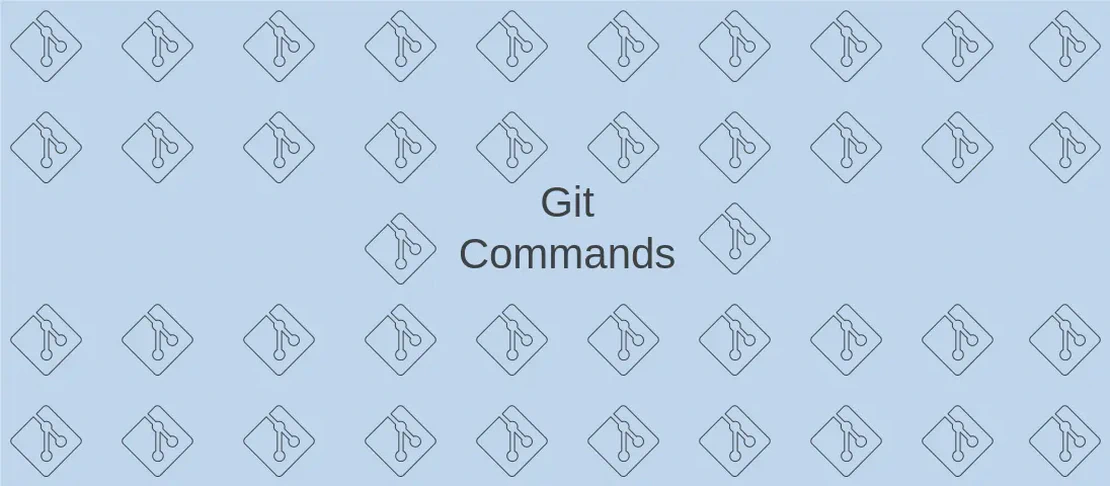
How to use the command 'git reset' (with examples)
The git reset
command is a powerful tool in Git that allows users to undo changes, be it unstaging files or even undoing previous commits. It essentially resets the current state of the Git repository HEAD to a specified point. The command can be utilized for minor tweaks, such as unstaging specific files, or for more substantial actions, like wiping out entire commit history up to a certain point. This flexibility makes git reset
an essential tool for developers managing version control in their projects.
Use case 1: Unstage everything
Code:
git reset
Motivation:
Imagine you’ve staged a multitude of files you thought were ready to be committed, but on second thought, you realize some need more work. Rather than unstaging each file individually, you can use git reset
to quickly unstage everything, allowing you to selectively stage the files again after making the necessary modifications.
Explanation:
git reset
: This command unstages all changes that are currently staged in your index. When no path or commit is specified, it automatically targets the whole staging area, making it clean without discarding any changes present in your working directory.
Example Output:
Upon executing the command, the staged changes within the index get moved back to the working directory, and running git status
afterwards displays no changes in the staging area but reflects the unstaged modifications.
Use case 2: Unstage specific file(s)
Code:
git reset path/to/file1 path/to/file2 ...
Motivation:
Sometimes while staging files for a commit, you might accidentally stage files not intended for the current commit. In such cases, it’s beneficial to unstage specific files without affecting others. This use allows you to refine your staging area with precision, focusing only on files relevant to the task at hand.
Explanation:
git reset
: The command is used to remove specific files from the staging area.path/to/file1 path/to/file2 ...
: These paths indicate the specific files you want to unstage. By specifying the paths, you’re instructing Git to keep the changes in those files while removing them from the index.
Example Output:
After executing the command, git status
will show the specified files as unstaged, listing them under “Changes not staged for commit,” while other files remain staged.
Use case 3: Interactively unstage portions of a file
Code:
git reset --patch path/to/file
Motivation:
Sometimes a single file may contain several changes, but only a portion of those are relevant for the next commit. Using git reset --patch
, you can unstage specific parts of a file, allowing more granular control and cleaner commit history that accurately reflects the logical changes.
Explanation:
git reset
: The base command used to alter the staging area’s content.--patch
: This flag enables an interactive mode, guiding you through each change in the specified file section by section, allowing you to precisely choose what to unstage.path/to/file
: Represents the file whose changes need to be selectively unstaged.
Example Output:
Executing the command provides an interactive session in which you are prompted to review each hunk of changes and decide whether to unstage it. The resulting ‘git status’ will only reflect the parts of the file as unstaged that you chose.
Use case 4: Undo the last commit, keeping its changes
Code:
git reset HEAD~
Motivation:
Suppose you’ve committed changes prematurely without including some vital modifications or you’ve realized the commit message was incorrect. You can use this particular use of git reset
to undo the last commit while keeping all its changes intact in your working directory, allowing you to amend or include additional work in a new commit.
Explanation:
git reset
: Initiates the reset process.HEAD~
: This reference denotes the last commit before the current HEAD. Resetting to HEAD~ essentially undoes the last commit while preserving its changes in the working directory.
Example Output:
After executing, the former commit disappears from the log, but the changes remain visible in your working directory as uncommitted. Running git status
will showcase these changes, ready to be staged again.
Use case 5: Undo the last two commits, adding their changes to the index
Code:
git reset --soft HEAD~2
Motivation:
Imagine a need arises where the past two commits need amendments or reforms, perhaps due to failing tests or project rebase requirements. The --soft
option facilitates this by adding their changes directly to the index, allowing quick adjustments and re-committing without manually adding them again.
Explanation:
git reset
: Commands the process of resetting.--soft
: This option ensures that the changes from the undone commits remain staged, so they’re ready to recombine into a new commit.HEAD~2
: Specifies the point two commits before HEAD to which you want to reset.
Example Output:
After running this command, the targeted commits disappear from the history. Nevertheless, all changes from those commits stay staged, which can be verified using git status
, ensuring they are prepared for an immediate new commit.
Use case 6: Discard any uncommitted changes
Code:
git reset --hard
Motivation:
In cases where your current work-in-progress becomes irrelevant or you need to revert a spaghetti code experiment without any intention of retaining changes, a hard reset quickly restores your working directory and index to their last commit state, removing all uncommitted changes entirely.
Explanation:
git reset
: Initiates the reset.--hard
: This flag ensures all changes in the working directory and index are discarded, restoring everything to the latest committed state.
Example Output:
Executing this command erases all uncommitted changes, resetting the workspace to mirror the latest commit. A git status
post-command indicates a clean working tree, reflecting no changes to be staged or committed.
Use case 7: Reset the repository to a specified commit
Code:
git reset --hard commit
Motivation:
You might discover a point in history where the project was in a stable state, and recent changes led to undesired outcomes. Using a hard reset to a specific commit lets you rollback entirely to that stable point in time, discarding all changes—committed and uncommitted—since then.
Explanation:
git reset
: Kick-starts the reset operation.--hard
: Dictates a comprehensive reset, clearing the working directory and index to reflect the specified commit.commit
: The hash or reference of the commit to which the repository should transition, discarding everything after.
Example Output:
Upon completion, your repository’s state exactly mirrors that specific commit, as shown by a git log
. Subsequent git status
confirms a clean slate, with no pending changes or stages.
Conclusion:
Mastering the git reset
command involves understanding its nuances across various situations, allowing developers to efficiently navigate and manage their repository states. With examples ranging from unstaging single files to completely resetting project timelines, users equipped with this command can handle both precise alterations and sweeping changes with confidence.