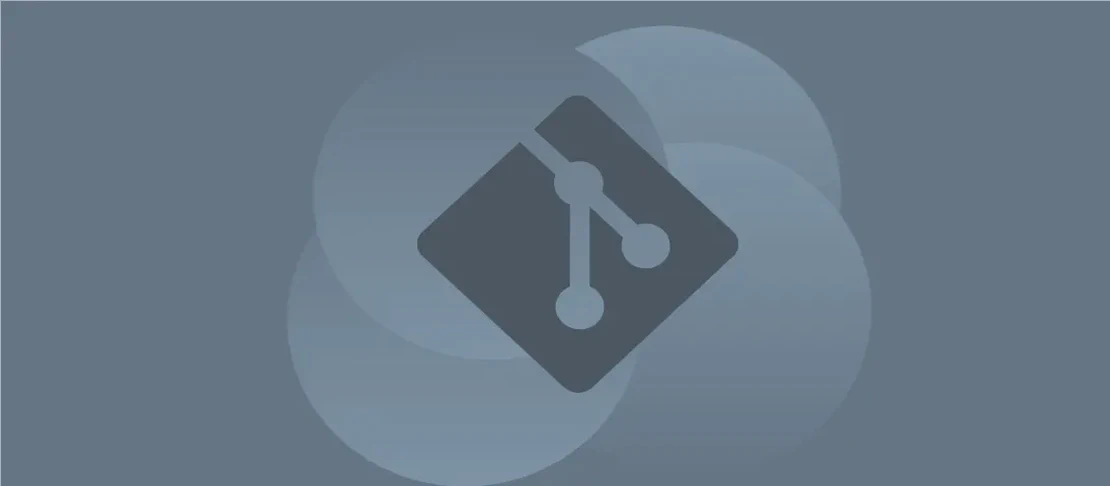
How to use the command 'git switch' (with examples)
The git switch
command is used to switch between Git branches. It is a more modern and user-friendly alternative to the git checkout
command. It requires Git version 2.23 or higher.
Use case 1: Switch to an existing branch
Code:
git switch branch_name
Motivation: This use case allows you to switch to an existing branch in your Git repository. It is useful when you want to start working on a different branch and continue your development or collaboration efforts.
Explanation:
branch_name
: The name of the branch you want to switch to.
Example output:
Switched to branch 'branch_name'
Use case 2: Create a new branch and switch to it
Code:
git switch --create branch_name
Motivation: This use case is helpful when you want to create a new branch and immediately switch to it. It allows you to work on a feature or fix without altering the state of the current branch.
Explanation:
--create
: Specifies that a new branch should be created.branch_name
: The name of the new branch.
Example output:
Switched to a new branch 'branch_name'
Use case 3: Create a new branch based on an existing commit and switch to it
Code:
git switch --create branch_name commit
Motivation: This use case is useful when you want to create a new branch that starts at a specific commit. It allows you to work on a branch based on a specific state of your project’s history.
Explanation:
--create
: Specifies that a new branch should be created.branch_name
: The name of the new branch.commit
: The commit ID or ref to base the new branch on.
Example output:
Switched to a new branch 'branch_name'
Use case 4: Switch to the previous branch
Code:
git switch -
Motivation: This use case enables you to quickly switch back to the previous branch you were on. It is helpful when you need to make changes or continue your work on a branch you were previously working on.
Explanation:
-
: Represents the previous branch you were on.
Example output:
Switched to branch 'previous_branch'
Use case 5: Switch to a branch and update all submodules to match
Code:
git switch --recurse-submodules branch_name
Motivation: When working with submodules in Git, this use case allows you to switch to a branch and update all submodules within the repository. It ensures that the submodules are aligned with the specified branch.
Explanation:
--recurse-submodules
: Specifies that all submodules should be updated.branch_name
: The name of the branch you want to switch to.
Example output:
Switched to branch 'branch_name'
Updating submodules...
Use case 6: Switch to a branch and automatically merge the current branch and any uncommitted changes into it
Code:
git switch --merge branch_name
Motivation: This use case is helpful when you want to switch to a branch and automatically merge the changes from the current branch and any uncommitted changes. It simplifies the process of incorporating your changes into the new branch.
Explanation:
--merge
: Specifies that the current branch and any uncommitted changes should be merged into the new branch.branch_name
: The name of the branch you want to switch to.
Example output:
Switched to branch 'branch_name'
Merging changes...
Conclusion
The git switch
command is a powerful tool for managing branches in Git. It allows you to switch branches, create new branches, update submodules, and merge changes effortlessly. Understanding the different use cases and their respective arguments enables efficient and effective branch management in your Git workflow.