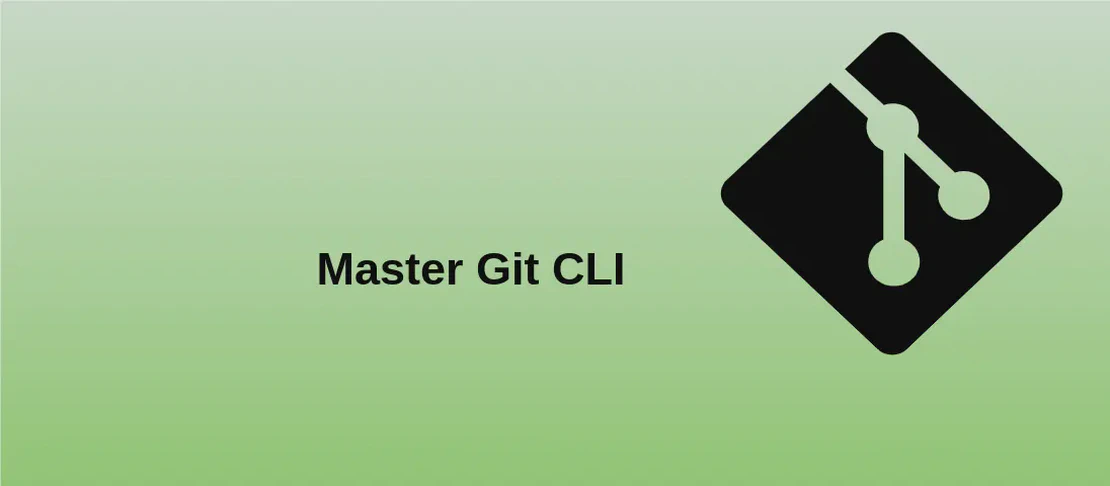
Mastering the `git show-branch` Command (with examples)
Git is a decentralized version control system that helps developers track changes, collaborate with others, and manage different versions of software projects. The git show-branch
command is a versatile tool that aids developers in examining branch and commit information within a Git repository. This command can be used to compare branches, review commit histories, and provide detailed information on remote and local branches, among other functionalities. Below, we explore various use cases of git show-branch
, demonstrating how it can streamline your development workflow.
Use case 1: Show a summary of the latest commit on a branch
Code:
git show-branch branch_name
Motivation: Sometimes, you need a quick overview of what the latest changes on a particular branch are, without diving deep into the entire commit history. This is particularly useful when working with multiple branches and seeking to verify the most recent changes.
Explanation: The branch_name
argument specifies the branch for which you want to retrieve the latest commit summary. This command provides an easy way to see recent modifications and verify that changes are correctly applied to the branch.
Example Output:
[branch_name] Commit message description
Use case 2: Compare commits in the history of multiple commits or branches
Code:
git show-branch branch_name1 branch_name2
Motivation: To reconcile differences between multiple branches, viewing their respective commits side by side is beneficial. This is essential during merges or when reviewing changes before integration.
Explanation: Here, branch_name1
and branch_name2
are the branches you wish to compare. This command juxtaposes the commit histories and highlights what differs across the specified branches.
Example Output:
! [branch_name1] Commit message of branch_name1
* [branch_name2] Commit message of branch_name2
-- [branch] Start point of divergence
Use case 3: Compare all remote tracking branches
Code:
git show-branch --remotes
Motivation: Modern development often involves collaboration over remote repositories. Being able to inspect all remote branches allows you to keep track of their latest state and identify changes others have made.
Explanation: The --remotes
flag instructs Git to display the commit history of all branches that track remotes. This broad comparison assists in understanding other contributors’ updates.
Example Output:
! [origin/branch1] Commit message of remote branch1
* [origin/branch2] Commit message of remote branch2
Use case 4: Compare both local and remote tracking branches
Code:
git show-branch --all
Motivation: In complex projects, it is necessary to evaluate changes both locally and remotely to ensure synchronization and identify any discrepancies across development lines.
Explanation: The --all
flag is a comprehensive command that compares every branch, compiling both local and remote insights into one view. This is crucial for a thorough status check of a project’s health.
Example Output:
! [branch1] Commit message of local branch1
* [origin/branch1] Commit message of remote branch1
Use case 5: List the latest commits in all branches
Code:
git show-branch --all --list
Motivation: Quickly listing recent commits from all branches provides a broad perspective on project progression and identifies the most recent updates across all development lines.
Explanation: The combined --all
and --list
flags instruct Git to enumerate the most recent commits from all branches, yielding a complete status overview of the repository.
Example Output:
[branch1] Commit message on branch1
[branch2] Commit message on branch2
Use case 6: Compare a given branch with the current branch
Code:
git show-branch --current branch_name
Motivation: When actively developing on one branch, it is often useful to understand how it compares to another branch, which might contain changes intended for merge or analysis.
Explanation: By specifying --current
, Git compares the branch designated by branch_name
with the branch you’re currently working on, facilitating preparation for a potential merge or analysis.
Example Output:
[branch_name] Commit message of given branch
[current] Commit message of current branch
Use case 7: Display the commit name instead of the relative name
Code:
git show-branch --sha1-name --current branch_name
Motivation: In scenarios where precise identification of commits is necessary (e.g., reviewing logs or debugging), displaying SHA-1 commit hashes provides definitive and recognizable identifiers.
Explanation: The --sha1-name
flag configures Git to display the complete SHA-1 hash for clearer reference. Combined with --current
and a specific branch_name
, it reveals exact commit identifiers for scrutiny.
Example Output:
SHA-1 hash | Commit message
Use case 8: Keep going a given number of commits past the common ancestor
Code:
git show-branch --more 5 branch_name1 branch_name2
Motivation: Understanding the divergence point and subsequent developments in branch histories is often necessary for debugging or detailed version reviews, particularly after merges.
Explanation: The --more 5
flag specifies that Git extends its evaluation to include five additional commits past the common ancestor of branch_name1
and branch_name2
. Testing further into the history clarifies how changes evolved after initially splitting.
Example Output:
[branch_name1] Commit message after divergence
[branch_name2] Divergent commit message
Conclusion:
By leveraging the git show-branch
command with its various flags and options, developers can gain a comprehensive understanding of their repository’s branches and commits. Whether comparing histories, preparing for merges, or conducting code reviews, these use cases demonstrate how git show-branch
is an invaluable command that enhances Git’s powerful suite of tools.