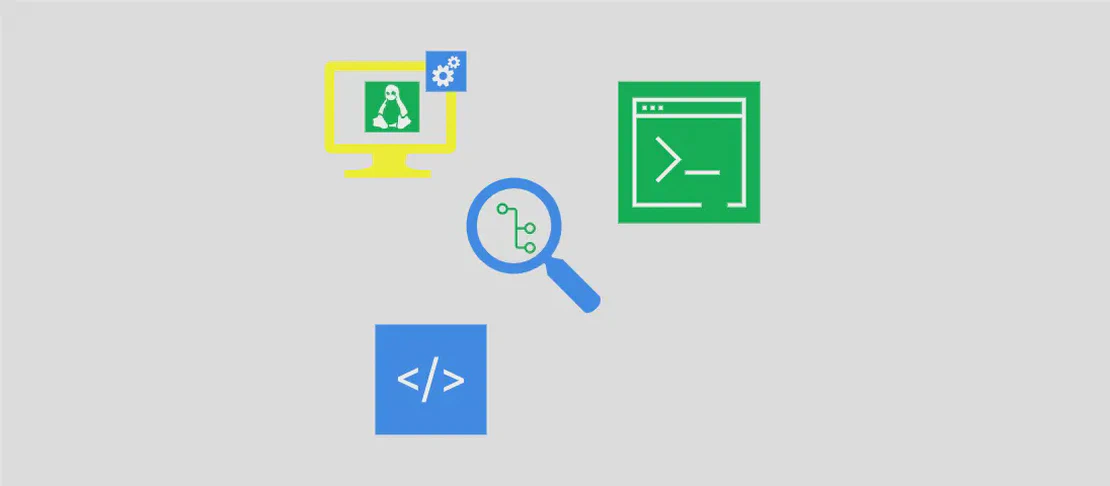
How to use the command 'go install' (with examples)
The go install
command is a powerful tool within the Go programming language ecosystem, primarily used for compiling and installing packages specified by their import paths. This command enables developers to build executable Go programs or reusable library packages and place them in a specific location, enabling easy access and execution. The go install
command plays a pivotal role in the Go language’s build and distribution process, streamlining the way packages are managed and utilized.
Use case 1: Compile and install the current package
Code:
go install
Motivation:
Using go install
without any additional path argument focuses on compiling and installing the current package in the directory you are working in. This is extremely useful for Go developers who are working on smaller projects or those parts of a large project that are separated into individual packages. By working within the directory of the respective package, a developer can conveniently compile it and make the updated executable or library accessible without additional parameters.
Explanation:
go install
: This command, when run without further arguments, instructs the Go toolchain to compile and install the package found within the current directory. The Go tool will look for amain
package, if the package is an application or compile the library if it’s a library package.
Example output:
After running the go install
command, you might not see an explicit output on the command line if the build process was successful. Instead, the executable (if it’s a main package) will be placed in your $GOBIN
directory, which is typically $GOPATH/bin
, or in the default Go bin directory.
Use case 2: Compile and install a specific local package
Code:
go install path/to/package
Motivation:
In scenarios where multiple packages exist and you need to work with or update a specific package that is not the one you are currently in, specifying the path to the package can be very efficient. This is especially helpful in large codebases that have a modular structure, allowing developers to focus and work on specific parts of the project without needing to navigate their directories.
Explanation:
go install
: This remains the core command that spearheads the build and installation process.path/to/package
: By specifying this relative or absolute path, you direct the Go tool to a particular package that you intend to compile and install. This path should point to the folder containing either themain
package or a library package inside your project or GOPATH.
Example output:
Like the previous use case, successful execution results in an updated executable in $GOBIN
. If there are errors, such as syntax errors or missing dependencies, those will be displayed in the command line interface.
Use case 3: Install the latest version of a program, ignoring go.mod
in the current directory
Code:
go install golang.org/x/tools/gopls@latest
Motivation:
In some cases, you may want to use a program that has been updated outside the constraints of your current project’s go.mod
file, which usually manages dependency versions. This command is useful when you wish to obtain the very latest version of a Go program from a module without altering the dependencies managed within your existing project’s setup.
Explanation:
go install
: Used to trigger the installation process.golang.org/x/tools/gopls
: This is the module path to the Go language server,gopls
, hosted on the public module repository.@latest
: By appending@latest
, you instruct Go to fetch and install the latest version of the specified module, irrespective of any version mentioned in yourgo.mod
file.
Example output:
Upon completion, the latest version of the program will be installed into your Go binary directory. If gopls
has updates or feature changes, they are immediately accessible after this command finishes execution. This command doesn’t alter your current go.mod
but updates the binary to the latest available version.
Use case 4: Install a program at the version selected by go.mod
in the current directory
Code:
go install golang.org/x/tools/gopls
Motivation:
Unlike the previous use case, this approach ensures that the version of the program installed aligns with the version constraints defined in the project’s go.mod
file. This is vital for maintaining consistency across development environments, particularly when collaborating in teams where reproducibility and environment parity are essential.
Explanation:
go install
: This functional element kicks off the process of building or installing the designated Go module.golang.org/x/tools/gopls
: Here, you specify the Go language server as before, but without a version tag, aligning it with the version specified in thego.mod
.
Example output:
Executing this command via the terminal will install the version of gopls
indicated in the current project’s go.mod
file. If go.mod
specifies a specific version through dependency management, the installed version will match accordingly. Errors pertaining to version fetching and compatibility are shown if issues arise.
Conclusion:
The go install
command in Go is a flexible and essential tool that aids developers in efficiently compiling and installing libraries and programs. Through its various use cases, it offers a range of capabilities, from installing the latest versions of external tools to ensuring project-specific version alignment, thus enabling streamlined development workflows.