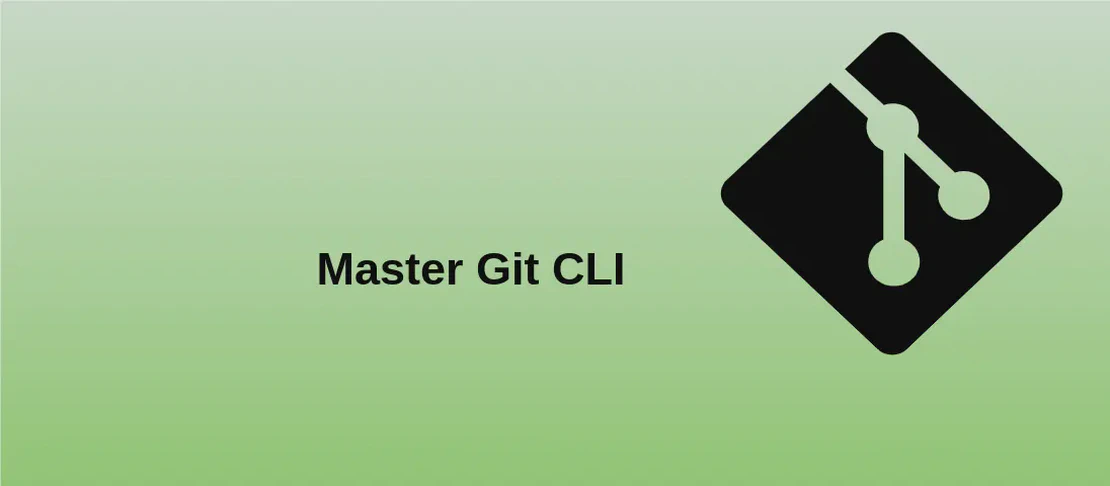
How to use the command git ls-files (with examples)
Git ls-files is a command that provides information about files in the index and the working tree. It allows users to view various aspects of the files, such as their status and whether they are tracked or untracked. This article will guide you through different use cases of the git ls-files
command with examples.
Use case 1: Show deleted files
Code:
git ls-files --deleted
Motivation: This use case is helpful when you want to see a list of files that have been deleted in your Git repository. It can be useful to confirm that the expected files have been removed, especially before committing the changes.
Explanation: The --deleted
option is used to filter the files and only display the deleted ones. This option limits the output to show only the files that have been deleted in the repository.
Example output:
deleted_file_1.txt
deleted_file_2.js
deleted_file_3.py
Use case 2: Show modified and deleted files
Code:
git ls-files --modified
Motivation: When working on a project, it is important to keep track of the modified and deleted files. This use case allows you to see a list of files that have been modified and deleted.
Explanation: The --modified
option filters the output to show only the files that have been modified or deleted in the repository. It excludes files that are unmodified.
Example output:
modified_file_1.txt
deleted_file_2.js
modified_file_3.py
Use case 3: Show ignored and untracked files
Code:
git ls-files --others
Motivation: While working with Git, it is common to have files that are ignored or not yet tracked. This use case allows you to view a list of ignored and untracked files in your Git repository.
Explanation: The --others
option filters the files to show only the ignored and untracked files. Ignored files are those specified in the .gitignore
file, while untracked files are those that have not been added to the Git repository.
Example output:
ignored_file_1.txt
untracked_file_2.js
untracked_file_3.py
Use case 4: Show untracked files, not ignored
Code:
git ls-files --others --exclude-standard
Motivation: Sometimes, you only want to see the list of untracked files that are not ignored. This use case allows you to filter out the ignored files and display a list of untracked files that should be considered for tracking.
Explanation: The --exclude-standard
option is used to exclude the standard ignore rules defined in the .gitignore
file. By combining it with the --others
option, the command displays only the untracked files that are not ignored.
Example output:
untracked_file_2.js
untracked_file_3.py
Conclusion
The git ls-files
command provides useful information about files in the index and the working tree of a Git repository. By utilizing the various options, such as --deleted
, --modified
, --others
, and --exclude-standard
, you can easily filter and view specific sets of files based on their status and ignore/tracking status. This command can be instrumental in managing your files and tracking their changes effectively.