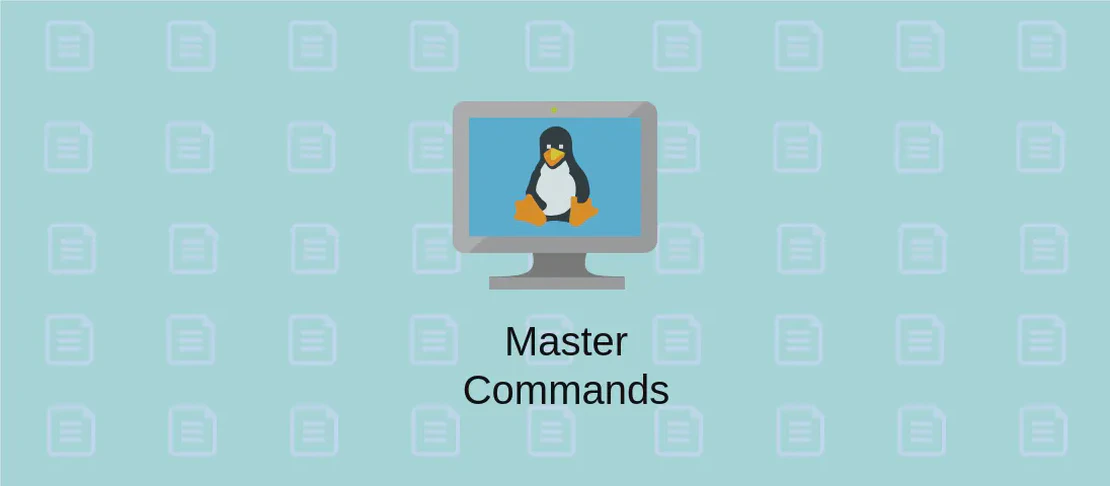
How to Use the Command 'go build' (with Examples)
The ‘go build’ command is an essential part of the Go programming language toolchain. It compiles packages and generates executable binaries from Go source code. Designed to be straightforward yet powerful, ‘go build’ ensures that developers can reliably compile their programs, verifying that all dependencies and packages are correctly assembled into a functional application.
Using ‘go build’, you can compile individual files, entire packages, and even enable additional runtime features like data race detection. This article demonstrates four key use cases of the ‘go build’ command with detailed examples.
Use case 1: Compile a ‘package main’ file
Code:
go build path/to/main.go
Motivation:
This is a common operation when you have a single Go source file that contains the ‘package main’ and a ‘main’ function, the entry point for Go applications. Often used during development, running this command helps developers quickly compile the file to check for any compilation errors and generate an executable with a default name.
Explanation:
go
: This is the Go tool itself, which facilitates operations like building, testing, and running Go programs.build
: A sub-command of the Go tool that compiles the package and dependencies.path/to/main.go
: The path to the main Go source file which typically contains the ‘main’ package. Here, the path is specified to direct the command to the correct file for compilation.
Example Output:
Once executed, this command generates an executable binary in the same directory. The output binary will be named after the Go source file (without the .go extension).
Use case 2: Compile, specifying the output filename
Code:
go build -o path/to/binary path/to/source.go
Motivation:
There are times when developers need to specify the exact name and location of the compiled binary. This is especially useful in continuous integration and deployment pipelines, where naming consistency of binaries is critical for subsequent processes.
Explanation:
-o path/to/binary
: Specifies the path and name of the output binary. The-o
flag stands for “output”.path/to/source.go
: The source file to compile.
Example Output:
The specified binary will appear at path/to/binary
, allowing integration with other tools or simply ensuring that the binary is generated in a particular location with a specific name.
Use case 3: Compile a package
Code:
go build -o path/to/binary path/to/package
Motivation:
In Go, a package can consist of multiple files, all of which contribute to creating a larger program or library. Compiling the entire package into a single binary is crucial for testing and distribution. This scenario is perfect when you want to build a re-usable component or service, not just an executable.
Explanation:
-o path/to/binary
: Directs the output to a specified file.path/to/package
: Refers to the directory containing the Go package source files.
Example Output:
Once executed, the command compiles all Go files in path/to/package
directory into a singular binary named as specified.
Use case 4: Compile a main package into an executable, enabling data race detection
Code:
go build -race -o path/to/executable path/to/main/package
Motivation:
Data races can lead to unpredictable behaviors and bugs in concurrent programs. Using the ‘-race’ flag during development helps identify data races by detecting unconventional writes to memory. This serves as a preventive measure, making it easier to catch complex concurrency issues early.
Explanation:
-race
: Enables the race detector, which identifies data race conditions at runtime.-o path/to/executable
: Sets the output name and location for the compiled executable.path/to/main/package
: Points to the main package directory that should be converted into an executable.
Example Output:
Running this command generates an executable with data race detection enabled. The output lets developers identify potential data race issues, with errors reported during runtime if races are encountered.
Conclusion:
The ‘go build’ command is a foundational tool for developers working in the Go programming ecosystem, providing flexibility and functionality to handle various build scenarios. Understanding how to leverage its capabilities across different use cases can dramatically enhance your development and build processes, ultimately leading to more efficient and reliable Go applications.