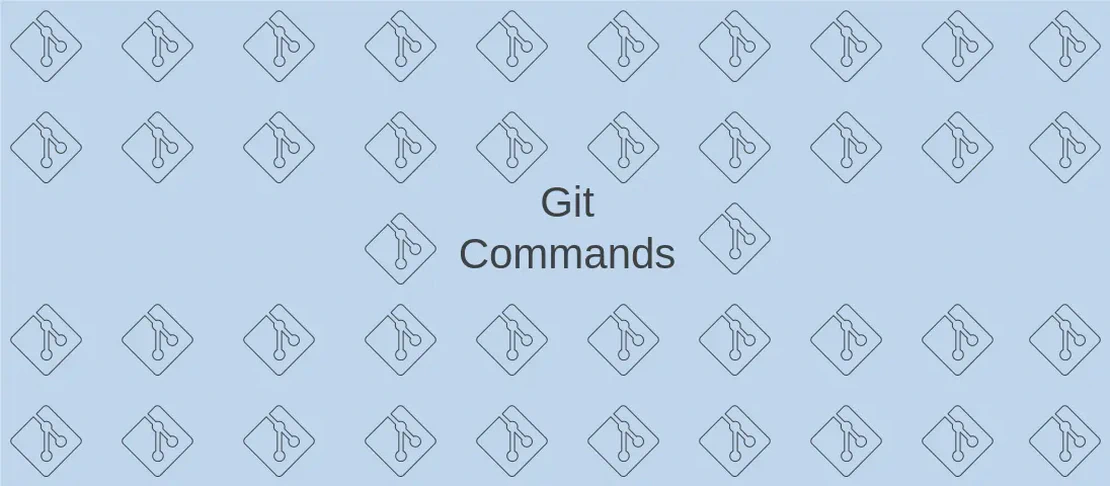
Mastering Git Submodules (with examples)
Git submodules serve as an essential tool for managing and integrating external repositories within your main project repository. They allow developers to keep a clean and organized structure by cloning dependencies as submodules, enabling better collaboration and version control. Submodules are particularly useful for projects that rely on third-party code or a shared internal library, offering precise control over which versions are included in your project. Let’s dive into some specific use cases to understand how to leverage Git submodules effectively.
Use case 1: Install a repository’s specified submodules
Code:
git submodule update --init --recursive
Motivation:
When you’re working with a project that includes submodules, cloning the main repository doesn’t automatically include these submodules. They are pointers to specific commits in other repositories. To ensure your working directory includes all the necessary code from these submodules, you need to initialize and update them. This command efficiently sets up the submodules after cloning a repository, saving you from any manual submodule configuration, which can be error-prone and tedious.
Explanation:
update
: This part of the command is telling Git to update the submodules to match what the superproject expects.--init
: Before you can use a submodule, you have to initialize it. This argument reads the.gitmodules
file in your repository and clones all submodules listed there.--recursive
: This ensures that any nested submodules (submodules within other submodules) are also initialized and updated, pulling down everything required for a complete codebase setup.
Example output:
Submodule 'libs/external-module' (https://github.com/user/external-module.git) registered for path 'libs/external-module'
Cloning into '/path/to/repository/libs/external-module'...
Submodule path 'libs/external-module': checked out 'abcdef123456789'
Use case 2: Add a Git repository as a submodule
Code:
git submodule add repository_url
Motivation:
In software development, you might want to use a portion of another git repository, such as a library or a shared component, inside your current project. Adding it as a submodule makes it easy to track changes in that dependency without copying files directly into your repository. This keeps your project manageable and helps in ensuring any new updates to the submodule can be easily integrated.
Explanation:
add
: This command initializes and adds the specified repository as a submodule.repository_url
: This is the URL of the repository you want to add as a submodule. Git will use this address to clone the submodule into your project.
Example output:
Cloning into 'external-module'...
remote: Enumerating objects: 100, done.
remote: Counting objects: 100% (100/100), done.
remote: Compressing objects: 100% (70/70), done.
remote: Total 100 (delta 20), reused 60 (delta 10), pack-reused 0
Submodule 'external-module' (https://github.com/user/external-module.git) registered for path 'external-module'
Use case 3: Add a Git repository as a submodule at the specified directory
Code:
git submodule add repository_url path/to/directory
Motivation:
Organizing your project directory structure is crucial for maintaining clarity and separating concerns. If you’re working with multiple submodules, you might want to structure them in specific directories for organization. This command allows you to control exactly where a submodule’s files will reside within your project, ensuring they coexist peacefully with other project components.
Explanation:
add
: Adds the repository as a submodule to your project.repository_url
: The URL of the repository you want to add.path/to/directory
: The specific directory path in your project where you want the submodule files to reside. This allows for custom organization beyond the default approach.
Example output:
Cloning into '/path/to/repository/path/to/directory'...
remote: Enumerating objects: 150, done.
remote: Counting objects: 100% (150/150), done.
remote: Compressing objects: 100% (100/100), done.
remote: Total 150 (delta 30), reused 90 (delta 15), pack-reused 0
Submodule 'custom-dir/module' (https://github.com/user/external-module.git) registered for path 'path/to/directory'
Use case 4: Update every submodule to its latest commit
Code:
git submodule foreach git pull
Motivation:
Submodules point to specific commits in their respective repositories. However, there might be cases where you want to ensure that all submodules are updated to their latest commits, reflecting the most recent developments. This is particularly useful in active projects where dependencies regularly receive updates. It helps keep your project aligned with the latest changes in your dependencies without manually updating each one.
Explanation:
foreach
: This instructs Git to perform the specified command in each submodule.git pull
: This is the command executed within each submodule, fetching changes from the remote repository and updating the current branch.
Example output:
Entering 'submodule1'
Already up to date.
Entering 'submodule2'
Updating a1b2c3d..d4e5f6g
Fast-forward
example-file.txt | 2 +-
1 file changed, 1 insertion(+), 1 deletion(-)
Conclusion:
Git submodules provide an effective strategy for managing external dependencies within a repository without inflating the codebase. By allowing for precise version control and organization, submodules improve project scalability and facilitate collaboration. Through the use of categorized commands, developers can seamlessly integrate, update, and manage submodules, harnessing the full potential of Git’s capabilities. Understanding these examples will enable you to implement submodules confidently, ensuring your project remains robust and streamlined.