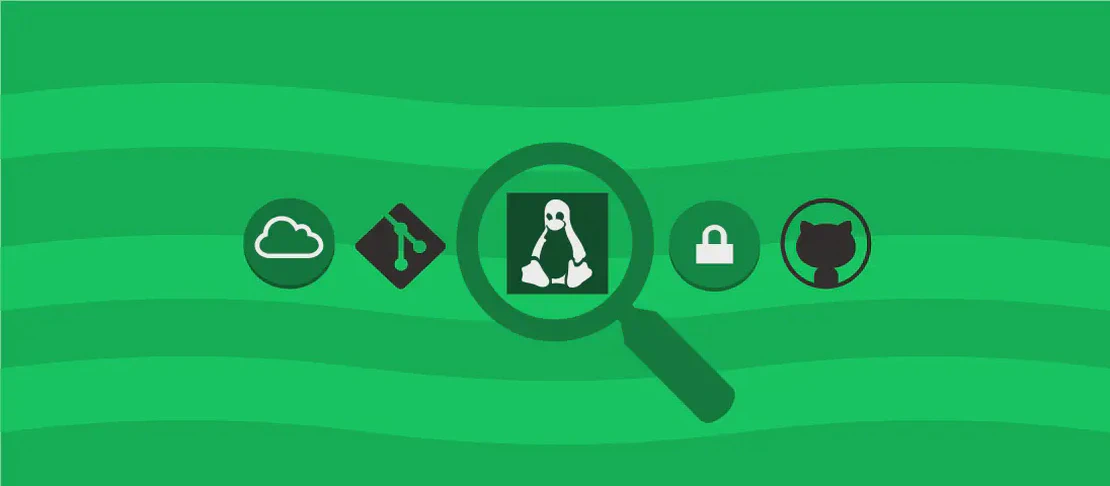
How to Use the 'go doc' Command (with Examples)
The go doc
command is a tool integrated into the Go programming language ecosystem, designed to fetch and display the documentation for Go packages and their constituent symbols. It serves as an invaluable resource for developers seeking to understand the functionalities of different packages, examine key symbols, or peruse the implementation details directly from the source code. This guide will review various applications of the go doc
command to highlight its versatility and utility.
Use Case 1: View Documentation for the Current Package
Code:
go doc
Motivation: This basic command is useful when you want to quickly review the documentation of the package in which you are currently working. By executing go doc
without any arguments, it assumes the current working directory contains your package and presents its documentation accordingly, thus allowing you to verify or refresh your understanding of its components on the fly.
Explanation: The command go doc
is typically run in the directory of the Go package you wish to inquire about. It automatically looks at the package in the present working directory and provides details about it, such as a top-level description and key usages.
Example Output:
package main // import "example.com/mypackage"
FUNCTIONS
func MyFunction()
Use Case 2: Show Package Documentation and Exported Symbols
Code:
go doc encoding/json
Motivation: When working with well-known packages like encoding/json
, it’s beneficial to explore all available functions, types, and symbols. This use case is particularly helpful if you’re starting to integrate a new package for a project and need a comprehensive overview of what the package offers.
Explanation: By specifying encoding/json
, you direct go doc
to provide the top-level documentation for the package, including any exported (publicly accessible) symbols such as functions, variables, and structs. This insight can accelerate your understanding of the package functionalities.
Example Output:
package json // import "encoding/json"
Package json implements encoding and decoding of JSON as defined in RFC 7159.
FUNCTIONS
func Marshal(v interface{}) ([]byte, error)
func Unmarshal(data []byte, v interface{}) error
TYPES
type Decoder
...
Use Case 3: Show Also Documentation of Symbols
Code:
go doc -all encoding/json
Motivation: Sometimes, a high-level overview is insufficient. When you need detailed documentation of all symbols within a package, including unexported ones, using the -all
flag provides a treasure trove of information that could make or break your understanding or debugging process.
Explanation: The -all
option extends the go doc
output to include exhaustive documentation not only of exported symbols but also the internal ones, thereby presenting a more complete picture of what the package encapsulates. This could be pivotal during in-depth code reviews or when attempting to extend or modify package functionality.
Example Output:
package json // import "encoding/json"
Package json implements encoding and decoding of JSON.
CONSTANTS
...
FUNCTIONS
func Marshal(v interface{}) ([]byte, error)
Marshal returns the JSON encoding of v.
...
UNEXPORTED SYMBOLS
...
Use Case 4: Show Also Sources
Code:
go doc -all -src encoding/json
Motivation: In advanced scenarios, you might need to dive deep into the source code to understand how a package operates or to modify it as per your project requirements. This command captures not only what a function does but also the exact implementation, which is crucial for any modification tasks or in-depth understanding.
Explanation: By adding the -src
flag to the command with -all
, you retrieve the source code behind every documented symbol. This inclusion of source code transforms go doc
into a powerful tool for any Go developer like an open book of code.
Example Output:
package json // import "encoding/json"
// Marshal returns the JSON encoding of v.
func Marshal(v interface{}) ([]byte, error) {
...
}
Use Case 5: Show a Specific Symbol
Code:
go doc -all -src encoding/json.Number
Motivation: In many instances, you may only be interested in a specific part of a package, such as a certain type or symbol. By directing your query to encoding/json.Number
, you filter the results to a pinpointed piece of interest, saving you time and focusing your attention.
Explanation: The command limits the search to the specific symbol Number
within the encoding/json
package. With the -all
and -src
flags, not only do you get complete documentation on Number
, but you also delve directly into its source code. This specificity is ideal for troubleshooting, understanding complex type behaviors, or auditing code for correctness.
Example Output:
package json // import "encoding/json"
type Number string
A Number represents a JSON number literal.
...
Conclusion
The go doc
command is an intricate part of the Go tooling environment. Through its various flags and options, it provides multiple levels of detail, from a simple package overview to detailed source code inspections. Its utility spans from everyday development tasks to detailed code investigation, making it an essential tool in a Go programmer’s repertoire. By understanding how to effectively leverage each aspect of go doc
, developers can enhance their coding efficiency and deepen their understanding of Go packages.