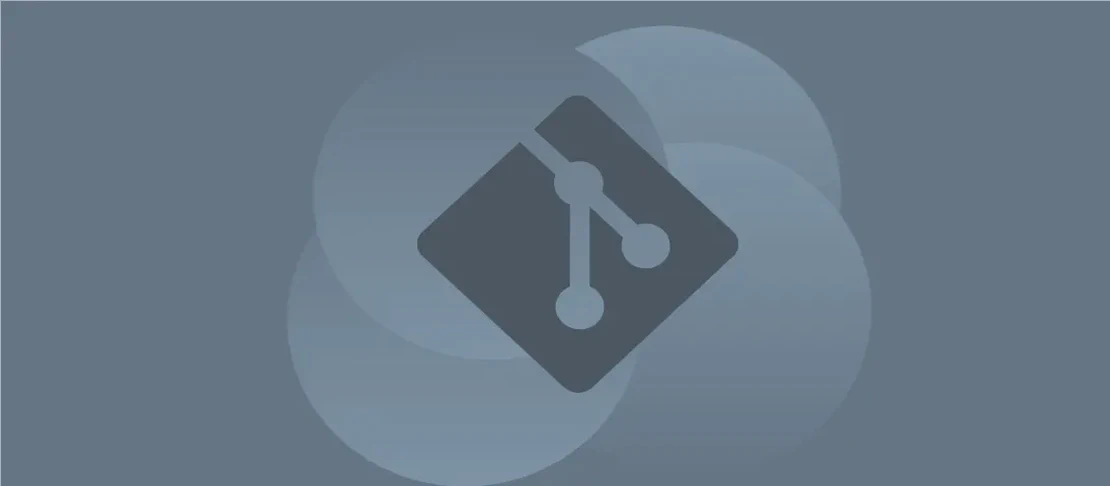
How to Use the Command 'git pull' (with Examples)
The git pull
command is an essential tool in the Git version control system that allows users to update their local branch with changes from a remote repository. It combines two basic functions: git fetch
, which downloads new data from a remote repository, and git merge
, which integrates those changes into your local branch. By streamlining these operations, git pull
keeps your local repository in sync with its remote counterpart, ensuring you have the most up-to-date version of the codebase.
Use Case 1: Download Changes from Default Remote Repository and Merge It
Code:
git pull
Motivation:
Often, developers work collaboratively on projects stored in remote repositories. Periodically updating your local repository with changes uploaded by teammates is crucial to maintaining the cohesion of your work with the ongoing project developments. The git pull
command without any additional arguments fetches changes from the default remote repository (usually called origin
) and automatically merges them into your current branch. This ensures that you are working with the latest version of the code, reducing code conflicts and integration issues.
Explanation:
git
: This is the command-line interface for Git, an open-source distributed version control system.pull
: This sub-command in Git automates the process of fetching updates from a remote repository and merging them with your local branch. Without specifying a remote or branch, Git assumes you want updates from the default remote (origin
) and branch you are currently on.
Example Output:
Updating f2a1c3d..4b90d2e
Fast-forward
file1.txt | 5 ++++-
file2.txt | 2 +-
2 files changed, 6 insertions(+), 1 deletion(-)
Use Case 2: Download Changes from Default Remote Repository and Use Fast-Forward
Code:
git pull --rebase
Motivation:
In some projects, maintaining a cleaner commit history is crucial. The --rebase
flag offers a way to integrate updates from a remote repository by reapplying your local changes on top of the incoming changes instead of creating a merge commit. This approach is particularly useful if you have made local commits and prefer a linear history without merge commits affecting the clarity of your commit logs.
Explanation:
--rebase
: This option alters the default merge behavior to reapply local changes on top of the changes from the fetch operation. It rebases your local changes to avoid creating a merge commit, keeping the commit history linear.
Example Output:
First, rewinding head to replay your work on top of it...
Applying: Updated design for feature module
Use Case 3: Download Changes from Given Remote Repository and Branch, Then Merge Them Into HEAD
Code:
git pull remote_name branch
Motivation:
In scenarios where multiple remotes or branches exist, it becomes essential to specify the exact remote repository and branch from which you wish to pull the updates. For instance, you might be working on a feature branch that requires integration of changes from another feature branch or a specific remote repository other than origin
. Specifying both the remote name and branch ensures precision in updating your local branch with the desired changes.
Explanation:
remote_name
: This refers to the specific remote repository name, such asorigin
,upstream
, or any custom name associated with the repository.branch
: This specifies the branch within the remote repository from which you want to pull updates. It is critical for ensuring you receive the correct set of changes.
Example Output:
From https://github.com/user/repo
* branch feature-xyz -> FETCH_HEAD
Updating 92c214b..03fcc8a
Fast-forward
feature_file.txt | 4 ++--
1 file changed, 2 insertions(+), 2 deletions(-)
Conclusion:
The git pull
command is a powerful and flexible tool in Git that streamlines the process of keeping your local repository in sync with remote changes. Whether you simply need to update your branch, want to maintain a clean commit history, or need to specify the exact source for updates, git pull
offers options to accommodate various workflow requirements. Mastery of this command is essential for any developer working in a collaborative environment using Git.