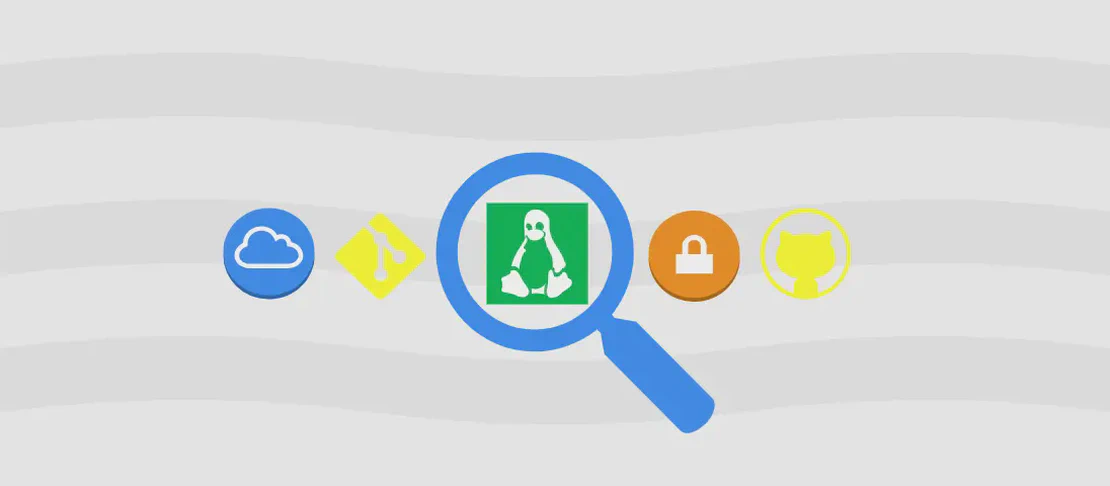
How to use the command "go-generate" (with examples)
In Go, the go generate
command is used to generate Go files by running commands within source files. This command is particularly useful in automating code generation or performing other tasks within the Go source files themselves. In this article, we will explore different use cases of the go generate
command and provide code examples for each case.
Use Case 1: Generate Go files by running commands within source files
The first and most common use case of the go generate
command is to generate Go files by running specific commands within the source files. This allows developers to automate the generation of code or perform other tasks using code annotations.
Code Example
Here’s an example of using go generate
to generate Go files:
//go:generate go run generate.go
In this example, we declare a code annotation using the //go:generate
comment. The comment is followed by the command we want to run, in this case, go run generate.go
. When we run go generate
in the command line, it will execute the specified command within the source file.
Motivation
Using go generate
for code generation can be particularly useful when we want to automate the creation of repetitive code or generate code based on specific criteria. It saves developers time and effort by automatically generating code that would otherwise need to be written manually.
Explanation
The //go:generate
comment is used to define the command that should be executed within the source file. It must be followed by the command itself, which can include any valid shell command or Go executable.
Example Output
When we run go generate
with the above code example, it will execute the go run generate.go
command within the source file. The output will depend on the specific command being executed and can vary for each use case.
Use Case 2: Customizing go generate
behavior with build constraints
The go generate
command can also be customized using build constraints. Build constraints are special comments that allow developers to control which commands are executed during the generation process based on certain conditions.
Code Example
Here’s an example of using build constraints with go generate
:
// +build generate
//go:generate go run generate.go
In this example, we first add a build constraint comment // +build generate
before the //go:generate
comment. This build constraint specifies that the go:generate
command should only be executed when the generate
build tag is present.
Motivation
Using build constraints with go generate
allows developers to customize the generation process based on different conditions. This can be useful when we want to generate different code based on the target platform, the build tags, or any other constraint defined in the build constraints comment.
Explanation
Build constraints are used to specify under which conditions the go:generate
command should be executed. They can be added as a comment before the go:generate
comment and consist of a build tag or a set of build tags.
Example Output
When running go generate
with the above code example, the go:generate
command will only be executed if the generate
build tag is specified during the build process. Otherwise, the command will be skipped.
Use Case 3: Customizing go generate
behavior with flags
In addition to build constraints, the go generate
command can also be customized further using flags. Flags allow developers to pass additional parameters to the go generate
command and modify its behavior based on the provided values.
Code Example
Here’s an example of using flags with go generate
:
//go:generate go run generate.go -packageName=myapp
In this example, we add the -packageName
flag to the go:generate
command, followed by the desired value (myapp
). The generate.go
program will then read this flag and use it for generating the appropriate code.
Motivation
Using flags with go generate
provides developers with flexibility in customizing the code generation process. It allows passing dynamic values as arguments to the go:generate
command, making it easy to generate code based on specific conditions or parameters.
Explanation
Flags can be added after the command in the go:generate
comment. They serve as parameters for the command being executed and can be accessed within the specified program or script. Flags can have different names and values, depending on the specific requirements of the code generation process.
Example Output
When running go generate
with the above code example, the generate.go
program will receive the -packageName
flag with the value myapp
. The program can then use this value to generate code specific to the provided package name.
Conclusion
The go generate
command is a powerful tool in Go that allows developers to automate code generation and perform various tasks within the source files. In this article, we explored different use cases of the go generate
command and provided code examples for each case. By leveraging go generate
, developers can save time and effort by automating repetitive tasks and customizing the code generation process.