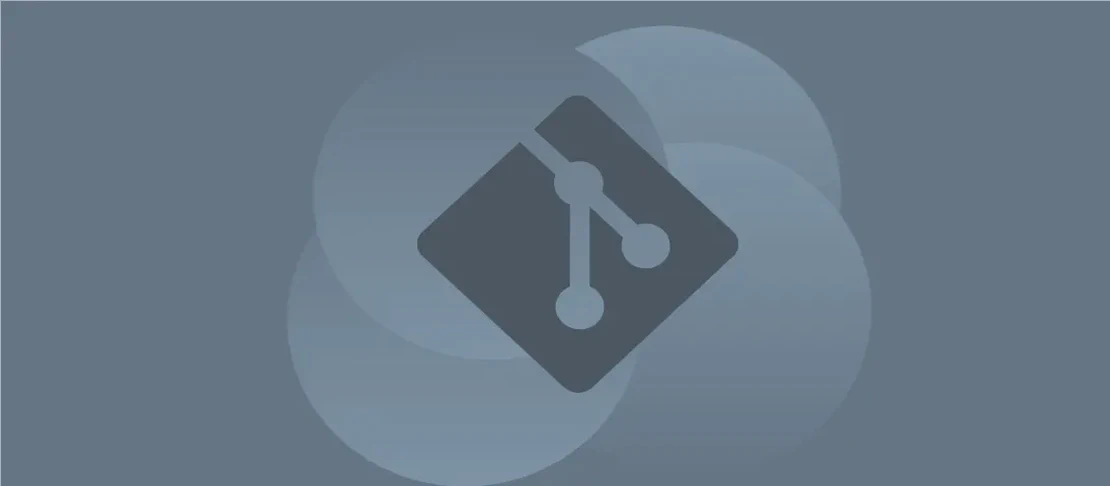
How to use the command 'git push' (with examples)
The git push
command in Git is used to upload local repository content to a remote repository. It transfers commits from your local repository to the remote repository’s equivalent branches. In a team environment, it facilitates collaborative development by allowing others to access the changes you’ve made.
Use case 1: Send local changes in the current branch to its default remote counterpart
Code:
git push
Motivation: This command is used when you want to update your default remote branch with the changes from your current local branch. It’s useful when you are working on a feature and wish to share your progress with others, or back it up to a remote server.
Explanation:
git
: This is the Git command-line tool you’re using.push
: This subcommand tells Git to transfer commits from your local repository to the remote repository.
Example Output:
Counting objects: 5, done.
Delta compression using up to 8 threads.
Compressing objects: 100% (3/3), done.
Writing objects: 100% (3/3), 345 bytes | 0 bytes/s, done.
Total 3 (delta 2), reused 0 (delta 0)
To https://github.com/username/repo.git
abc1234..def5678 main -> main
Use case 2: Send changes from a specific local branch to its remote counterpart
Code:
git push remote_name local_branch
Motivation: This use case is valuable when you have multiple branches and need to push changes from a specific branch, not necessarily the one you are currently on. It offers control over which branch’s changes get shared with the remote.
Explanation:
remote_name
: The name of the remote repository (likeorigin
) where you want to push the changes.local_branch
: The specific local branch you wish to update on the remote repository.
Example Output:
Counting objects: 10, done.
Delta compression using up to 8 threads.
Compressing objects: 100% (6/6), done.
Writing objects: 100% (6/6), 678 bytes | 0 bytes/s, done.
Total 6 (delta 4), reused 0 (delta 0)
To https://github.com/username/repo.git
123abc4..789def6 feature-branch -> feature-branch
Use case 3: Send changes from a specific local branch to its remote counterpart, and set the remote one as the default push/pull target of the local one
Code:
git push -u remote_name local_branch
Motivation:
This is particularly useful when you initially push a branch to a remote repository because the -u
option sets up tracking between the local and remote branches, making future git push
or git pull
commands simpler by omitting branch names.
Explanation:
-u
: Sets upstream tracking, linking the local branch with its remote counterpart for easier coordination.remote_name
andlocal_branch
: As previously explained, you specify the remote and local branches involved.
Example Output:
Branch 'feature-branch' set up to track remote branch 'feature-branch' from 'origin'.
Counting objects: 8, done.
Delta compression using up to 8 threads.
Compressing objects: 100% (5/5), done.
Writing objects: 100% (5/5), 789 bytes | 0 bytes/s, done.
Total 5 (delta 3), reused 0 (delta 0)
To https://github.com/username/repo.git
345def6..678abc9 feature-branch -> feature-branch
Use case 4: Send changes from a specific local branch to a specific remote branch
Code:
git push remote_name local_branch:remote_branch
Motivation: This operation is useful when you want to push changes from a local branch directly to a different remote branch. It gives you flexibility when the remote branch structure needs to differ from the local setup, perhaps for coordinating with other teams or parallel tracks of development.
Explanation:
local_branch:remote_branch
: This syntax allows specifying the source branch (local) and the destination branch (remote), bypassing the assumption that they are named the same.
Example Output:
Counting objects: 6, done.
Delta compression using up to 8 threads.
Compressing objects: 100% (4/4), done.
Writing objects: 100% (4/4), 567 bytes | 0 bytes/s, done.
Total 4 (delta 2), reused 0 (delta 0)
To https://github.com/username/repo.git
abc2345..def4567 temp-branch -> new-branch
Use case 5: Send changes on all local branches to their counterparts in a given remote repository
Code:
git push --all remote_name
Motivation: This command is beneficial when you need to synchronize all your local branches with the remote repository. It saves time by allowing a single command to handle the operation instead of pushing each branch individually.
Explanation:
--all
: This flag indicates that all local branches should be pushed to their remote counterparts.
Example Output:
Counting objects: 18, done.
Delta compression using up to 8 threads.
Compressing objects: 100% (10/10), done.
Writing objects: 100% (10/10), 890 bytes | 0 bytes/s, done.
Total 10 (delta 7), reused 0 (delta 0)
To https://github.com/username/repo.git
123abc4..456def7 main -> main
abc2345..def4567 feature1 -> feature1
def3456..ghi5678 feature2 -> feature2
Use case 6: Delete a branch in a remote repository
Code:
git push remote_name --delete remote_branch
Motivation: Utilize this command when you need to remove a branch from the remote repository after it has been merged or deemed unnecessary. This keeps the repository clean and prevents confusion.
Explanation:
--delete remote_branch
: This syntax specifies the desire to remove the named branch from the remote repository.
Example Output:
To https://github.com/username/repo.git
- [deleted] deprecated-branch
Use case 7: Remove remote branches that don’t have a local counterpart
Code:
git push --prune remote_name
Motivation: This operation cleans up remote branches that no longer have a local branch counterpart, which is useful after branch re-organization or cleanup to prevent unnecessary or obsolete branches on the remote.
Explanation:
--prune
: This option instructs Git to remove remote branches that don’t correspond to any local branches.
Example Output:
Pruning unused remote branches:
- [deleted] old-feature
Use case 8: Publish tags that aren’t yet in the remote repository
Code:
git push --tags
Motivation: Tags are often used for marking release points (e.g., version v1.0.0). This command helps in ensuring that all local tags, which might signify important points in the repository’s history, are also available in the remote repository.
Explanation:
--tags
: This flag indicates that all local tags should be pushed to the remote repository.
Example Output:
Counting objects: 3, done.
Writing objects: 100% (3/3), 356 bytes | 0 bytes/s, done.
Total 3 (delta 0), reused 0 (delta 0)
To https://github.com/username/repo.git
* [new tag] v1.0.0 -> v1.0.0
Conclusion:
The git push
command has many versatile options that are indispensable for working with remote repositories. Whether synchronizing changes, managing branches, or sharing important release tags, understanding these various use cases enhances efficiency in collaborative environments and keeps repositories well-organized. Each option complements different aspects of remote collaboration, ensuring that every team member is always on the same page.