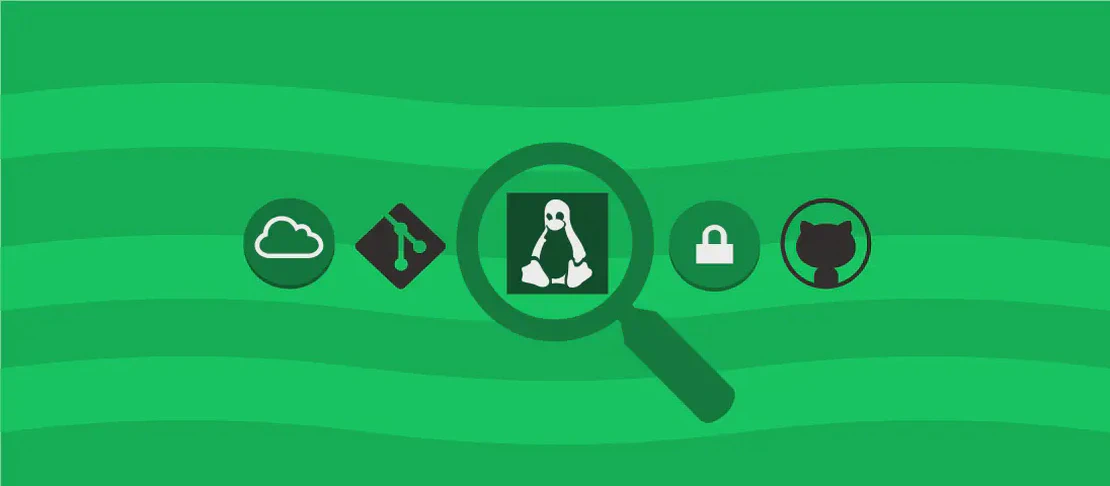
Exploring the Uses of the `go list` Command (with examples)
The go list
command is an integral part of the Go programming language ecosystem that provides developers with the ability to list and inspect packages and modules. This command is useful for gathering information about Go codebases such as identifying package dependencies, discovering standard library packages, and updating module information. By using go list
, developers can easily maintain and explore their Go projects.
Use case 1: List Packages
Code:
go list ./...
Motivation:
Suppose you are working on a relatively large Go project, and you need to compile a list of all the local packages within the project. This task is essential for gaining insights into the project’s structure, locating where certain functionalities are implemented, or conducting mass package updates. The go list ./...
command will help you achieve this efficiently by recursively listing all Go packages within the current directory and its subdirectories.
Explanation:
go
: This is the Go command line tool that helps execute commands related to the Go language.list
: This subcommand tells Go to list packages or modules../...
: The./
refers to the current directory, and the ellipsis...
is a wildcard that extends the search to all subdirectories recursively, ensuring all related packages are included in the output.
Example Output:
example.com/project/package1
example.com/project/package2
example.com/project/subpackage/package3
...
Use case 2: List Standard Packages
Code:
go list std
Motivation:
When developing Go applications, it is often necessary to know which packages are available in the standard library. This knowledge can prevent developers from importing third-party packages unnecessarily when equivalent functionality exists in Go’s standard library. By using the go list std
command, you can get a comprehensive list of all standard packages, aiding in efficient library usage and reducing external dependencies.
Explanation:
std
: This argument specifies that the list command should include only standard library packages rather than third-party or local project packages.
Example Output:
archive/tar
archive/zip
bufio
...
Use case 3: List Packages in JSON Format
Code:
go list -json time net/http
Motivation:
JSON output is highly structured and easily machine-readable, making it beneficial when integrating with tools or scripts that process package information. When needing to programmatically analyze the details of specific packages such as time
and net/http
, the go list -json
command provides a comprehensive JSON representation of these packages, including metadata like import paths, compiled binaries, and source files.
Explanation:
-json
: This flag indicates that the output should be formatted in JSON, providing detailed and structured information.time net/http
: These are the specific packages being queried, showcasing how to get details for multiple packages at once.
Example Output:
{
"ImportPath": "time",
"Name": "time",
...
}
{
"ImportPath": "net/http",
"Name": "http",
...
}
Use case 4: List Module Dependencies and Available Updates
Code:
go list -m -u all
Motivation:
One of the critical aspects of maintaining a Go module is ensuring that all dependencies are up to date. With frequent updates and improvements to libraries, keeping them up to date is vital for security and performance enhancements. By using go list -m -u all
, developers can view all dependencies of their project and check if newer versions are available. This command facilitates informed decision-making on whether to upgrade packages.
Explanation:
-m
: This flag specifies that the command should consider modules instead of individual packages.-u
: Indicates that the list should include information about module versions and whether any newer versions are available.all
: Lists all modules involved in the current module, ensuring comprehensive coverage of dependencies.
Example Output:
example.com/project/module v1.0.0 => v1.0.1
github.com/some/dependency v0.9.0 => v0.10.0
golang.org/x/net v0.0.0-20210921155107-c860ab60c48
Conclusion:
The go list
command is a versatile and powerful tool for Go developers, providing vital information about packages and modules. Whether you need to understand the structure of a project, explore standard library offerings, extract detailed JSON insights, or manage and update dependencies, go list
proves indispensable. Mastery of this command aids in the effective and efficient management of Go projects, enhancing both development and maintenance workflows.