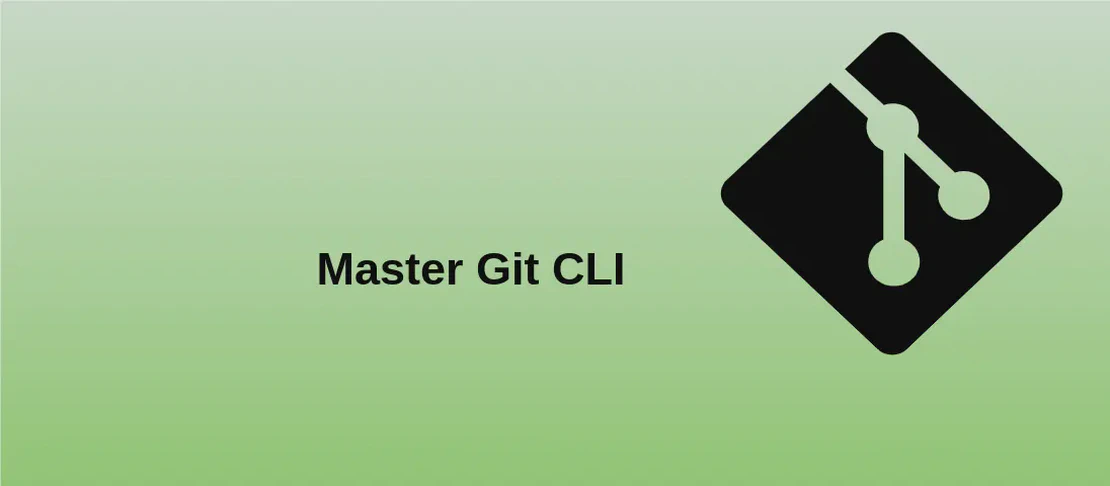
Mastering the Git Stash Command (with examples)
The git stash
command is a powerful tool in Git that allows developers to temporarily save modifications without committing them to the repository. It provides a way to clean your working directory without losing your changes, facilitating a seamless workflow when switching between branches or pulling in new changes. This article illustrates various use cases of the git stash
command with practical examples.
Stash Current Changes with a Message, Except New (Untracked) Files
Code:
git stash push --message optional_stash_message
Motivation:
Imagine you’re halfway through a new feature and ready to start a new hotfix on the same branch. You want to temporarily set aside your unfinished work but still have a meaningful message to recall what was stashed.
Explanation:
git stash push
: Initiates the stashing process, saving your changes.--message optional_stash_message
: Provides a human-readable message to the stash entry, making it easier to retrieve later. Theoptional_stash_message
is a placeholder for your custom message.
Example Output:
Saved working directory and index state WIP on main: abc1234Optional stash message
Stash Current Changes, Including New (Untracked) Files
Code:
git stash --include-untracked
Motivation:
During development, you may create new files that aren’t yet tracked. When switching contexts to another task, you want to stash changes, including those new files, to prevent losing unsaved work.
Explanation:
git stash
: Initiates the stashing process.--include-untracked
: Ensures that untracked files are included in the stash, safeguarding all modifications and additions.
Example Output:
Saved working directory and index state WIP on main: abc1234 Changes with untracked files
Interactively Select Parts of Changed Files for Stashing
Code:
git stash --patch
Motivation:
Suppose you’ve made extensive changes across multiple files and only want to stash specific parts of those modifications, while continuing to work on other parts.
Explanation:
git stash
: Initiates the stashing process.--patch
: Launches an interactive mode, allowing you to choose precise chunks of code from modified files to stash.
Example Output:
Stashing changes in 'file.txt'
Stage this hunk [y,n,q,a,d,e,?]? y
...
Saved working directory and index state WIP on main: abc1234
List All Stashes
Code:
git stash list
Motivation:
Over time, you may end up with multiple stashes. Listing them allows you to review the stash entries and decide which one needs to be applied or dropped.
Explanation:
git stash list
: Displays all the stashes in the repository, showing details like the stash name, branch, and message attached.
Example Output:
stash@{0}: WIP on main: abc1234 Optional stash message
stash@{1}: WIP on feature-branch: def5678 Update feature X
Show the Changes as a Patch Between the Stash and Creation Commit
Code:
git stash show --patch stash@{0}
Motivation:
Reviewing stashed changes as a patch can be helpful to re-assess what exactly was stashed before deciding to apply, drop, or edit the changes.
Explanation:
git stash show
: Displays the changes contained in a stash.--patch
: Presents these changes as a patch, which includes comprehensive details of additions and deletions.stash@{0}
: Refers to the most recent stash entry. You can replace0
with another number to reference older stashes.
Example Output:
diff --git a/file.txt b/file.txt
index 83db48f..5219343 100644
--- a/file.txt
+++ b/file.txt
@@ -1,4 +1,4 @@
-Hello world
+Hello new feature
Apply a Stash
Code:
git stash apply {{optional_stash_name_or_commit}}
Motivation:
Stashes provide a non-linear development process. After saving your changes in a stash and switching branches, you might conclude your work and want to apply those changes to the current state.
Explanation:
git stash apply
: Reapplies changes from a specific stash without removing it from the stash list.{{optional_stash_name_or_commit}}
: Specifies a particular stash entry or commit to apply. If omitted, the latest stash (stash@{0}
) is applied.
Example Output:
Changes applied from stash@{0} successfully.
Drop or Apply a Stash and Remove It From the Stash List
Code:
git stash pop {{optional_stash_name}}
Motivation:
This command is useful when you’ve reviewed a stash and are confident about integrating it back into your work. It applies the stash and then deletes it, keeping your stash list tidy.
Explanation:
git stash pop
: Applies the stash and removes it afterward. This command helps in maintaining an organized stash list.{{optional_stash_name}}
: The specific stash to be applied and dropped. If omitted, the latest stash is used.
Example Output:
Applied stash@{0}
Dropped stash@{0}
Drop All Stashes
Code:
git stash clear
Motivation:
After a long project and multiple context switches, you may have accumulated numerous stashes. Once these stashes are considered redundant or no longer needed, clearing them outright can help declutter the stash list.
Explanation:
git stash clear
: This command deletes all stash entries, making it an effective clean-up operation when you are sure that no stashes are required anymore.
Example Output:
(No output if successful)
Conclusion
The git stash
command is an invaluable part of a developer’s toolkit, offering flexibility and control over the workspace to manage ongoing changes seamlessly. From stashing partial changes interactively to cleaning up all stashes in one go, understanding each of its functionalities allows for smoother project management, especially in dynamic work environments where priorities can change frequently.