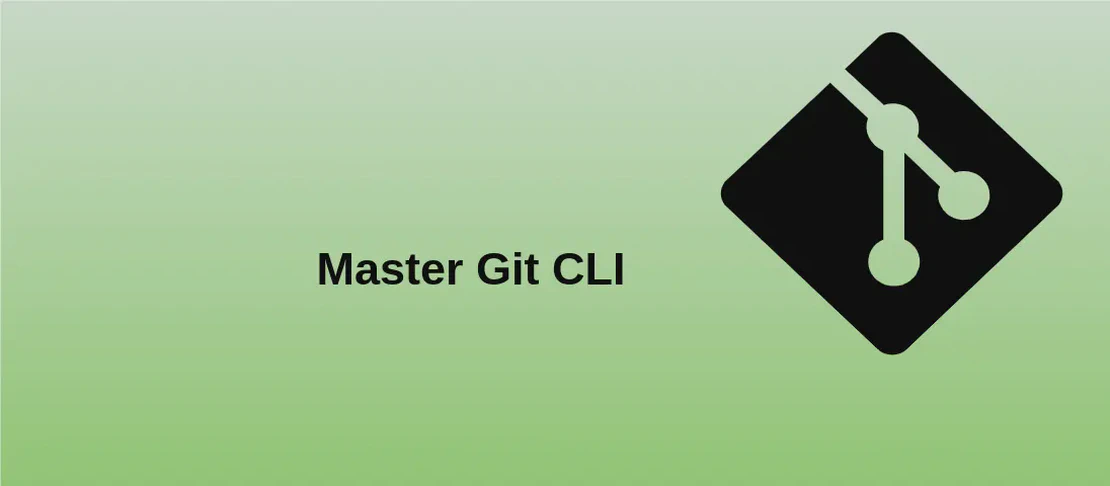
How to use the command 'git subtree' (with examples)
The git subtree
command is a powerful tool within the Git version control system that allows developers to manage project dependencies by embedding other Git repositories directly inside a directory of the main repository. This provides a seamless way to incorporate third-party libraries, share code between different projects, or manage large monolithic codebases modularly. By using subtrees, you sidestep some of the complexities and constraints associated with submodules, such as their separate history and independence from the main project’s lifecycle.
Use case 1: Add a Git repository as a subtree
Code:
git subtree add --prefix=path/to/directory/ --squash repository_url branch_name
Motivation:
When you’re working on a project that relies on an external library or module that is hosted in a separate Git repository, you might want to include it in your project seamlessly. Using git subtree add
allows you to do just that by embedding the external repository in a specific directory within your project, integrating it directly into your project’s workflow.
Explanation:
--prefix=path/to/directory/
: This argument specifies the directory in your working directory where you want to add the subtree. It essentially acts as the container for the external repository.--squash
: This option combines all the changes from the added branch into a single commit, simplifying your project’s history by minimizing the clutter from the subtree’s history.repository_url
: This is the URL of the external Git repository that you want to include in your project.branch_name
: Specifies the branch from the external repository that you want to add as a subtree.
Example Output:
When executed, this command would check out the specified branch from the external repository, squash its history, and place it within the defined directory of your project. You might see output indicating the fetching of the branch, squashing commits, and finalization of adding the subtree.
Use case 2: Update subtree repository to its latest commit
Code:
git subtree pull --prefix=path/to/directory/ repository_url branch_name
Motivation:
Over time, the external repository included in your project may receive updates or bug fixes. To keep your project up-to-date with the latest changes in the external repository, you can use git subtree pull
. This ensures that your project benefits from enhancements and maintains compatibility with the external code.
Explanation:
--prefix=path/to/directory/
: Specifies the directory of your project where the subtree is located and needs to be updated.repository_url
: The URL of the external Git repository containing the updates you want to pull.branch_name
: Specifies which branch from the external repository you want to pull the latest commits from.
Example Output:
The command output would show the process of pulling in the latest commits from the external repository’s branch into your subtree directory, resolving any merge conflicts that may arise, and updating your project accordingly.
Use case 3: Merge recent changes up to the latest subtree commit into the subtree
Code:
git subtree merge --prefix=path/to/directory/ --squash repository_url branch_name
Motivation:
There might be scenarios where you’ve made changes to your project that you need to integrate back into the subtree or vice versa before updating. By using git subtree merge
, you can combine changes effortlessly, ensuring both the main project and the subtree remain in sync.
Explanation:
--prefix=path/to/directory/
: Indicates the directory where the subtree resides within your project.--squash
: Collapses all the updates into a single merge commit to keep the project’s history clean.repository_url
: The URL of the subtree repository you are merging changes from.branch_name
: Defines the branch from which you are merging updates.
Example Output:
As this command runs, you can expect a readout of the merge process, detailing file changes, any conflicts encountered, and the successful or unsuccessful integration of updates into the subtree.
Use case 4: Push commits to a subtree repository
Code:
git subtree push --prefix=path/to/directory/ repository_url branch_name
Motivation:
If you’ve made meaningful improvements or fixes to the code within your subtree, these may need to trickle back into the original external repository. Using git subtree push
, you can push your local commits from your subtree back to a branch in the external repository, fostering collaboration and sharing improvements.
Explanation:
--prefix=path/to/directory/
: The path indicates which part of your project should be pushed to the external repository.repository_url
: The URL of the target external Git repository you wish to update.branch_name
: Denotes the branch in the target repository to which changes will be pushed.
Example Output:
The command would connect to the specified repository and branch, pushing the local subtree commits. You might see logs detailing which objects are being pushed and confirmation once completed.
Use case 5: Extract a new project history from the history of a subtree
Code:
git subtree split --prefix=path/to/directory/ repository_url -b branch_name
Motivation:
Sometimes, you may want to spin off part of your project into a new or separate repository for modularization or to distribute it independently. By using git subtree split
, it becomes possible to create a clean history for just the section of your project contained within the subtree directory.
Explanation:
--prefix=path/to/directory/
: Specifies the directory whose history you wish to split into a new branch.repository_url
: Defines the repository containing the existing project history.-b branch_name
: Indicates the creation of a new branch with history derived exclusively from the subtree directory.
Example Output:
Once executed, the command would return the SHA1 hash of the new commit that represents the root of your newly created branch. The command output typically includes a clear process of building the new history from your specified prefix.
Conclusion:
Git subtree is a versatile command offering various functionalities to integrate, update, and manage external repositories within your project. By understanding and utilizing the commands shared in these examples, developers can achieve better modularization, dependency management, and collaboration across multiple projects and teams.