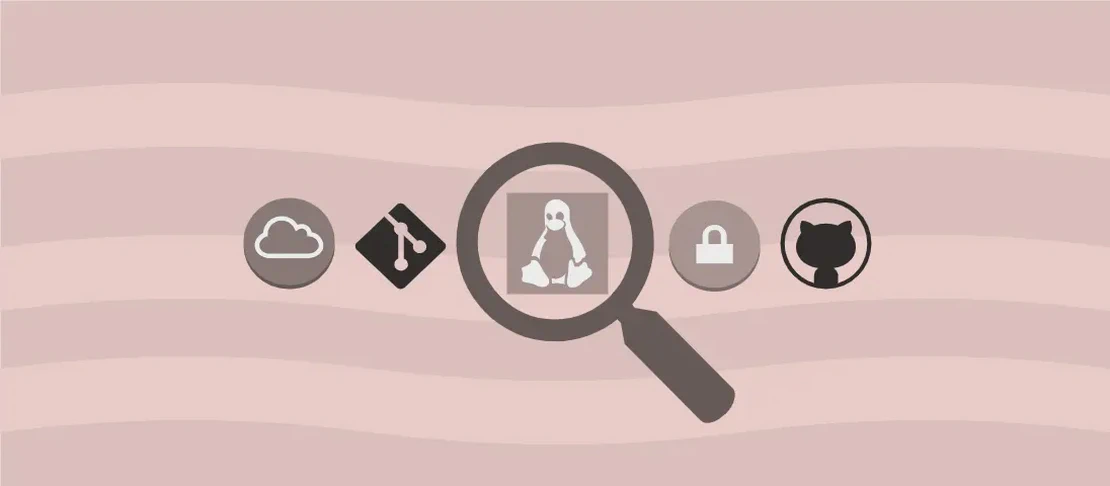
How to Use the Command 'go mod' (with Examples)
The go mod
command is an essential tool in the Go programming language ecosystem. It provides developers with a set of functions to manage Go modules, which are collections of related Go packages. Introduced in Go 1.11, modules are the recommended way to manage dependencies in a Go project, replacing the older GOPATH-based dependency management. The go mod
command helps developers to initialize, maintain, verify, and manage dependencies and modules to streamline the development process.
Use Case 1: Initialize New Module in Current Directory
Code:
go mod init moduleName
Motivation:
When starting a new Go project, it’s crucial to define a module to organize and manage the project’s dependencies effectively. By initializing a module, you create a go.mod
file, which acts as the manifest for your project. This file registers the module and specifies its dependencies, versions, and requirements. This setup helps in maintaining consistent builds and eases collaboration among team members.
Explanation:
init
: This subcommand initializes a new module by creating ago.mod
file in the current directory.moduleName
: This is a placeholder for the actual name you’d like to assign to your module. ThemoduleName
is typically the module’s import path, often derived from a version control system or a repository URL likegithub.com/user/repo
.
Example Output:
go: creating new go.mod: module moduleName
Use Case 2: Download Modules to Local Cache
Code:
go mod download
Motivation:
Downloading modules into a local cache ensures that all required modules and their dependencies are available offline and ready for compilation. This is particularly useful for continuous integration/continuous deployment (CI/CD) environments or securing earlier preparation of dependencies before building the module.
Explanation:
download
: This subcommand downloads the modules specified in yourgo.mod
file, storing them in Go’s module cache, usually located within your user directory. It fetches not only the selected versions of direct dependencies but also their transitive dependencies.
Example Output:
go: downloading module github.com/example/dependency v1.2.3
go: downloading module github.com/example/other-dependency v0.5.0
Use Case 3: Add Missing and Remove Unused Modules
Code:
go mod tidy
Motivation:
Over time, as a project evolves, certain dependencies may become obsolete, or new ones might be mistakenly missing from the module file. This command is used to clean up the go.mod
and go.sum
files by automatically identifying and downloading missing dependencies and removing unused ones. This helps in keeping your module manifest efficient and decluttered.
Explanation:
tidy
: This subcommand rechecks the code to determine which modules are necessary, adds missing module requirements and sums, and cleans up unused code dependencies. It’s a practical step for housekeeping in a Go project.
Example Output:
go: finding module for package github.com/new/dependency
go: found github.com/new/dependency in github.com/new/dependency v1.0.0
go: removing unused module github.com/old/dependency v0.1.0
Use Case 4: Verify Dependencies Have Expected Content
Code:
go mod verify
Motivation:
Verifying module dependencies ensures the integrity and consistency of your code base. It confirms that the module’s contents match the expected cryptographic checksums listed in the go.sum
file. This is an essential step to mitigate issues with unexpected changes in dependencies that could otherwise lead to compilation errors or security vulnerabilities.
Explanation:
verify
: This subcommand checks each module to ensure that the content downloaded to the local module cache matches the expected checksums. It compares the modules in thego.sum
file with the cached ones to detect potential discrepancies.
Example Output:
all modules verified
Use Case 5: Copy Sources of All Dependencies into the Vendor Directory
Code:
go mod vendor
Motivation:
Using a vendor directory can be advantageous for teams working in environments with restricted internet access or needing to lock all dependencies in a singular location within the project. It provides a way to bundle dependent module source code directly into your project’s workspace, ensuring build consistency without relying on external sources.
Explanation:
vendor
: This subcommand copies all the modules specified by thego.mod
file into a directory namedvendor
within the project’s root. This operation supports traditional vendoring and allows dependency resolution without access to the module cache.
Example Output:
go: creating vendor/modules.txt
go: copying dependencies to vendor directory
Conclusion:
The go mod
command offers a powerful suite of functionalities for effectively managing Go project modules. Each subcommand targets specific needs, from initializing new projects and importing dependencies to ensuring dependency integrity and facilitating vendor management. This practice streamlines Go projects, making them easier to maintain and more robust in collaborative and production environments. By leveraging these go mod
utilities, Go developers can ensure smooth project workflows and enhance the overall reliability of their software applications.