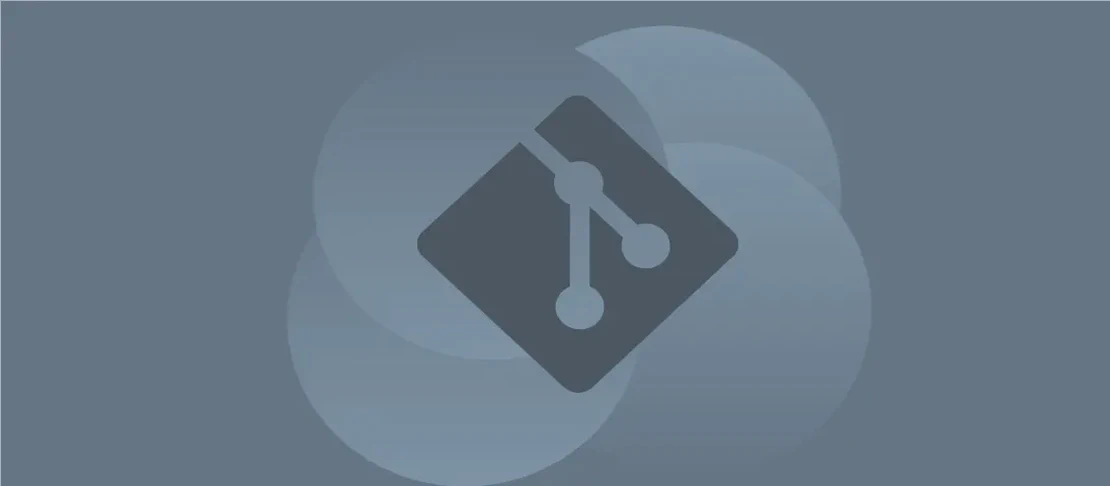
How to Use the Command 'git restore' (with examples)
The git restore
command is a powerful tool introduced in Git version 2.23, designed to restore files in your working directory and staging area to previous states. It serves as a more specialized alternative to older commands like git checkout
and git reset
, focusing on file restoration tasks. The command can be used to revert changes, unstage files, and even select parts of files to restore interactively.
Use case 1: Restore an Unstaged File to the Version of the Current Commit (HEAD)
Code:
git restore path/to/file
Motivation:
You might make several changes to a file and realize that you want to discard them, reverting the file back to the last committed state (i.e., the HEAD). This situation can arise when experimenting with different code strategies that ultimately do not work as intended.
Explanation:
git restore
: This command restores the file to a desired state.path/to/file
: Specifies the file path that you want to restore to its last committed version.
Example Output:
Restored 'path/to/file' to match the current commit.
Use case 2: Restore an Unstaged File to the Version of a Specific Commit
Code:
git restore --source commit path/to/file
Motivation:
If you’ve identified that a specific commit contains the favorable version of a file, use this command to restore that exact state. This is particularly useful when you need to backtrack to a known stable environment in a project.
Explanation:
--source commit
: Specifies the commit hash you want to restore the file from.path/to/file
: Indicates the file whose version you want restored from the specified commit.
Example Output:
Restored 'path/to/file' from commit [commit-hash].
Use case 3: Discard All Unstaged Changes to Tracked Files
Code:
git restore :/
Motivation:
During extensive changes across multiple files, some changes may prove unnecessary or incorrect, and you may want to discard all unstaged alterations for all tracked files. This brings your working directory back in line with the current commit without affecting staged changes.
Explanation:
:/
: A pathspec that targets all tracked files.
Example Output:
Discarded changes in tracked files.
Use case 4: Unstage a File
Code:
git restore --staged path/to/file
Motivation:
When a file is added to the staging area but you realize you need to make more changes or revert it completely, unstaging it can prevent errors from creeping into the commit.
Explanation:
--staged
: This option specifies that the file should be removed from the staging area.path/to/file
: Refers to the file you want to unstage.
Example Output:
Unstaged changes from 'path/to/file'.
Use case 5: Unstage All Files
Code:
git restore --staged :/
Motivation:
This is helpful when you’ve mistakenly staged many files and need to start over without committing incorrect or incomplete changes. Unstaging all files at once can save time and effort.
Explanation:
--staged
: Indicates the operation applies to files in the staging area.:/
: Targets all staged files.
Example Output:
Unstaged changes from all files.
Use case 6: Discard All Changes to Files, Both Staged and Unstaged
Code:
git restore --worktree --staged :/
Motivation:
At times, you’ve made a bunch of changes, staged some, unstaged others, and everything seems wrong. Instead of manually reverting each change, this command lets you clear out everything and restore all files to their last committed state efficiently.
Explanation:
--worktree
: Specifies files in the working directory.--staged
: Specifies files in the staging area.:/
: Applies to all files tracked by Git.
Example Output:
Discarded all changes in tracked and staged files.
Use case 7: Interactively Select Sections of Files to Restore
Code:
git restore --patch
Motivation:
This use case offers an interactive approach to reverting changes. When modifications are scattered across a file, and not all sections need to be restored, selecting specific hunks to restore gives greater control.
Explanation:
--patch
: Initiates an interactive mode where you can choose particular changes (hunks) to restore.
Example Output:
@@ -1,4 +1,4 @@
-Original line
+Modified line
Restore this hunk [y,n,q,a,d,/,e,?]?
Conclusion:
The git restore
command is versatile, offering precise control over which changes to discard and what files to unstage or reset. It’s an essential command for anyone dealing with frequent code changes, providing a structured approach to managing code evolution effectively. Whether you need to restore a single file, unstage changes, or remove all modifications, git restore
has the right tool for the job.