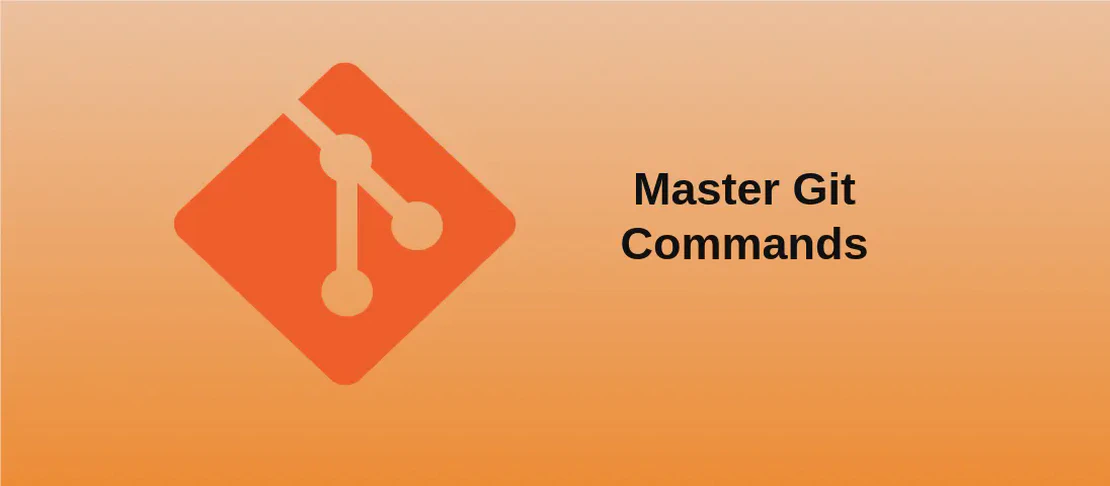
How to use the command 'git status' (with examples)
The git status
command is a fundamental tool in the Git version control system. It helps you keep track of the state of your working directory and the staging area. The command informs you about changes that have been made to the files, helping you understand what is staged for the next commit, what is not yet staged, and what files are not being tracked by Git. It is an essential part of the workflow that assists developers in ensuring their code changes are precisely managed and appropriately committed.
Use case 1: Show changed files which are not yet added for commit
Code:
git status
Motivation:
Using the simple git status
command is crucial for developers who want to quickly review the status of their working directory and staging area before making a commit. This command provides a snapshot of the current state without making any changes, thereby preventing mistakes such as forgetting to stage modified files or inadvertently including unwanted changes in a commit.
Explanation:
git status
: This simple command, when executed with no additional arguments, displays a comprehensive view of your repository’s status. It lists all the files that have been changed in your working directory, showing whether they are staged or not staged for commit. Additionally, it lists any untracked files that Git doesn’t have information about yet.
Example output:
On branch main
Your branch is up-to-date with 'origin/main'.
Changes not staged for commit:
(use "git add <file>..." to update what will be committed)
(use "git restore <file>..." to discard changes in working directory)
modified: example.txt
Untracked files:
(use "git add <file>..." to include in what will be committed)
newfile.txt
Use case 2: Give output in short format
Code:
git status --short
Motivation:
The short format of git status
is ideal for experienced developers who want a quick and condensed overview of the status of their files. This format provides a cleaner, less verbose summary, which is particularly useful when managing large projects with many changes, as it allows for rapid assessment without excessive detail.
Explanation:
--short
: This option simplifies the output ofgit status
, providing a concise format where each file’s status is indicated by a two-letter code. The first column shows the status of the staging area and the second column denotes the state of the working directory.
Example output:
M example.txt
?? newfile.txt
Use case 3: Show verbose information on changes in both the staging area and working directory
Code:
git status --verbose --verbose
Motivation:
The verbose option for git status
is useful for developers who need more detailed output, including the exact lines changed in modified files. This can be particularly useful during a thorough code review or when debugging complex changes, providing clarity on what adjustments have been made before they are committed.
Explanation:
--verbose
: By using the verbose option twice, the command provides an in-depth perspective on changes. This includes not only the file names of modified files but also a line-by-line breakdown of changes for files in the working directory and the staging area.
Example output:
On branch main
Your branch is up-to-date with 'origin/main'.
Changes not staged for commit:
(use "git add <file>..." to update what will be committed)
(use "git restore <file>..." to discard changes in working directory)
modified: example.txt
@@ -1 +1 @@
-Hello World
+Hello Git World
Use case 4: Show the branch and tracking info
Code:
git status --branch
Motivation:
Understanding your current branch status and its relationship with remote branches is crucial in distributed version control systems like Git. This command is beneficial when collaborating in teams, as it helps developers keep track of their current branch, its upstream branch, and whether it’s ahead or behind, ensuring everyone is in sync.
Explanation:
--branch
: This option augmentsgit status
by including additional information about the branch you’re currently on. It shows the relationship between your branch and its upstream branch in terms of commits, aiding in better management of branch synchronization with remote repositories.
Example output:
On branch main
Your branch is ahead of 'origin/main' by 2 commits.
(use "git push" to publish your local commits)
Use case 5: Show output in short format along with branch info
Code:
git status --short --branch
Motivation:
This combined format provides a compact view while still including critical branch information. It’s particularly useful when developers are switching between branches frequently and want to stay informed about their current branch status while maintaining a succinct overview of changes.
Explanation:
--short
: Produces a minimized version of the status display.--branch
: Adds branch information, such as the current branch name and its commit relation to the upstream branch, integrated into this concise format.
Example output:
## main...origin/main [ahead 2]
M example.txt
?? newfile.txt
Use case 6: Show the number of entries currently stashed away
Code:
git status --show-stash
Motivation:
Keeping track of stashed changes is essential for developers who frequently use the stash feature to save unfinished work. This command helps by displaying how many stash entries are available, ensuring that developers do not forget about their stashed updates and can manage them effectively.
Explanation:
--show-stash
: This option extends thegit status
output to include the number of stashes saved. It’s beneficial for developers looking to manage their stashes efficiently, especially in workflows involving frequent context switches.
Example output:
On branch main
Your branch is up-to-date with 'origin/main'.
Stash entries: 2
Use case 7: Don’t show untracked files in the output
Code:
git status --untracked-files=no
Motivation:
Dealing with numerous untracked files can become distracting, especially in large projects or when performing repetitive tests that generate temporary files. This command allows developers to focus solely on tracked files’ status, removing clutter and making it easier to comprehend changes that affect the version-control system.
Explanation:
--untracked-files=no
: Prevents untracked files from appearing in thegit status
output. It’s useful for simplifying the display, ensuring developers see only the tracked files that have been modified, thus providing a cleaner view.
Example output:
On branch main
Your branch is up-to-date with 'origin/main'.
Changes not staged for commit:
(use "git add <file>..." to update what will be committed)
modified: example.txt
Conclusion:
The git status
command, with its various options, is an indispensable tool for developers using Git. It provides a comprehensive understanding of the current state of a repository, facilitating better management of code changes. From basic assessments with the standard output to more detailed analyses using verbose settings, each use case of git status
serves a distinct purpose, enhancing the workflow of tracking and committing changes efficiently.