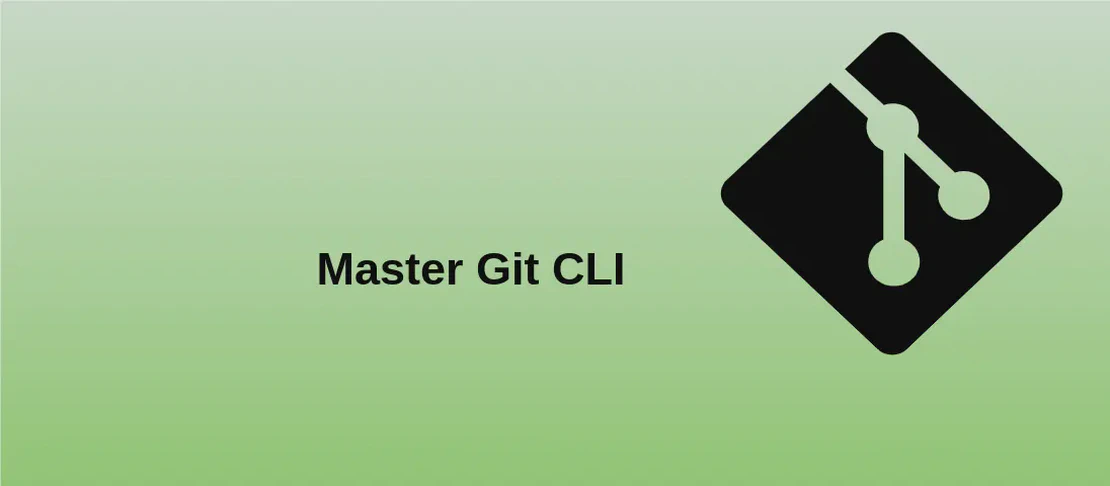
How to use the command 'git tag' (with examples)
The git tag
command is used to create, list, delete, or verify tags in Git. A tag is a static reference to a specific commit. This command is useful for adding additional information to specific commits and organizing them for easier reference.
Use case 1: List all tags
Code:
git tag
Motivation: By listing all the tags in the repository, you can quickly see which commits have been tagged. This can be useful for identifying significant milestones or releases in the project.
Explanation: This command simply lists all the tags in the repository.
Example output:
tag1
tag2
tag3
Use case 2: Create a tag pointing to the current commit
Code:
git tag tag_name
Motivation: Creating a tag pointing to the current commit can help mark important points in the development process, such as stable releases or major feature additions.
Explanation: This command creates a tag with the given tag_name
pointing to the current commit.
Example output: No output is provided when creating a tag.
Use case 3: Create a tag pointing to a given commit
Code:
git tag tag_name commit
Motivation: By tagging a specific commit, you can easily reference it in the future or share it with others.
Explanation: This command creates a tag with the given tag_name
pointing to the specified commit
.
Example output: No output is provided when creating a tag.
Use case 4: Create an annotated tag with a message
Code:
git tag tag_name -m tag_message
Motivation: Annotated tags provide additional information such as release notes or context for a specific commit.
Explanation: This command creates an annotated tag with the given tag_name
and attaches the provided tag_message
to it.
Example output: No output is provided when creating a tag.
Use case 5: Delete a tag
Code:
git tag -d tag_name
Motivation: Deleting a tag can be necessary if the tag is no longer relevant or if you made a mistake when creating it.
Explanation: This command deletes the tag with the given tag_name
.
Example output: No output is provided when deleting a tag.
Use case 6: Get updated tags from upstream
Code:
git fetch --tags
Motivation: Fetching updated tags from upstream allows you to stay up to date with any new or deleted tags.
Explanation: This command fetches the updated tags from the remote repository and updates the local repository to reflect those changes.
Example output: No output is provided when fetching updated tags.
Use case 7: List tags containing a specific commit
Code:
git tag --contains commit
Motivation: Listing all the tags that contain a specific commit can help track down which releases or versions include that specific commit.
Explanation: This command lists all the tags whose ancestors include the specified commit
.
Example output:
tag1
tag2
Conclusion:
The git tag
command provides a range of functionalities for working with tags in Git. By utilizing these different use cases, you can effectively organize and reference specific commits within your repository. Whether it’s creating tags pointing to significant milestones or fetching updated tags from the remote repository, the git tag
command is a valuable tool in managing your Git workflow.