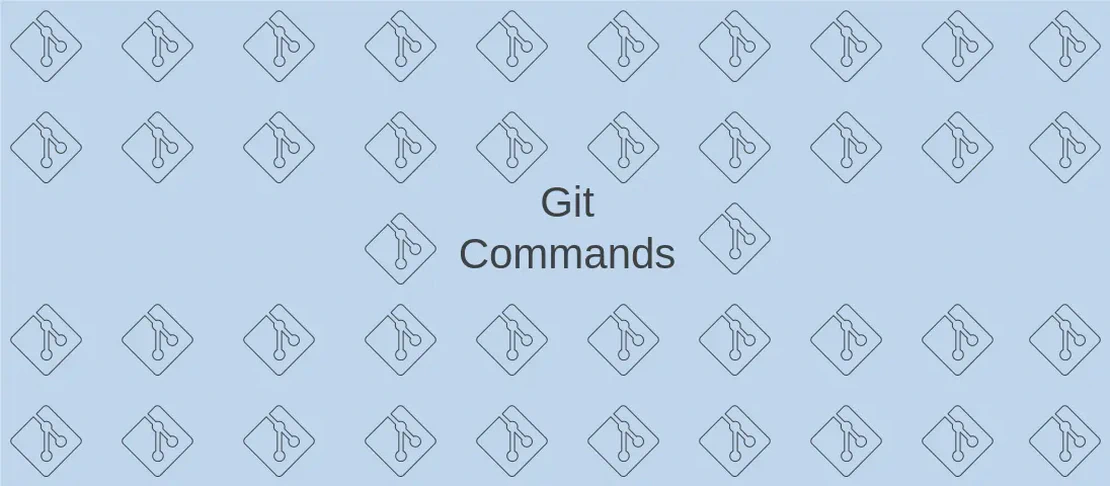
How to use the command 'git lfs' (with examples)
Git LFS (Large File Storage) is an extension to Git that is designed to improve the handling of large files and binary files in Git repositories. By storing large files outside the main Git repository, Git LFS helps reduce the overall size of the repository and improves performance during cloning, fetching, and other processes. This is particularly useful for developers dealing with large media files, massive datasets, or any other substantial files that need to be managed efficiently within version control. The following sections provide practical examples and detailed explanations on how to use various commands in Git LFS.
Initialize Git LFS - Use case 1
Code:
git lfs install
Motivation:
Initial setup is crucial when starting to work with Git LFS in a repository. The git lfs install
command ensures that the necessary hooks and configurations are added to the Git environment to manage large files effectively. This is a one-time setup step that prepares the repository to use Git LFS by modifying the .git/config
file to enable automatic downloading of LFS-managed files.
Explanation:
The install
sub-command initializes the current repository to use Git LFS by configuring required LFS hooks and settings. This ensures that any future interactions will account for large file handling, permitting seamless integration as LFS tracks and manages files.
Example Output:
Git LFS initialized.
Track files that match a glob - Use case 2
Code:
git lfs track '*.bin'
Motivation:
Tracking specific types of large files, such as binaries, is essential for reducing the repository size and maintaining its performance. The git lfs track
command allows users to specify patterns or globs of file types that should be managed by Git LFS. This ensures that binary files, which are often large and undergo frequent changes, are stored efficiently without overloading the Git history.
Explanation:
The argument *.bin
is a glob pattern that matches all files with the .bin
extension. By using git lfs track '*.bin'
, you instruct Git LFS to track all files of this type. This pattern-based approach provides flexibility in managing a wide variety of file types without manually specifying each file.
Example Output:
Tracking "*.bin"
Change the Git LFS endpoint URL - Use case 3
Code:
git config -f .lfsconfig lfs.url lfs_endpoint_url
Motivation:
Sometimes, the server that hosts the Git LFS objects may be different from the primary Git server. In such cases, altering the endpoint URL is necessary to direct LFS operations towards the appropriate server. This ensures that large files are pushed to and fetched from the correct location, maintaining repository consistency and availability.
Explanation:
The -f .lfsconfig
flag specifies the configuration file (.lfsconfig
) where the LFS-specific settings are stored. The lfs.url
setting is adjusted to a new lfs_endpoint_url
, which is the URL of the separate LFS server. This modifies the LFS endpoint to guide where files should be managed.
Example Output:
There is typically no output, as this command updates configuration settings silently.
List tracked patterns - Use case 4
Code:
git lfs track
Motivation:
Understanding which file patterns are being tracked by Git LFS helps in auditing and verifying that all necessary files are managed properly. This command provides an overview of current LFS-managed file patterns, ensuring that no essential file types are left out by mistake.
Explanation:
This sub-command track
when used without additional arguments lists all the file patterns that are currently being tracked by Git LFS in the repository. It helps in verifying tracking rules and making necessary adjustments if needed.
Example Output:
Listing tracked patterns
*.bin
*.img
List tracked files that have been committed - Use case 5
Code:
git lfs ls-files
Motivation:
Once files are being managed by Git LFS, it’s important to see which specific files have been successfully committed under LFS management. This command gives insight into the files already handled by LFS, providing a comprehensive list of managed files as part of repository maintenance and tracking.
Explanation:
The ls-files
sub-command lists all files that have been committed under Git LFS. It provides details about the files such as the path and their size, confirming their inclusion in LFS management.
Example Output:
Listing LFS-managed files
1d3f4ef6c3 * file1.bin
c29c7a8b09 * file2.bin
Push all Git LFS objects to the remote server - Use case 6
Code:
git lfs push --all origin main
Motivation:
Pushing all Git LFS objects to the remote server ensures that every large file object tracked by LFS is uploaded, resolving any synchronization issues between the local repository and remote server. This is particularly useful when encountering errors like missing LFS objects during a push.
Explanation:
The --all
option forces all LFS objects to be pushed to the specified remote, origin
, and branch, main
. This comprehensive push ensures that every tracked large file residing in the local LFS cache is uploaded to the remote LFS server.
Example Output:
Uploading LFS objects
Uploading file1.bin [ => ] 50%...
Uploading file2.bin [===========> ] 75%...
Fetch all Git LFS objects - Use case 7
Code:
git lfs fetch
Motivation:
Fetching all LFS objects is essential when you need all the large files that have been added by anyone on your team. This command retrieves all necessary LFS objects for your current local repository state, ensuring no file is missing due to repository moves or changes.
Explanation:
The fetch
sub-command downloads all the LFS files for your current repository state, gathering every needed large file object to ensure consistent operation and availability in your local environment.
Example Output:
Fetching LFS objects
Checkout all Git LFS objects - Use case 8
Code:
git lfs checkout
Motivation:
After fetching all LFS objects, checking them out into the working directory ensures you can access the actual file content rather than placeholders. This step is necessary to effectively work with the files after a clone or fetch operation has occurred.
Explanation:
The checkout
sub-command replaces the pointer files in the working directory with the actual large file content from the local LFS cache, enabling users to interact directly with LFS-managed files.
Example Output:
Checking out LFS-managed files
Restored file1.bin
Restored file2.bin
Conclusion:
Git LFS improves the management of large files within Git repositories by providing efficient storage solutions and enhancing performance. From initializing Git LFS to managing endpoints, tracking patterns, and handling file objects, these examples illustrate the power of Git LFS in simplifying the complexity of working with large files. Adopting Git LFS practices ensures a balance between the utility of version control and the necessity of handling large datasets, thereby empowering developers to maintain high-performance repositories.