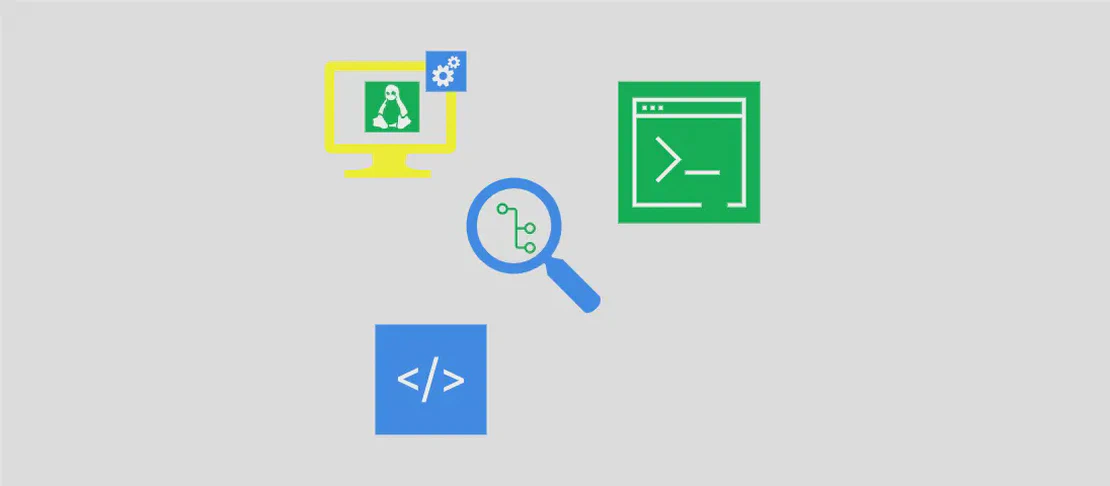
How to Use the `go` Command (with Examples)
The Go programming language, often referred to as Golang, is renowned for its efficiency, simplicity, and robustness. The go
command is the primary tool for managing Go source code, carrying out functions such as building executable binaries, running code, managing dependencies, and running tests. This comprehensive guide will illustrate several practical use cases of the go
command.
Use Case 1: Download and Install a Package
Code:
go get package_path
Motivation:
When working on a Go project, you often need to import third-party libraries to utilize specific functions or features that are not part of the core language. The go get
command is employed to download and install these libraries, ensuring they are available as part of your project’s dependencies.
Explanation:
go get
: This command tells the Go tool to download a package. It fetches the required Go package from version control repositories and updates your project’s go.mod file.package_path
: This argument is the import path of the package you’re interested in. It usually follows the formatgithub.com/user/repo
, representing where the package is hosted.
Example Output:
go: finding module for package github.com/example/package
go: downloading github.com/example/package v1.0.0
go: found github.com/example/package in github.com/example/package v1.0.0
Use Case 2: Compile and Run a Source File
Code:
go run file.go
Motivation:
During the development phase, you regularly need to execute Go files to test and verify their functionality. The go run
command is particularly useful as it compiles and executes the Go source file in one go, facilitating quick testing cycles.
Explanation:
go run
: This command compiles and executes the Go program specified.file.go
: This is the file containing your Go source code. It should belong to themain
package and declare amain
function for execution.
Example Output:
Hello, World!
Use Case 3: Compile a Source File into a Named Executable
Code:
go build -o executable file.go
Motivation:
There are scenarios where you need to compile your Go code into a standalone executable file, which can then be distributed or executed independently. The go build
command is used to compile code into a binary, while the -o
flag specifies the output’s name.
Explanation:
go build
: This command compiles the packages named by the import paths.-o executable
: The-o
flag allows you to name the resulting executable file.file.go
: This is the source file that you are compiling.
Example Output:
No output; instead, an executable file named executable
is created in your working directory.
Use Case 4: Compile the Package Present in the Current Directory
Code:
go build
Motivation:
When your Go project spans multiple source files in a directory, you might want to compile the entire package instead of just a single file. The go build
command executed without arguments compiles the package found in the current directory.
Explanation:
go build
: By running this command without additional arguments, it compiles all Go source files in the current directory that are part of the package, producing an executable if they belong to themain
package.
Example Output: No output; an executable is created in the current directory if applicable.
Use Case 5: Execute All Test Cases of the Current Package
Code:
go test
Motivation:
Testing is critical in software development to ensure code correctness and functionality. Go provides an inbuilt test framework, allowing you to write test cases in files ending with _test.go
. The go test
command runs these tests to validate your code.
Explanation:
go test
: This command runs the tests defined in the_test.go
files of the current package. It searches for files containing testing functions and executes them.
Example Output:
PASS
ok your/package/path 0.005s
Use Case 6: Compile and Install the Current Package
Code:
go install
Motivation:
Once development and testing are complete, you may need to install your Go package in your workspace or binary path. The go install
command compiles and installs the Go package, making it conveniently executable from the command line.
Explanation:
go install
: This command compiles the package and moves the output binary to the$GOPATH/bin
directory, making it easily accessible in your system’s PATH.
Example Output:
No output; the result is an installed executable or library in $GOPATH/bin
.
Use Case 7: Initialize a New Module in the Current Directory
Code:
go mod init module_name
Motivation:
When starting a new Go project, initializing a module is crucial for managing dependencies using Go modules. The go mod init
command creates a new module by generating a go.mod
file, serving as the dependency manifest for the project.
Explanation:
go mod init
: This command initializes a new module in the current directory.module_name
: This specifies the module path, which is often the URL where the module source code is hosted.
Example Output:
go: creating new go.mod: module module_name
Conclusion
The go
command is an essential toolset in the arsenal of any Go developer. Each subcommand, from building and testing to installing and managing dependencies, enables developers to efficiently handle various aspects of programming in Go. Understanding these use cases enhances productivity and streamlines the software development lifecycle in Golang projects.