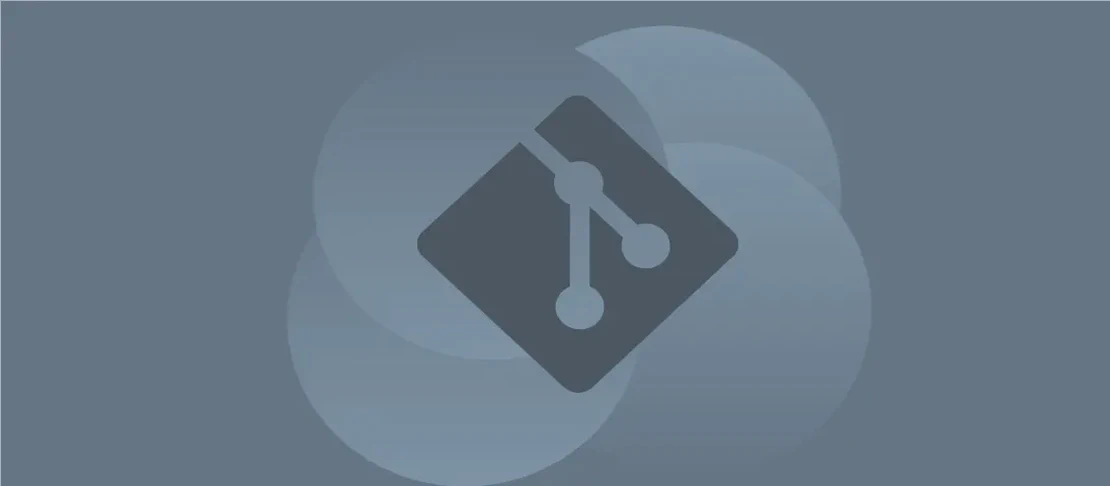
How to Securely Manage Sensitive Files with 'git secret' (with examples)
‘git secret’ is a tool designed to safely store private data within a Git repository by making use of encryption. Written in Bash, it allows teams to manage sensitive information like passwords or API keys securely while maintaining the benefits of version control. By encapsulating encryption and decryption processes, ‘git secret’ ensures that only authorized users can access specific files, providing an effective method for collaboration on projects with confidential data.
Initialize git-secret
in a local repository (with examples)
Code:
git secret init
Motivation:
The first step in using ‘git secret’ is initialization. By setting up ‘git secret’ in a repository, you prepare the environment to store encrypted files. This process sets up the necessary configuration for ‘git secret’ to operate, creating essential directories and files that will manage secret files. It’s crucial for any project that will handle sensitive data securely.
Explanation:git secret init
takes no additional arguments, as it simply initializes the necessary files and directories in the current repository for using ‘git secret’. It creates a hidden folder named ‘.gitsecret’ which contains essential setup files.
Example Output:
git-secret: init created: .gitsecret/keys
git-secret: done.
Grant access to the current Git user’s email (with examples)
Code:
git secret tell -m
Motivation:
This command is useful for quickly configuring access for the current user who has committed to the repository. It simplifies the process of adding a user to the list of authorized users by utilizing the email associated with their Git configuration, ensuring they can encrypt and decrypt secret files without manually entering their email.
Explanation:git secret tell -m
automatically detects the email from the user’s local Git configuration and adds it to the access list, allowing the user to encrypt and decrypt secret files. ‘-m’ is a shorthand for using the user’s email from the local Git settings.
Example Output:
git-secret: adding user@example.com to .gitsecret/keys/pubring.kbx
git-secret: done.
Grant access by email (with examples)
Code:
git secret tell email
Motivation:
There may be scenarios where you need to grant access to a specific user whose email is not part of your local Git configuration. This command accommodates such situations by allowing you to specify the email address of the user who requires access to the encrypted content.
Explanation:git secret tell email
requires a single argument, ’email’, representing the email address of the user you want to provide access to. This action adds the user’s GPG key to the repository, granting them permission to utilize ‘git secret’ commands to encrypt and decrypt files.
Example Output:
git-secret: adding another-user@example.com to .gitsecret/keys/pubring.kbx
git-secret: done.
Revoke access by email (with examples)
Code:
git secret killperson email
Motivation:
There are times when you need to revoke access from a user who no longer requires or is authorized to view the secret files. This could be due to changes in project roles or security protocols. Revoking access allows you to maintain control over sensitive information and ensures that only current authorized users have access.
Explanation:git secret killperson email
takes one argument, ’email’, specifying the email address of the user whose access you want to revoke. This command removes the specified user’s key from the repository’s keyring, preventing them from decrypting any of the secret files.
Example Output:
git-secret: removed key for user@example.com from .gitsecret/keys/pubring.kbx
git-secret: done.
List emails with access to secrets (with examples)
Code:
git secret whoknows
Motivation:
Being able to audit or review the list of users who currently have access to your secret files is essential for ensuring that your security and access protocols are being properly followed. This command gives you a snapshot of who is authorized to decrypt files, helping maintain a clear understanding of your project’s security posture.
Explanation:git secret whoknows
does not require additional arguments. It queries the current list of users whose GPG keys are stored in the .gitsecret/keys/pubring.kbx
file, showing who can access the encrypted files in the repository.
Example Output:
user@example.com
another-user@example.com
Register a secret file (with examples)
Code:
git secret add path/to/file
Motivation:
Registering a secret file allows you to track which files will be encrypted in the repository. This step is necessary for designating files as sensitive and ensuring they are not exposed in plaintext. It’s a vital process in securing sensitive data within your project’s repository.
Explanation:git secret add path/to/file
requires one argument, ‘path/to/file’, which is the location of the file you want to register as a secret. This action tells ‘git secret’ to include the specified file in encryption operations, marking it for protection.
Example Output:
git-secret: adding path/to/file
git-secret: done.
Encrypt secrets (with examples)
Code:
git secret hide
Motivation:
Encrypting your secret files is the core function of ‘git secret’. This step is necessary whenever you make changes to the registered secret files to ensure they are stored securely. Encrypting the files transforms them into an unreadable format unless decrypted with the correct access, thereby protecting sensitive information from unauthorized viewing.
Explanation:git secret hide
functions without additional arguments. It encrypts all files that have been registered with ‘git secret add’, creating an encrypted version of each file with a .secret
extension. This step ensures that the sensitive information is only accessible by users granted decrypt privileges.
Example Output:
git-secret: encrypting path/to/file
git-secret: done. 'path/to/file.secret' created.
Decrypt secret files (with examples)
Code:
git secret reveal
Motivation:
At certain points, authorized users need to access the raw data in the secret files. ‘git secret reveal’ decrypts these files, making them readable for users granted the decryption privilege. Ensuring the correct people can accurately access necessary information is key for productivity and secure collaboration.
Explanation:git secret reveal
requires no additional arguments and decrypts all the files that were encrypted using ‘git secret hide’. During this process, it utilizes the user’s GPG key stored in the repository to unlock the files for viewing or editing.
Example Output:
git-secret: revealing path/to/file.secret
git-secret: done. 'path/to/file' decrypted.
Conclusion:
Using ‘git secret’ to manage encrypted files in a Git repository offers a robust solution to maintaining data confidentiality. Each of these use cases highlights essential features of ‘git secret’ for initializing, managing access, handling secret files, and performing critical encryption and decryption operations. By following these steps, users can ensure sensitive information is stored safely, accessible only by authorized team members, keeping collaboration secure across the repository.