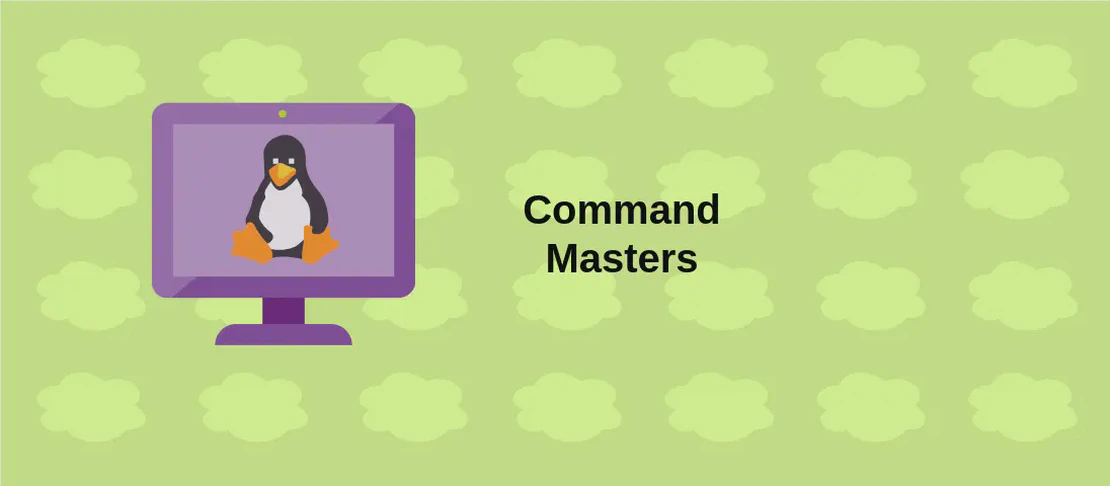
How to use the command 'go test' (with examples)
The go test
command is a powerful tool in the Go programming language suite that is used to execute tests written for Go packages. These tests are essential for validating that your code behaves as expected and is free of defects. By specifying arguments and flags, you can tailor the testing process to suit your requirements, ranging from basic function checks to complex performance analysis with benchmarks. This command supports testing code files that must end with _test.go
, enabling a structured way to maintain and run your test suites effectively.
Use case 1: Test the package found in the current directory
Code:
go test
Motivation for using the example:
This command is the simplest form of invoking tests for the current package. It’s ideal when you want a quick verification of your changes to ensure that existing functionality isn’t broken. Imagine you’ve just debugged a small issue or added a new function; this command helps confirm that all tests in the current package still pass.
Explanation for every argument given in the command:
go
: This is the main Go command which provides access to various Go tools such as building, running, and testing Go code.test
: The subcommand under Go that’s specifically used to run tests.
Example output:
ok my/package 0.123s
Use case 2: Verbosely test the package in the current directory
Code:
go test -v
Motivation for using the example:
Sometimes, a simple success or failure message isn’t enough. You might need detailed output on which tests were run and their respective statuses. This verbosity can be essential when debugging test failures as it provides more context and insight into the execution flow.
Explanation for every argument given in the command:
-v
: A flag to increase verbosity, printing the names of tests, their results, and any log output from within the tests.
Example output:
=== RUN TestFunction1
--- PASS: TestFunction1 (0.00s)
=== RUN TestFunction2
--- PASS: TestFunction2 (0.01s)
ok my/package 0.123s
Use case 3: Test the packages in the current directory and all subdirectories
Code:
go test -v ./...
Motivation for using the example:
Software projects are often composed of multiple packages. Testing just the current directory may not cover changes affecting submodules or components. By using ./...
, you can recursively test all packages within the directory structure, ensuring thorough integration testing across your entire project.
Explanation for every argument given in the command:
./...
: Specifies that the tests should run for all directories and subdirectories from the current path.
Example output:
=== RUN ExampleSubPackage
--- PASS: ExampleSubPackage (0.02s)
ok my/package/subpackage 0.123s
ok my/package 1.456s
Use case 4: Test the package in the current directory and run all benchmarks
Code:
go test -v -bench .
Motivation for using the example:
Benchmarking is crucial when performance considerations are integral to your application, like in high-frequency trading systems or large data processing. This example not only runs your tests but also your benchmarks, allowing you to track execution times for performance-critical sections of your code.
Explanation for every argument given in the command:
-bench .
: Executes benchmark tests in addition to the regular tests, where.
matches any benchmark in the current package.
Example output:
PASS
BenchmarkFunctionX 5000000 350 ns/op
ok my/package 3.456s
Use case 5: Test the package in the current directory and run all benchmarks for 50 seconds
Code:
go test -v -bench . -benchtime 50s
Motivation for using the example:
When you need a more comprehensive performance analysis, running benchmarks over an extended period can provide reliable insights by overshadowing short-lived fluctuations. Use this command for detecting even subtle differences in your system’s performance over an extended duration.
Explanation for every argument given in the command:
-benchtime 50s
: Directs the testing framework to run benchmarks continuously for 50 seconds to enhance result accuracy and reliability.
Example output:
PASS
BenchmarkFunctionY 10000000 250 ns/op
ok my/package 58.789s
Use case 6: Test the package with coverage analysis
Code:
go test -cover
Motivation for using the example:
Code coverage indicates how much of your code is being tested, thus identifying untested parts of your application. This command is vital for ensuring that significant areas of your codebase are exercised by tests, improving the reliability and robustness of the application.
Explanation for every argument given in the command:
-cover
: Generates a coverage report that specifies what percentage of the package code is actually covered by the tests.
Example output:
ok my/package 0.123s coverage: 85.2% of statements
Conclusion:
The go test
command is an indispensable utility for developers working within the Go language, ensuring code reliability and performance. By exploring these various use cases, developers can utilize go test
not only to validate functional correctness but also to enhance performance through benchmarks and coverage analysis. Each command has specific benefits that cater to different aspects of the development and testing lifecycle, making it a versatile and indispensable part of Go software development.