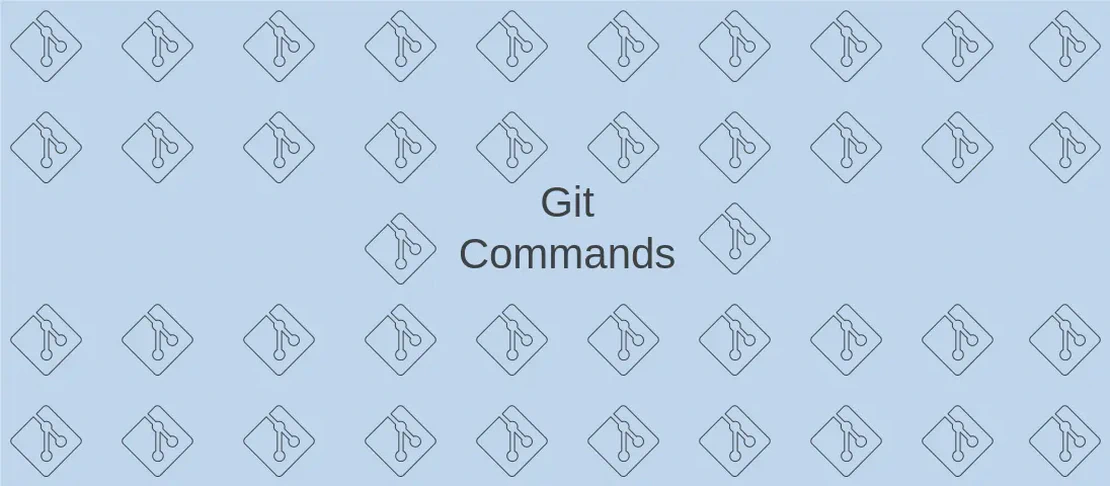
How to use the command 'git revert' (with examples)
Git is a powerful version control system that helps developers manage changes to their code over time. One essential command within Git is git revert
, which allows you to reverse the changes made by earlier commits in a controlled manner. Unlike git reset
, which erases commit history, git revert
creates new commits that effectively “undo” previous changes while preserving the commit history.
Revert the most recent commit
Code:
git revert HEAD
Motivation for using the example:
There are times when you commit a change and immediately realize it was a mistake—perhaps you merged a bug into the main codebase or accidentally included sensitive information. By using git revert HEAD
, you can quickly undo the last commit’s changes without disrupting the repository’s commit history, making it visible to others what change was reversed and why.
Explanation for every argument given in the command:
HEAD
: This shorthand reference points to the last commit on the current checked-out branch. RevertingHEAD
effectively negates the changes made by the most recent commit, creating a new commit that undoes those changes.
Example output:
[main abc123def] Revert "Incorrect feature implementation"
Date: Wed, 3 Nov 2023 10:01:00 -0400
1 file changed, 2 deletions(-), 2 insertions(+)
Revert the 5th last commit
Code:
git revert HEAD~4
Motivation for using the example:
Suppose you spot a problematic commit after some time, but it wasn’t the most recent one. The fifth last commit might have introduced a bug or instability that wasn’t immediately apparent. By targeting this specific commit with git revert HEAD~4
, you can fix the issue retrospectively without altering subsequent changes that were correct.
Explanation for every argument given in the command:
HEAD~4
: Represents the fifth commit from the current HEAD, moving four commits backward into your commit history. This parameter indicates which commit you are looking to reverse.
Example output:
[main cde456ghi] Revert "Feature X caused unexpected behavior"
Date: Thu, 4 Nov 2023 11:05:00 -0400
1 file changed, 3 deletions(-), 1 insertion(+)
Revert a specific commit
Code:
git revert 0c01a9
Motivation for using the example:
Often, developers need to target specific commits for reversion due to errors discovered at a later date, or because a commit was found to have conflicts with newer developments. By specifying a certain commit hash, such as 0c01a9
, git revert
allows precision reversal of undesirable changes, leaving other alterations intact.
Explanation for every argument given in the command:
0c01a9
: This is a short hash representation of a specific commit you want to revert. The hash uniquely identifies the commit and allows git to locate and undo the changes introduced by it.
Example output:
[main def789jkl] Revert "Introduce Feature Y prematurely"
Date: Fri, 5 Nov 2023 12:10:00 -0400
1 file changed, 4 deletions(-), 4 insertions(+)
Revert multiple commits
Code:
git revert branch_name~5..branch_name~2
Motivation for using the example:
In some cases, multiple consecutive commits need to be reverted collectively. Perhaps a series of commits implemented a feature that needs to be temporarily rolled back due to significant bugs. In such scenarios, the git revert branch_name~5..branch_name~2
command allows you to address multiple problematic commits in a range efficiently.
Explanation for every argument given in the command:
branch_name~5..branch_name~2
: This specifies a range of commits to be reverted, starting from the fifth last commit (branch_name~5
) and ending just before the second last commit (branch_name~2
) on the specified branch. This syntax efficiently selects multiple commits for reversal.
Example output:
[main ghi012mno] Revert "Comprehensive feature rollout - Part 1"
Date: Sat, 6 Nov 2023 13:15:00 -0400
1 file changed, 6 deletions(-), 6 insertions(+)
[main jkl345pqr] Revert "Comprehensive feature rollout - Part 2"
Date: Sat, 6 Nov 2023 13:15:02 -0400
1 file changed, 3 deletions(-), 2 insertions(+)
Don’t create new commits, just change the working tree
Code:
git revert -n 0c01a9..9a1743
Motivation for using the example:
There might be scenarios where you want to reverse the effects of specific commits but don’t want to commit these reversals immediately—for example, you may want to revert and then combine the changes with additional edits before committing. The --no-commit
(or -n
) option provides a flexible workflow to make multiple changes at once.
Explanation for every argument given in the command:
-n|--no-commit
: This flag applies the revert changes to the working tree and index without committing them, allowing further modifications to be staged or discarded before creating a new commit.0c01a9..9a1743
: This indicates a range of commit hashes from0c01a9
to9a1743
, targeting all included commits for reversion in your working directory.
Example output:
Changes to be committed:
(use "git reset HEAD <file>..." to unstage)
modified: file1.js
modified: file2.js
Conclusion
The git revert
command is an essential tool in any developer’s version control toolkit, providing a way to undo changes while preserving the project’s full commit history. Through various examples, you can see how git revert
can adapt to different scenarios—whether immediately reversing the last commit or carefully handling a range of problematic changes. Understanding these use cases not only ensures a safer codebase but also maintains clear, comprehensible documentation of the changes made over time.