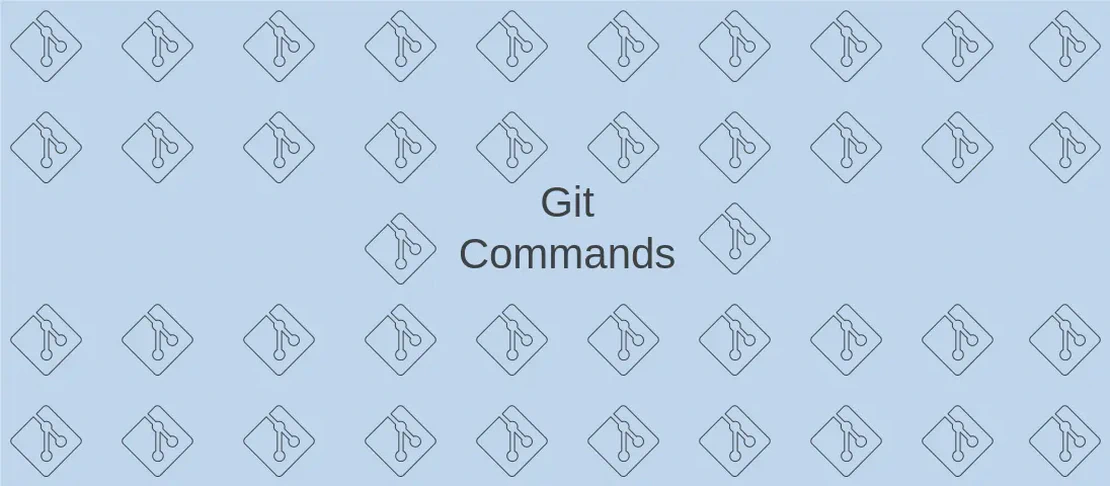
How to use the command 'git remote' (with examples)
The git remote
command is an integral part of Git version control, facilitating the management of connections to remote repositories. It allows developers to list, add, delete, and modify remote connections, effectively linking their local repositories with those hosted on platforms such as GitHub, GitLab, or Bitbucket. By managing these connections, developers can streamline collaboration and ensure seamless integration between local and remote codebases.
Use case 1: List existing remotes with their names and URLs
Code:
git remote -v
Motivation:
Understanding the remote repositories linked to your local repository is crucial for effective collaboration and code management. By listing existing remotes, you can verify the connections, especially when working on multiple projects or when you join a project with existing configurations.
Explanation:
git
: Invokes the Git command-line tool.remote
: Targets Git remote functionalities.-v
or--verbose
: Ensures all URLs for each remote are listed, providing comprehensive information about each connection for clarity and auditing purposes.
Example output:
origin https://github.com/your-username/your-repo.git (fetch)
origin https://github.com/your-username/your-repo.git (push)
upstream https://github.com/someone-else/another-repo.git (fetch)
upstream https://github.com/someone-else/another-repo.git (push)
Use case 2: Show information about a remote
Code:
git remote show origin
Motivation:
To maintain an effective workflow, it’s important to understand the specifics of interaction with a remote repository, such as branch tracking and head information. This command assists in diagnosing and planning next steps for a project’s remote setup.
Explanation:
git
: Initiates the Git tool.remote
: Engages with remote management tasks.show
: Requests detailed information about the specified remote.remote_name
(e.g.,origin
): Specifies which remote you want detailed insight into.
Example output:
* remote origin
Fetch URL: https://github.com/your-username/your-repo.git
Push URL: https://github.com/your-username/your-repo.git
HEAD branch: main
Remote branches:
develop tracked
main tracked
Local branches configured for 'git pull':
main merges with remote main
Local refs configured for 'git push':
develop pushes to develop (up to date)
main pushes to main (up to date)
Use case 3: Add a remote
Code:
git remote add origin https://github.com/your-username/your-new-repo.git
Motivation:
Adding a new remote repository is fundamental when setting up a new project or integrating a new remote branch source. It’s essential when setting up your project on a new machine or collaborating on an unfamiliar branch.
Explanation:
git
: Calls the Git executable.remote
: Manages remote repository interactions.add
: Instructs Git to create a new remote entry.remote_name
(e.g.,origin
): Assigns a name to this connection, allowing for easy reference.remote_url
: Specifies the full path (likely an HTTPS or SSH URL) to the remote repository you wish to track.
Example output:
Backend confirmation, no direct output: The remote is added successfully. You can verify it by running git remote -v
.
Use case 4: Change the URL of a remote
Code:
git remote set-url origin https://github.com/your-username/changed-repo.git
Motivation:
Changing the URL of a remote repository becomes necessary when a project’s repository location changes, such as moving to another platform or switching from HTTPS to SSH for better security.
Explanation:
git
: Activates Git operations.remote
: Deals with remote configurations.set-url
: Modifies the URL for the specified remote.remote_name
(e.g.,origin
): Identifies which remote’s URL will be updated.new_url
: Indicates the new URL of the remote repository.
Example output:
No direct output will be shown: Confirmation occurs upon listing remotes again (git remote -v
).
Use case 5: Show the URL of a remote
Code:
git remote get-url origin
Motivation:
Knowing the URL of a specific remote repository is crucial for ensuring that you’re fetching and pushing the changes to the correct destination. This is particularly critical in environments with similar project names or during migrations.
Explanation:
git
: Executes Git commands.remote
: Operates on aspects related to remotes.get-url
: Retrieves and displays the remote URL.remote_name
(e.g.,origin
): Specifies which remote’s URL will be returned.
Example output:
https://github.com/your-username/your-repo.git
Use case 6: Remove a remote
Code:
git remote remove origin
Motivation:
Removing a remote is necessary when a connection to a repository is no longer needed or when the repository is deprecated. This cleans up unnecessary configurations, reducing confusion and potential errors.
Explanation:
git
: Runs the Git command suite.remote
: Executes remote-related operations.remove
: Deletes the specified remote connection.remote_name
(e.g.,origin
): Indicates which remote to delete.
Example output:
No output reflects this executed action: The remote is removed successfully. Verification can be done through git remote -v
.
Use case 7: Rename a remote
Code:
git remote rename origin upstream
Motivation:
Renaming a remote allows developers to better organize and categorize their remotes as projects evolve. For instance, changing ‘origin’ to a more descriptive name like ‘upstream’ can enhance clarity, particularly when syncing changes from a forked repository.
Explanation:
git
: Begins the Git process.remote
: Focuses on managing remote connections.rename
: Changes the existing name of a remote.old_name
(e.g.,origin
): Refers to the current name of the remote.new_name
(e.g.,upstream
): The new name to be assigned to the remote for future references.
Example output:
No immediate output; action completion is silent: Confirm the change through git remote -v
.
Conclusion:
The git remote
command is pivotal for managing how your local repositories interact with remote repositories. Understanding and utilizing these use cases ensure seamless repository tracking, enhance collaborative efforts, and aid in maintaining an organized workflow. Whether you’re setting up, modifying, or tidying your repository connections, these examples underpin an adept use of git remote
.