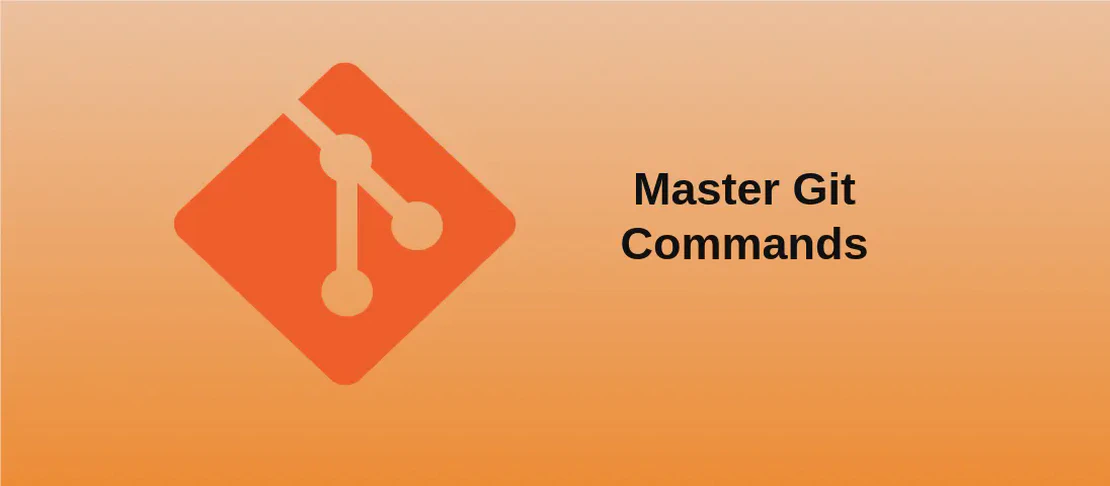
Exploring the 'git ls-tree' Command (with examples)
The git ls-tree
command is a versatile tool within the Git version control system that allows users to explore the contents of tree objects, which represent directories in a Git repository. It provides details about the files and directories at a specific point in the commit history, making it immensely useful for developers who need to navigate or inspect the state of a project at various stages of its development. The command supports several options to customize its behavior, enabling users to list file names, explore contents recursively, and more.
Use case 1: List the contents of the tree on a branch
Code:
git ls-tree branch_name
Motivation:
This use case is particularly valuable for developers who want to quickly check which files and directories are present on a specific branch. Whether you’re comparing branches, tracking changes, or just familiarizing yourself with a branch’s structure, listing the entirety of a branch’s contents can enhance your understanding and provide clarity without checking out the branch explicitly.
Explanation:
git
: The Git command-line tool.ls-tree
: The specific Git subcommand used to list tree objects.branch_name
: The name of the branch whose contents you’d like to list.
Example output:
100644 blob 5d41402abc4b2a76b9719d911017c592 README.md
100644 blob 8299cb40d5f4ea9fbf17ce739eb7356d index.js
040000 tree d670460b4b4aece5915caf5c68d12f560a9fe3e4 lib
Use case 2: List the contents of the tree on a commit, recursing into subtrees
Code:
git ls-tree -r commit_hash
Motivation:
When working with a large codebase, understanding all files affected by a particular commit can be essential for code reviews, debugging, or simply getting insights into historical changes. Recursively listing the contents of a commit allows developers to dive deeply into the structure without manually traversing directories.
Explanation:
git
: The Git command-line tool.ls-tree
: The Git subcommand for listing tree contents.-r
: The option to recursively explore subdirectories and list their contents.commit_hash
: The unique identifier (hash) of the commit you want to explore.
Example output:
100644 blob 2fd4e1c67a2d28fced849ee1bb76e7391b93eb12 README.md
100644 blob 3b18e09c1f8e973c52f7b9eeede3d9de6c0f6e86 src/app.js
100755 blob 7b7a53f7c1d2e9bc1e0c1f02f12bebb6a7ffb46e scripts/deploy.sh
Use case 3: List only the filenames of the tree on a commit
Code:
git ls-tree --name-only commit_hash
Motivation:
This is a streamlined approach when you only need to see file names without worrying about additional details like file modes or object IDs. This can be especially helpful in scripts or when logging changes where just the list of files is sufficient.
Explanation:
git
: The Git command-line tool.ls-tree
: The command used for listing tree contents.--name-only
: An option that specifies only the names of files should be listed, omitting details like the blob hash or permissions.commit_hash
: The hash of the commit whose filenames you want to list.
Example output:
README.md
src/app.js
scripts/deploy.sh
Use case 4: Print the filenames of the current branch head in a tree structure
Code:
git ls-tree -r --name-only HEAD | tree --fromfile
Motivation:
Visualizing your project directory in a structured tree format directly from the command line can significantly aid in understanding the hierarchy and overall layout, especially when working with complex codebases. This visualization can help you better manage and organize files within your repository.
Explanation:
git
: Invokes the Git tool.ls-tree
: Lists the contents of the tree.-r
: Recursively traverses subdirectories in the current branch head.--name-only
: Limits the output to filenames only.HEAD
: Refers to the latest commit on the current branch.tree --fromfile
: Thetree
command uses the output filenames to display them in a tree format. It’s important to note that this might not be supported on Windows by default without additional tools.
Example output:
.
├── README.md
├── src
│ └── app.js
└── scripts
└── deploy.sh
Conclusion:
The git ls-tree
command is a powerful utility for exploring and inspecting the contents of directories in Git repositories. Whether you are managing a project, reviewing historical changes, or visualizing your codebase’s structure, this command provides the necessary tools to navigate and understand the various states and versions of your files. Each use case outlined above offers unique capabilities tailored to specific workflows, making git ls-tree
an indispensable part of any developer’s toolkit.