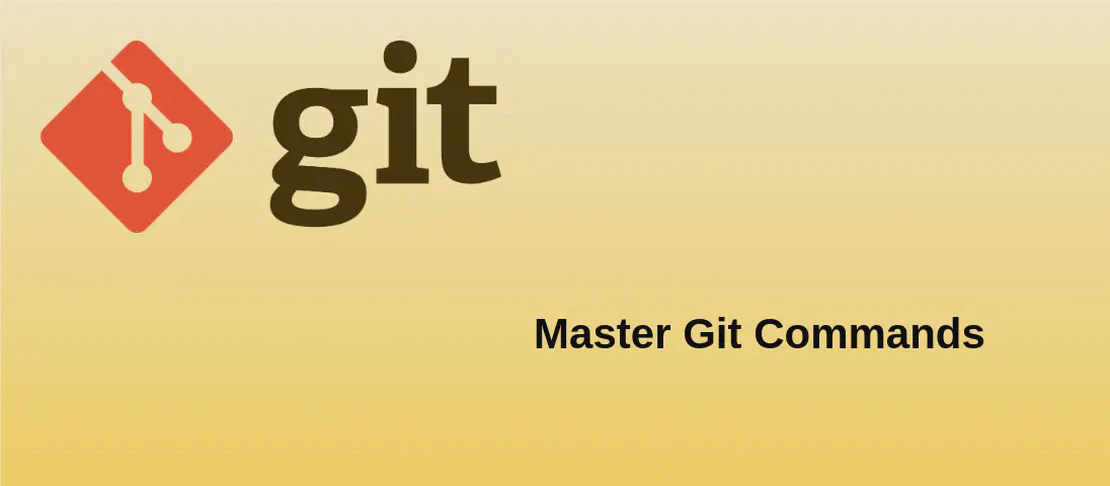
Mastering Git Rebase for Efficient Branch Management (with examples)
Git rebase is a powerful command used in version control with Git to efficiently manage and manipulate the commit history of a project. The primary function of git rebase is to reapply commits from one branch on top of another, effectively “moving” a branch to a new base. This can be particularly useful for maintaining a cleaner project history, integrating work in a tidy fashion, and resolving conflicts. Below are various use cases of the git rebase command, along with explanations and examples to illustrate its utility.
Rebase the current branch on top of another specified branch
Code:
git rebase new_base_branch
Motivation: When working in a collaborative environment, integrating the latest updates from a shared branch into your feature branch is often necessary. Rebasing allows you to incorporate these changes seamlessly, keeping the commit history linear and easier to understand. This is especially beneficial for maintaining a clean project history and simplifying merging efforts later.
Explanation:
git rebase
: The command initiates the rebase process.new_base_branch
: This argument specifies the branch onto which the current branch’s commits will be reapplied. It is the new base for your existing work.
Example Output:
First, rewinding head to replay your work on top of it...
Applying: Add new feature
Applying: Fix bug in new feature
Start an interactive rebase, which allows the commits to be reordered, omitted, combined or modified
Code:
git rebase -i target_base_branch_or_commit_hash
Motivation: Interactive rebasing offers developers the flexibility to refine the commit history of their project. By allowing modifications such as reordering, squashing, editing, or even removing specific commits, developers can craft a clear and concise history, making code reviews more straightforward and manageable.
Explanation:
git rebase -i
: The-i
flag stands for interactive, enabling a mode where the user can edit the commit history.target_base_branch_or_commit_hash
: The starting point (branch or commit hash) from where you want to begin manipulating commits.
Example Output:
pick a1b2c3d Add new feature
pick d4e5f6g Fix typo in feature
Continue a rebase that was interrupted by a merge failure, after editing conflicting files
Code:
git rebase --continue
Motivation:
During a rebase, merge conflicts are not uncommon. Once you have resolved these conflicts manually, it is crucial to proceed with the rebase process to integrate all intended changes. The --continue
command facilitates this progression, ensuring that your work is uninterrupted and properly applied.
Explanation:
git rebase --continue
: Continues the rebase process after resolving conflicts, effectively applying remaining unprocessed commits.
Example Output:
Applying: Add feature enhancement
Applying: Correct unit tests
Continue a rebase that was paused due to merge conflicts, by skipping the conflicted commit
Code:
git rebase --skip
Motivation: In certain situations, it might be more pragmatic to skip a specific commit during a rebase that causes a conflict, especially if that particular change is not essential or is being handled elsewhere. Skipping allows you to move past the problematic commit without halting the entire rebase process.
Explanation:
git rebase --skip
: Ignores the conflicted commit and proceeds with the remaining changes in the rebase sequence.
Example Output:
No changes - did you forget to use 'git add'?
Skipped patch 'Modify configuration' (a1b2c3d)
Applying: Add more tests
Abort a rebase in progress (e.g., if it is interrupted by a merge conflict)
Code:
git rebase --abort
Motivation: When a rebase process becomes too complex due to numerous conflicts or unexpected complications, aborting the operation might be the best option to revert to the original state. This approach allows developers to regroup, reassess their strategy, and preserve the stability of the existing codebase.
Explanation:
git rebase --abort
: Terminates the ongoing rebase process and returns to the state before the rebase initiated.
Example Output:
Git rebase aborted successfully. Working directory is clean.
Move part of the current branch onto a new base, providing the old base to start from
Code:
git rebase --onto new_base old_base
Motivation: In some scenarios, you might need to transplant a sequence of commits from your current work onto a different base, perhaps due to changes in project structure or alterations in upstream branches. This precise control over commit transcripts allows for strategic historical changes across feature or hotfix branches.
Explanation:
git rebase --onto new_base
: Designates the new starting point.old_base
: Specifies the original base up to which commits are to be moved.
Example Output:
Rebase in progress; onto d4f5e6g
Applying: Refactor authentication
Applying: Update login handler
Reapply the last 5 commits in-place, stopping to allow them to be reordered, omitted, combined or modified
Code:
git rebase -i HEAD~5
Motivation: Frequently, developers need to review recent work for efficiency improvements or eliminate redundant changes. Interactive rebasing of recent commits allows comprehensive opportunity for such adjustments without affecting older, stable commits.
Explanation:
git rebase -i
: The interactive flag for modifying commits.HEAD~5
: Targets the five most recent commits for alteration.
Example Output:
pick a1b2c3d Commit A
edit d4e5f6g Commit B
squash f7g8h9i Commit C
Auto-resolve any conflicts by favoring the working branch version (theirs
keyword has reversed meaning in this case)
Code:
git rebase -X theirs branch_name
Motivation:
Occasionally, certain merge conflicts may naturally favor the changes present in the incoming branch (theirs
). Using the -X theirs
strategy during rebasing automates conflict resolution in favor of these changes, streamlining integration efforts and reducing manual intervention.
Explanation:
git rebase -X theirs
: Automatically resolves conflicts preferring the changes frombranch_name
.branch_name
: The branch whose changes are prioritized in conflict resolution.
Example Output:
First, rewinding head to replay your work on top of it...
Auto-merging file.txt
CONFLICT (content): Merge conflict in file.txt
Automatic merge failed; fix conflicts and then commit the result.
Conclusion:
The git rebase command enhances version control by providing efficiency in managing branches, improving commit histories, and resolving conflicts. Each of its varied use cases—from simple rebasing to interactive modification of commits and conflict resolution—underscores its importance in a developer’s toolkit. With careful application, rebasing leads to cleaner, more understandable project histories, making it a preferred strategy in many collaborative environments.