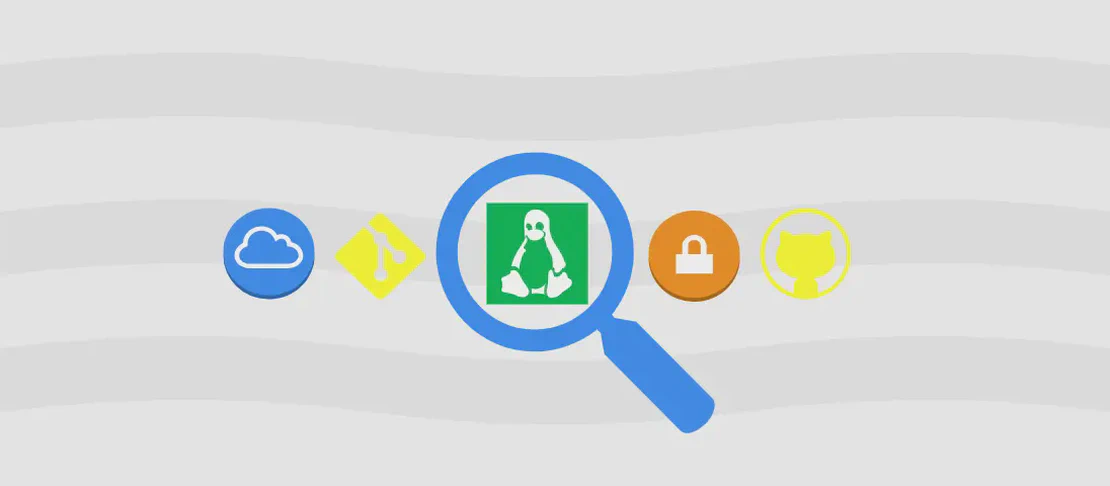
How to use the command `go fmt` (with examples)
The go fmt
command is used to format Go source files. It automatically updates the formatting of the source code according to the standard Go formatting rules. This helps to ensure consistent and readable code across projects.
Use case 1: Format Go source files in the current directory
Code:
go fmt
Motivation:
Running go fmt
without any arguments will format all Go source files in the current directory. This is useful when you want to quickly update the formatting of all source files in your project.
Explanation:
go
: The Go command.fmt
: The subcommand for formatting Go source files.
Example output:
main.go
util.go
Use case 2: Format a specific Go package in your import path ($GOPATH/src
)
Code:
go fmt path/to/package
Motivation: Sometimes, you may only want to format a specific Go package instead of all the packages in your project. This allows you to selectively format the parts of your codebase that you are working on, without affecting the entire project.
Explanation:
go
: The Go command.fmt
: The subcommand for formatting Go source files.path/to/package
: The import path of the package you want to format. This should be relative to$GOPATH/src
.
Example output:
path/to/package/source.go
Use case 3: Format the package in the current directory and all subdirectories (note the ...
)
Code:
go fmt ./...
Motivation:
When you have a large project with multiple nested packages, running go fmt
on each package individually can be cumbersome. The ./...
argument allows you to format the package in the current directory and all its subdirectories at once.
Explanation:
go
: The Go command.fmt
: The subcommand for formatting Go source files../...
: Represents all packages in the current directory and its subdirectories.
Example output:
./main.go
./pkg/util.go
./pkg/api/handler.go
Use case 4: Print what format commands would’ve been run, without modifying anything
Code:
go fmt -n
Motivation:
Before making any changes to your code, it can be beneficial to preview the formatting changes that will be applied. The -n
flag allows you to simulate the formatting process without actually modifying anything.
Explanation:
go
: The Go command.fmt
: The subcommand for formatting Go source files.-n
: The flag to print the format commands that would have been run.
Example output:
gofmt -w main.go
gofmt -w util.go
Use case 5: Print which format commands are run as they are run
Code:
go fmt -x
Motivation:
When you want to see the details of the formatting process, including which files are being formatted and where they are located, you can use the -x
flag. This provides a more verbose output during the formatting process.
Explanation:
go
: The Go command.fmt
: The subcommand for formatting Go source files.-x
: The flag to print the format commands as they are run.
Example output:
gofmt -w main.go
gofmt -w util.go
Conclusion:
The go fmt
command is a powerful tool for automatically formatting Go source files. Whether you want to format a single package, multiple packages, or the entire project, go fmt
provides flexibility and simplicity in improving the code readability and maintainability. Additionally, the additional flags -n
and -x
allow you to preview and monitor the formatting changes before applying them.