another match with the search_string
This output shows the filenames where ‘search_string’ was found, along with the corresponding lines of code.
Use case 2: Search for a string in files matching a pattern in tracked files
Code:
git grep search_string -- file_glob_pattern
Motivation:
Sometimes, you may only want to search for a specific string within a subset of files in your repository. To accomplish this, you can specify a file glob pattern to filter the search only within those files matching the pattern. This can be particularly useful when searching for strings in a specific type of file, such as “*.py” for Python files.
Explanation:
git grep
: The command used to initiate the search.search_string
: The string to search for.--
: Separates the options from the file glob pattern.file_glob_pattern
: A pattern that specifies the files to be searched. This can include wildcard characters like ‘*’, ‘?’ to match different filenames or extensions.
Example output:
filename1: This line contains the search_string
This output only shows the filename and line where the ‘search_string’ was found in a file matching the specified pattern.
Use case 3: Search for a string in tracked files, including submodules
Code:
git grep --recurse-submodules search_string
Motivation:
When working with submodules in Git, it can be useful to search for specific strings within both the main repository and its submodules. By using the ‘–recurse-submodules’ option with the ‘git grep’ command, you can search within both the repository and its submodules, saving time and effort.
Explanation:
git grep
: The command used to initiate the search.--recurse-submodules
: An option that enables searching within submodules as well.search_string
: The string to search for.
Example output:
filename1: This line contains the search_string
submodule/filename2: Another match with the search_string
This output displays the filenames in both the repository and its submodules where the ‘search_string’ was found.
Use case 4: Search for a string at a specific point in history
Code:
git grep search_string HEAD~2
Motivation:
When debugging or analyzing changes in a project, it can be useful to search for a specific string at a particular point in the project’s history. By specifying the desired commit with ‘HEAD~2’, you can search for the string in the files as they were in that state.
Explanation:
git grep
: The command used to initiate the search.search_string
: The string to search for.HEAD~2
: The commit reference indicating the point in history where you want to search.
Example output:
filename1: This line contains the search_string
This output shows the filename and line where the ‘search_string’ was found in the files at the specified commit.
Use case 5: Search for a string across all branches
Code:
git grep search_string $(git rev-list --all)
Motivation:
When working on a large project with multiple branches, it can be convenient to search for a particular string across all the branches. By using the ‘git rev-list –all’ command to get a list of all the commits in the repository’s history, you can then search for the desired string in the files associated with those commits.
Explanation:
git grep
: The command used to initiate the search.search_string
: The string to search for.$(git rev-list --all)
: Command substitution that expands to a list of all commit references in the repository’s history.
Example output:
filename1: This line contains the search_string
filename2: Another match with the search_string
This output displays the filenames where the ‘search_string’ was found across all branches in the repository.