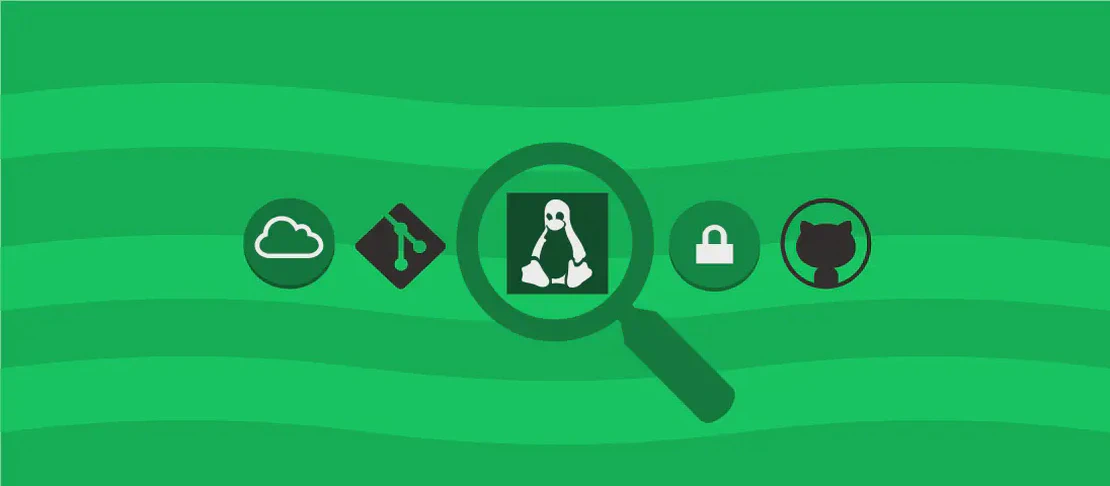
How to Use the Command 'go clean' (with Examples)
The go clean
command in Go is a utility that assists developers in maintaining a clean development environment by removing unnecessary files. Specifically, it deletes object files and cached data that accumulate during the software development process. This aids in managing disk space and ensures that any issues due to stale files are mitigated, promoting a smoother development workflow.
Use Case 1: Print the Remove Commands Instead of Actually Removing Anything
Code:
go clean -n
Motivation:
This use case is crucial for developers who want to understand what files would be removed without actually deleting anything. It’s particularly beneficial when you want to ensure that executing a go clean
command won’t inadvertently delete necessary files.
Explanation:
-n
: The-n
flag stands for “no action”. When this flag is specified,go clean
will print the remove commands it intends to execute without performing the actual file deletions. This is a safeguard mechanism allowing developers to review the operations before execution.
Example Output:
rm -rf ./bin
rm -f ./file.o
rm -f ./main
Use Case 2: Delete the Build Cache
Code:
go clean -cache
Motivation:
Over time, build caches can consume a significant amount of disk space, especially in large and long-running projects. Cleaning the build cache helps reclaim disk space and can resolve issues stemming from corrupted cache files.
Explanation:
-cache
: This flag instructsgo clean
to target and delete the build cache specifically. The build cache in Go is used to store previous build outputs to speed up incremental builds. Sometimes, however, deleting this cache may be necessary to ensure a clean state if the cache gets corrupted or disk space becomes a concern.
Example Output:
cache: clearer output indicating files or bytes removed
Use Case 3: Delete All Cached Test Results
Code:
go clean -testcache
Motivation:
Test caches in Go are used to store test results from previous test runs. Over multiple test iterations, these can grow and potentially harbor outdated or incorrect test results. Clearing the test cache ensures that subsequent test runs are fresh and based solely on the latest codebase.
Explanation:
-testcache
: This flag targets cached test results, deleting them to ensure that only newly run tests reflect in any future testing results. This helps avoid confusion caused by potentially incorrect or obsolete test result outputs.
Example Output:
testcache: 5 files removed
Use Case 4: Delete the Module Cache
Code:
go clean -modcache
Motivation:
The module cache stores copies of downloaded modules and packages. While this cache speeds up dependency retrieval in Go builds, it can occupy substantial disk space. If there’s a need to recover space or resolve dependency issues, clearing the module cache is effective.
Explanation:
-modcache
: This flag instructsgo clean
to delete all files in the module cache. It’s beneficial when developers need to resolve dependency conflicts or need a clean slate for module downloads.
Example Output:
modcache: deletion complete, cache cleared
Conclusion:
The go clean
command is a powerful tool for Go developers, offering options to manage and clean disk space effectively by eliminating stale or unnecessary files. Whether you’re reviewing what would be removed with the -n
flag or reclaiming storage by clearing caches, understanding and using these options can lead to a more efficient and problem-free development process.