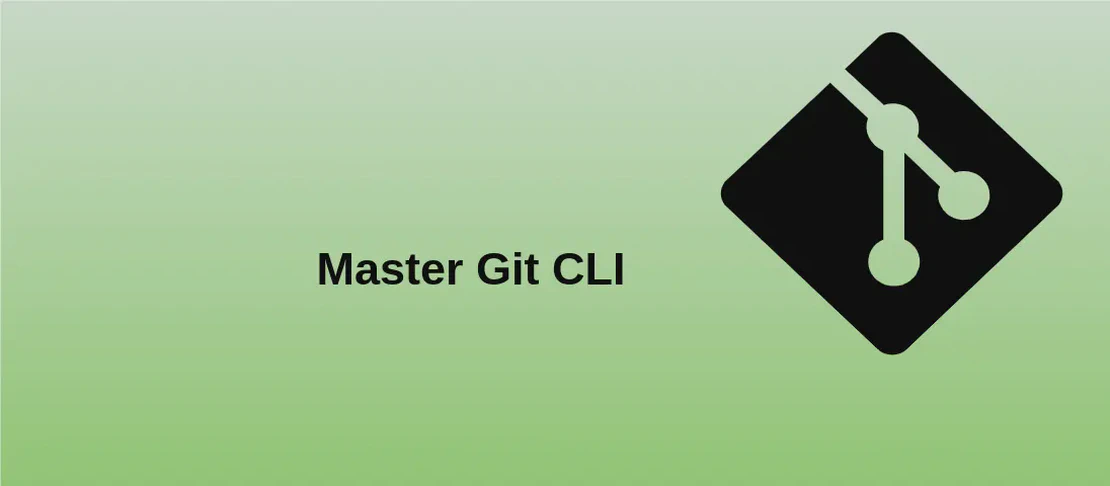
How to Use the Command 'git sed' (with examples)
The git sed
command is a powerful tool designed for developers working with Git-controlled repositories. It allows users to perform search-and-replace operations using sed
, a stream editor for filtering and transforming text. This command is a part of the git-extras
package, which enhances Git by introducing additional functionalities. Whether it’s about making quick text replacements throughout an entire repository or performing more complex operations using regular expressions, git sed
streamlines the process and integrates seamlessly with Git’s version control system.
Use case 1: Replace the specified text in the current repository
Code:
git sed 'find_text' 'replace_text'
Motivation:
In the world of software development, it’s common to encounter situations where a term or variable name needs to be updated across an entire codebase. This use case is particularly beneficial for developers who need to standardize naming conventions or rectify a typo throughout multiple files in a repository. Using git sed
, this process becomes automated, reducing manual effort and the risk of human error.
Explanation:
'find_text'
: This is the text string you are searching for within the repository. You need to replace this specific string.'replace_text'
: This is the text string that will replace all occurrences offind_text
across the repository.
Example Output:
If you had a variable named oldVar
in several files and you wanted to change it to newVar
, after running the command, all instances of oldVar
in the repository would be replaced with newVar
.
Use case 2: Replace the specified text and then commit the resulting changes with a standard commit message
Code:
git sed -c 'find_text' 'replace_text'
Motivation:
Automation is key in DevOps practices and continuous integration pipelines. When a modification is made, such as updating a library name, it often needs to be committed immediately. This command not only replaces the specified text but also commits the change, ensuring your repository is up-to-date with minimal manual intervention.
Explanation:
-c
: The-c
flag enables automatic committing of changes after the text replacement, utilizing a default commit message.'find_text'
: Represents the text targeted for replacement.'replace_text'
: The new text that will take the place offind_text
.
Example Output:
Upon execution, the repository will not only have all occurrences of find_text
replaced with replace_text
, but a commit will also be created capturing these changes with a message like “Replaced text in repository”.
Use case 3: Replace the specified text using regular expressions
Code:
git sed -f g 'find_text' 'replace_text'
Motivation:
Text patterns don’t always appear as simple strings, especially in complex codebases where developers may use syntaxes like dates, file paths, or email formats. This use case is designed for situations requiring regular expressions, allowing developers to employ advanced pattern matching techniques to identify and replace text effectively.
Explanation:
-f g
: This option informsgit sed
to interpret thefind_text
as a regular expression, providing greater flexibility in identifying patterns for replacement.'find_text'
: A regex pattern describing the text to search for.'replace_text'
: The replacement text for any matching pattern.
Example Output:
Someone wanting to replace all comments in the code that start with # TODO
with # FIXME
would see these changes applied across any pattern matching # TODO
in the repository.
Use case 4: Replace a specific text in all files under a given directory
Code:
git sed 'find_text' 'replace_text' -- path/to/directory
Motivation:
When working in a large project, changes might need to be isolated to a particular section of the codebase, such as a new feature module or a helper directory. This targeted approach allows developers to confidently make updates without touching other parts of the repository, thus maintaining stability in unaffected areas.
Explanation:
'find_text'
: The text to find within the given directory.'replace_text'
: The replacement string for thefind_text
instances found.--
: This delimiter separates the text replacement parameters from the directory path to ensure the command correctly interprets the target.path/to/directory
: Specifies the directory path within which the text replacement should be executed.
Example Output:
If changing errorHandler
to exceptionHandler
just in the src/components
directory, the command applies the changes only within that directory, leaving other parts of the repository untouched.
Conclusion:
The git sed
command is a versatile addition to a developer’s toolkit for performing text replacement tasks within Git repositories. By understanding its various use cases—simple text replacement, automating commits, using regular expressions, and focusing on specific directories—developers can greatly enhance their efficiency in managing codebases, minimizing manual workload, and maintaining consistency across their projects.