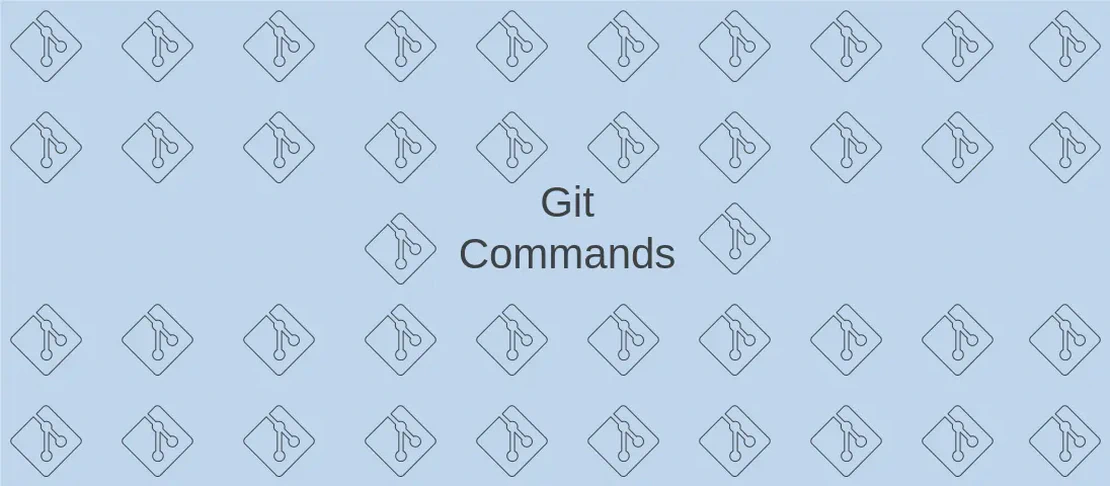
How to Use the Command 'git log' (with Examples)
The git log
command is an essential tool in the Git version control system, providing a comprehensive view of a repository’s history. It allows developers to traverse through the project’s commits, examine changes, and gain insights into the evolution of the codebase. By understanding how to effectively use git log
, developers can better manage their projects and collaborate more effectively. In this article, we explore different use cases of the git log
command with detailed examples.
Use Case 1: Show a Sequence of Commits
Code:
git log
Motivation: Understanding the progression of a project requires knowing its commit history. By displaying a reverse chronological sequence of commits, developers can quickly traverse through the timeline, identify significant changes, and understand the project’s evolution.
Explanation: The command git log
with no arguments shows a complete history of all commits in the current branch. It displays each commit’s hash, author, date, and commit message.
Example Output:
commit e34bc92faffedec23fe8f86e8f6bc38b94ab1013
Author: Jane Doe <jane@example.com>
Date: Mon Oct 1 10:00:00 2023 -0400
Implement feature X
commit f3a920fe8457ced230c5bd4d8f4b5423a72dc8ef
Author: John Smith <john@example.com>
Date: Sat Sep 30 14:30:00 2023 -0400
Fix bug Y
Use Case 2: Show the History of a Particular File or Directory, Including Differences
Code:
git log -p path/to/file_or_directory
Motivation: When you need to understand how a specific file or directory evolved over time, viewing its commit history and the specific changes made in each commit helps in identifying when bugs were introduced or features were implemented.
Explanation: The -p
option stands for “patch,” which shows the diffs for each commit affecting the specified file or directory. This provides detailed insight into what lines were changed, added, or removed.
Example Output:
commit 9e8b0ds89773d92eb0d8e12b3a111f60aaeef836
Author: Jane Doe <jane@example.com>
Date: Thu Sep 29 16:15:00 2023 -0400
Update config settings
diff --git a/config/settings.py b/config/settings.py
index 3b18b393..a29dd84 100644
--- a/config/settings.py
+++ b/config/settings.py
@@ -12,7 +12,7 @@
## Use Case 3: Show an Overview of Which Files Changed in Each Commit
Code:
```shell
git log --stat
Motivation: For a high-level overview of changes, understanding which files were altered and the extent of those changes (in terms of lines of code added or removed) can be incredibly useful for assessing the impact of each commit.
Explanation: The --stat
option presents a summary of the changes in each commit, including the number of lines added or removed for each file.
Example Output:
commit 654a44f0e51d8f517792e8f164daf156342bdbfa
Author: John Smith <john@example.com>
Date: Fri Sep 28 20:45:00 2023 -0400
Refactor authentication module
auth/user.py | 8 ++++----
auth/auth_helpers.py | 5 ++---
2 files changed, 6 insertions(+), 7 deletions(-)
Use Case 4: Show a Graph of Commits in the Current Branch Using Only the First Line of Each Commit Message
Code:
git log --oneline --graph
Motivation: Visualizing the branch structure and commit history succinctly helps developers quickly grasp how changes interrelate, especially in branches with many commits.
Explanation: The --oneline
option condenses each commit to its first line, and --graph
draws a text-based graph of the branch structure, showing parent-child relationships between commits.
Example Output:
* 654a44f (HEAD -> main) Refactor authentication module
* e34bc92 Implement feature X
* f3a920f Fix bug Y
Use Case 5: Show a Graph of All Commits, Tags, and Branches in the Entire Repo
Code:
git log --oneline --decorate --all --graph
Motivation: When working in a collaborative environment with multiple branches and tags, gaining an overall picture of the repository’s history and structure helps developers synchronize their work and predict the impact of their changes.
Explanation: --decorate
shows references to branches and tags, --all
displays the history across all branches, and the combination provides a comprehensive, graphical overview of the repository.
Example Output:
* 654a44f (HEAD -> main, tag: v1.0) Refactor authentication module
| * 3b5791c (feature-branch) Start new feature
|/
* e34bc92 Implement feature X
Use Case 6: Show Only Commits with Messages that Include a Specific String, Ignoring Case
Code:
git log --grep search_string --regexp-ignore-case
Motivation: Searching for commits associated with specific terms—such as bug identifiers or feature names—helps isolate relevant changes and accelerates troubleshooting or audits.
Explanation: The --grep
option filters commits based on a search string, while --regexp-ignore-case
allows case-insensitive searching.
Example Output:
(Assuming “feature x” is the search_string)
commit e34bc92faffedec23fe8f86e8f6bc38b94ab1013
Author: Jane Doe <jane@example.com>
Date: Mon Oct 1 10:00:00 2023 -0400
Implement feature X
Use Case 7: Show the Last N Number of Commits from a Certain Author
Code:
git log --max-count 3 --author "Jane Doe"
Motivation: Focusing on a specific contributor’s work helps review code, understand past contributions, or prepare for performance evaluations.
Explanation: --max-count
limits the log output to a set number of commits, while --author
filters commits by the given author’s name.
Example Output:
commit e34bc92faffedec23fe8f86e8f6bc38b94ab1013
Author: Jane Doe <jane@example.com>
Date: Mon Oct 1 10:00:00 2023 -0400
Implement feature X
commit 9e8b0ds89773d92eb0d8e12b3a111f60aaeef836
Author: Jane Doe <jane@example.com>
Date: Thu Sep 29 16:15:00 2023 -0400
Update config settings
Use Case 8: Show Commits Between Two Dates
Code:
git log --before "2017-01-29" --after "2017-01-17"
Motivation: Analyzing changes within a specific timeframe is crucial for project milestones and reports on development progress during sprints or other defined periods.
Explanation: The --before
and --after
options limit the commits to those made before and after the specified dates, respectively.
Example Output:
commit e19bf0d7ae89245235998423974f568d069835ff
Author: John Smith <john@example.com>
Date: Fri Jan 27 15:20:00 2017 -0400
Finalize documentation for release 1.0
commit 4d2a6h3bc45a2f23bd458f0a6d985bba493a1122
Author: Jane Doe <jane@example.com>
Date: Wed Jan 18 11:55:00 2017 -0400
Address feedback from code review
Conclusion:
The git log
command is a versatile tool that can cater to numerous scenarios depending on the information required from a Git repository’s history. By employing its various options and filters, developers can efficiently manage and review project progress, aiding in decision-making and collaboration efforts. Mastery of git log
and its use cases equips developers with the insights needed to maintain high-quality code throughout the software development lifecycle.