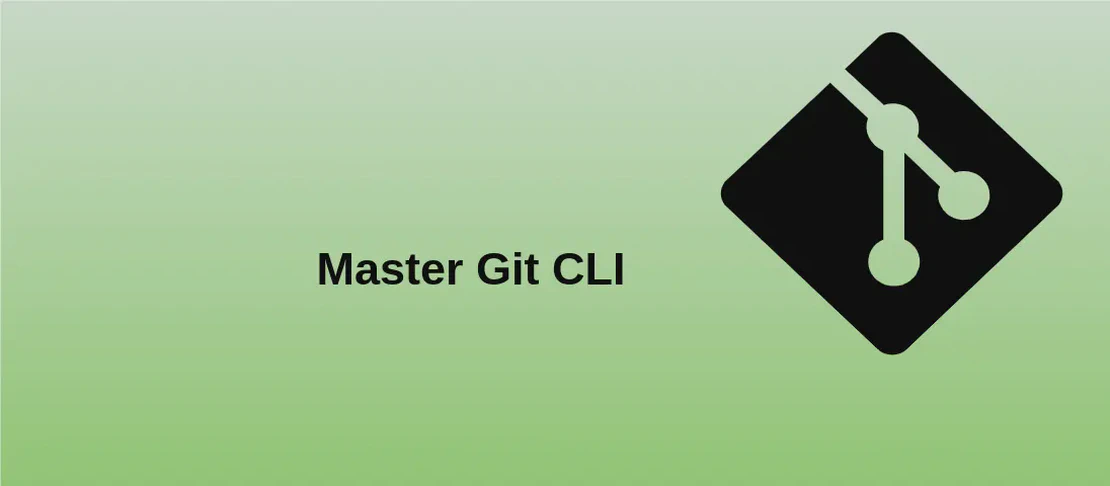
How to Use the Command 'git merge' (with Examples)
The git merge
command is one of the fundamental operations in the Git version control system. It allows users to integrate changes from different branches, facilitating the development process by combining the work from multiple team members or feature branches. Merging can be straightforward or complex, depending on the project’s scope and team’s workflow. This article explores various use cases for the git merge
command, highlighting its practical applications and how it streamlines collaborative efforts in software development.
Use Case 1: Merge a Branch into Your Current Branch
Code:
git merge branch_name
Motivation:
Merging a branch into the current branch is a frequent task in collaborative environments. When different team members work on separate features or fixes, they may work on separate branches. Once their work is complete and reviewed, the changes need to be merged into a main branch like main
or develop
. This command ensures that the current branch includes all updates and changes introduced in the specified branch. It helps synchronize the workspace across all contributors.
Explanation:
git
: Indicates that the command is utilizing the Git version control system.merge
: This subcommand specifies the action to integrate changes from a different branch.branch_name
: Represents the name of the branch whose changes you want to incorporate into your current active branch.
Example Output:
Updating 1a2b3c4..5d6e7f8
Fast-forward
file1.txt | 5 +++++
file2.txt | 3 ---
2 files changed, 5 insertions(+), 3 deletions(-)
Use Case 2: Edit the Merge Message
Code:
git merge --edit branch_name
Motivation:
When performing a merge, especially in larger teams or projects, clarity in commit history becomes pivotal. By editing the merge message, developers can provide context or details about the changes being integrated, ensuring clarity in the project’s history. This is particularly useful for documenting why certain decisions were made or noting any relevant discussions associated with the merge.
Explanation:
--edit
: This option opens an editor for you to write or modify the default merge commit message.branch_name
: Denotes the branch whose changes are being merged into your current branch.
Example Output:
Merge made by the 'recursive' strategy.
Please enter the commit message for your changes. Lines starting
with '#' will be ignored, and an empty message aborts the commit.
Use Case 3: Merge a Branch and Create a Merge Commit
Code:
git merge --no-ff branch_name
Motivation:
Creating explicit merge commits is beneficial when you want to preserve the historical context of feature branches. The --no-ff
(no fast-forward) option ensures that a merge commit is created even if the merge resolves as a fast-forward. This practice maintains a clear picture of project history, showing exactly when changes from one branch are combined with another, enhancing traceability.
Explanation:
--no-ff
: Prevents the merge from being fast-forwarded, thus creating a merge commit to record this integration explicitly.branch_name
: Name of the branch whose changes need to be incorporated.
Example Output:
Merge made by 'recursive'.
25 files changed, 120 insertions(+), 43 deletions(-)
Use Case 4: Abort a Merge in Case of Conflicts
Code:
git merge --abort
Motivation:
Merges may sometimes lead to conflicts, causing a state where manual intervention is required to resolve discrepancies between branch changes. In such scenarios, it’s crucial to restore the repository to its original state before the merge attempt. The --abort
option is a safety net, allowing developers to cancel the current merge process and maintain a clean repository state.
Explanation:
--abort
: This option reverts the current merge process, restoring the branch to the state before the merge operation was initiated.
Example Output:
Aborting: no changes.
You have unmerged paths.
Use Case 5: Merge Using a Specific Strategy
Code:
git merge --strategy strategy --strategy-option strategy_option branch_name
Motivation:
In some situations, the default merging strategy may not resolve the branch changes effectively. By specifying a particular strategy and accompanying options, developers gain control over how the merge proceeds, choosing methods better suited to their project’s needs. This flexibility is invaluable for complex repositories with intricate branching histories.
Explanation:
--strategy
: Allows you to specify the merging strategy, such as ‘recursive’, which is particularly useful for non-trivial merges.--strategy-option
: Provides extra parameters to modify the strategy’s behavior, giving more fine-grained control.branch_name
: The branch from which changes will be merged.
Example Output:
Auto-merging file3.txt
CONFLICT (content): Merge conflict in file3.txt
Automatic merge failed; fix conflicts and then commit the result.
Conclusion:
The Git merge
command is a versatile tool essential for integrating work across different branches in a collaborative project. Understanding these use cases and their applications enhances the efficiency and effectiveness of development workflows. By using the command strategically, developers ensure not only code integration but also clarity and maintainability of the project history.