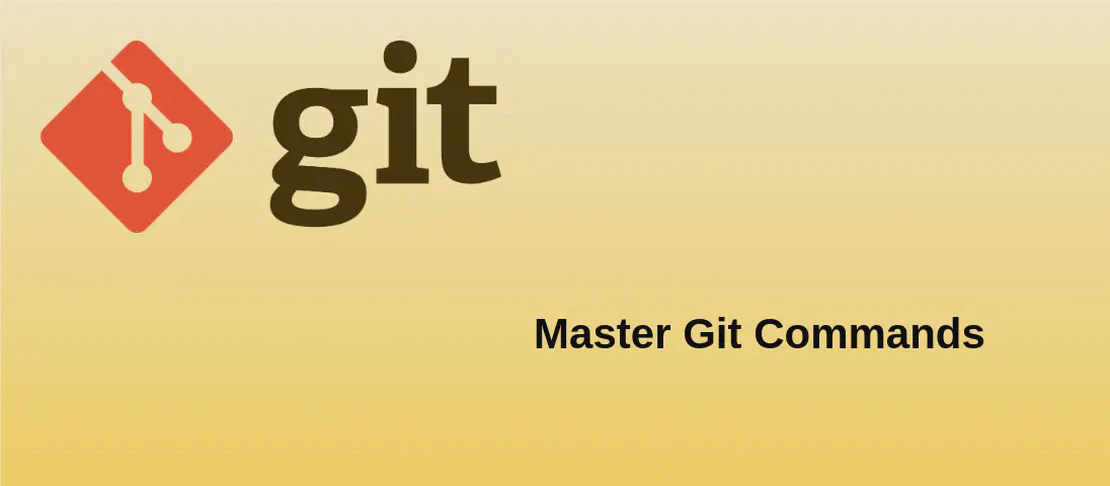
How to use the command 'git release' (with examples)
The git release
command is a convenient tool used for creating Git tags intended for releases. Part of the git-extras
toolkit, this command streamlines the process of tagging a specific commit in your git repository, which typically signifies the launch of a new version or milestone in your project. This functionality is fundamental for version control and release management, allowing developers to checkpoint their project’s progress at significant stages. The command simplifies what would otherwise be a more complex multi-step process into a single command line operation.
Use case 1: Create and push a release
Code:
git release tag_name
Motivation: In any development project, especially those following Agile methodologies or frequent public releases, there comes a moment when a certain state of the code is ready to be marked as a particular version. This is frequently done by tagging the commit, which allows developers and users to reference a specific point in the history that corresponds to a stable or significant state of the project. Using the git release tag_name
command provides a quick and simple way to create a lightweight tag and push it to the remote repository. This ensures that everyone working on the project, as well as automated deployment and CI/CD services, recognize it as a new release version.
Explanation: The git release
command followed by the tag_name
creates a new Git tag. A tag in Git is a reference to a specific commit and is commonly used to mark release points (e.g., v1.0, v2.1.2). When you run the command, it performs two main actions: it tags the commit checked out in your working directory with the provided tag_name
, and it pushes the new tag to the remote repository. This eliminates the manual steps of creating a tag and then pushing it separately.
Example output:
Tagged 'tag_name'.
Pushed tag 'tag_name' to origin.
The output confirms that the tag has been successfully created and pushed to the remote repository named ‘origin’.
Use case 2: Create and push a signed release
Code:
git release tag_name -s
Motivation: There are instances, especially in more security-conscious environments or open-source projects, where it is essential to ensure the authenticity and integrity of a release. For these cases, signing a release is crucial because it allows others to verify that the code has been authored by a trusted developer and has not been altered. The -s
flag in the git release
command is used to create a signed tag with GPG (GNU Privacy Guard), providing a cryptographic signature that associates the tag with the committer’s identity.
Explanation: The -s
option stands for ‘sign’, which instructs Git to sign the tag with your GPG key. This requires that you have already configured Git with a GPG key. When the command git release tag_name -s
is executed, Git will prompt you to provide a passphrase for your GPG key if one is set, and then it will proceed to create a signed tag. The signing process appends a digital signature to the tag data, enhancing its security and verifiability.
Example output:
You need a passphrase to unlock the secret key for
user: "Your Name <your.email@example.com>"
4096-bit RSA key, ID 12345678, created YYYY-MM-DD
Tagged 'tag_name'.
Pushed tag 'tag_name' to origin.
The message indicates that the tag was successfully signed and then pushed to the remote repository.
Use case 3: Create and push a release with a message
Code:
git release tag_name -m "message"
Motivation: Communicating the purpose or content of a release through a descriptive message is a best practice in version control. By associating a message with a Git tag, you can provide more context regarding what the specific commit or state represents, such as features added, bugs fixed, or other notable changes. Tagged messages serve as documentation that can be invaluable for team members or external users seeking to understand the history and progression of a project.
Explanation: The -m
option allows you to specify a tag message in the command line. This creates an annotated tag rather than a lightweight tag. Annotated tags include a descriptive message, the name of the person tagging, and the date. They are stored as full objects in the Git database. Executing git release tag_name -m "message"
attaches the given “message” to the tag, making it easily accessible whenever the tag history is reviewed.
Example output:
Created tag 'tag_name' with message: "message"
Pushed tag 'tag_name' to origin.
The output reflects the successful creation and push of the message-annotated tag, ensuring that the message is now part of the tag’s metadata.
Conclusion:
The git release
command from git-extras
offers a simplified approach to manage release tagging operations in Git. Whether you are marking a new version, ensuring authenticity with signed tags, or providing important contexts through tag messages, git release
streamlines the effort with efficient command line options. Integrating this command into your development workflow ensures better control and communication over the project’s version history and significant milestones.