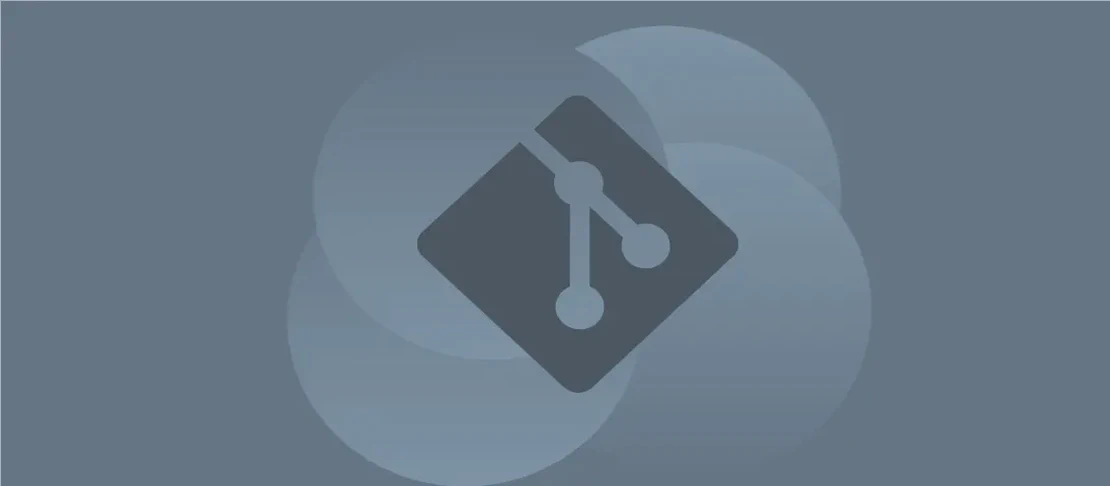
How to Use the Command 'git rm' (with Examples)
The git rm
command is a powerful tool in Git that allows users to remove files or directories from both the repository index and the local filesystem. It can also be used to simply remove files from the index, keeping the local versions intact. This article explores the different ways you can utilize git rm
through practical examples.
Use case 1: Remove File from Repository Index and Filesystem
Code:
git rm path/to/file
Motivation:
Imagine you have several obsolete files cluttering your project, which not only need to be removed from the version control system but also from your local working directory. This command performs a dual function: cleaning up the repository and freeing up space on your local machine. It helps maintain an organized codebase, ensuring that only necessary files are tracked and stored.
Explanation:
git rm
: This command initiates the removal process for file entries from the index and the filesystem.path/to/file
: This specifies the exact directory and name of the file you wish to remove. You replace this placeholder with your actual file path.
Example Output:
When you run the git rm path/to/file
command, a message appears in your terminal confirming that the file has been deleted from your project directory and added to the staging area for the next commit:
rm 'path/to/file'
Use case 2: Remove Directory
Code:
git rm -r path/to/directory
Motivation:
Sometimes, entire directories become redundant in a project due to refactoring or changes in project requirements. In such situations, removing a directory and its contents one file at a time can be cumbersome. The git rm -r
command is designed to handle this efficiently by recursively removing all files within the specified directory, streamlining your workflow and ensuring no residual files are left untracked.
Explanation:
git rm
: Initiates the command for removing items from the index and the local filesystem.-r
: Stands for recursive, meaning the command will remove the directory and all its contents.path/to/directory
: The path specifies the directory that needs to be removed.
Example Output:
After executing git rm -r path/to/directory
, the terminal should list each file and subdirectory removed, helping you verify the cleanup operation:
rm 'path/to/directory/file1'
rm 'path/to/directory/file2'
...
Use case 3: Remove File from Repository Index but Keep It Untouched Locally
Code:
git rm --cached path/to/file
Motivation:
At times, you may want to keep a file in your project locally but ensure it is no longer tracked by Git - perhaps a configuration file that is machine-specific and shouldn’t be part of the repository. The git rm --cached
command is valuable in such scenarios. It allows you to exclude a file from version control while preserving the file on your local disk, preventing any data loss or disruption in your workflow.
Explanation:
git rm
: Used to remove items from the index.--cached
: The flag signifies that the file should be removed from the repository’s index but not from your local filesystem.path/to/file
: Specifies the filepath of the file to be removed from version control but kept intact locally.
Example Output:
On running git rm --cached path/to/file
, you’ll receive confirmation that the file is removed from the index but remains on your local machine:
rm 'path/to/file'
Conclusion:
The git rm
command is integral to efficient Git workflow management. It assists in maintaining a clean repository by removing unnecessary files and directories, along with offering options to keep local copies of files that must not be tracked. Understanding and utilizing these different use cases ensures robust collaboration and streamlined project maintenance in Git-based environments.