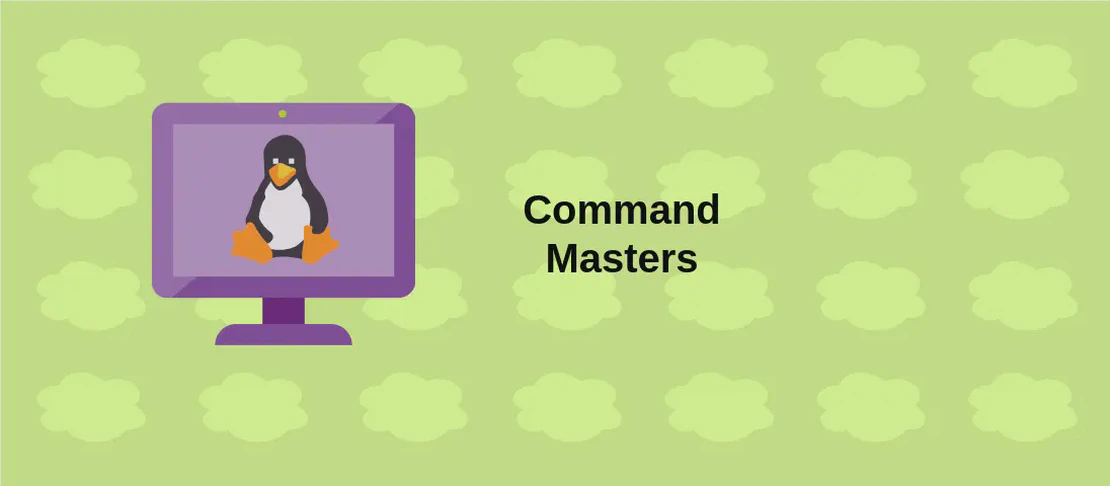
How to Use the Command 'go tool' (with examples)
The go tool
command is an integral part of the Go programming language ecosystem, providing developers with access to a variety of tools that help in development, debugging, and cross-compilation. The command allows users to execute a specific Go tool or command as a stand-alone binary. Each tool housed under go tool
serves a unique purpose, ranging from linking binaries to viewing cross-compilation targets.
Use case 1: List available tools
Code:
go tool
Motivation:
When working with the Go programming language, it’s often essential to understand the various tools at your disposal that can help optimize your workflow or solve specific problems. Listing the available tools helps developers quickly identify the tools they may want to explore further or that could be useful for a current task. This command is especially useful for those new to Go or those who have upgraded their Go installation and want to see what new tools are available.
Explanation:
The go tool
command when run without any additional arguments, outputs a list of all the tools available in the current Go installation. This includes core tools maintained as part of the Go distribution, like link
, vet
, and others.
Example Output:
addr2line
cgo
compile
cover
dist
link
nm
objdump
pack
pprof
trace
vet
Use case 2: Run the go link tool
Code:
go tool link path/to/main.o
Motivation:
Linking is a critical step in combining compiled code (object files) into an executable binary. The link
tool provided by go tool
helps developers in the final step of building Go applications. This example shows how to run the Go linker, which will take object files generated from compiled Go source code and produce a runnable binary. This is crucial after the compilation of a Go program when one needs to deploy or execute the application.
Explanation:
link
: Specifies the tool to be used, in this case, the Go linker.path/to/main.o
: The path to the object file that you want to link. Object files are typically generated by the compiler.
Assuming main.o
is in a typical directory setup, this would produce an executable based on the object files you provide.
Example output:
Linking...
Executable created: ./main
Use case 3: Print the command that would be executed, but do not execute it
Code:
go tool -n command arguments
Motivation:
This use case is particularly beneficial when you want to see the exact system-level command that would be executed by a specific go tool
invocation. This is helpful for debugging, learning purposes, or when you want to get insight into what parameters or flags are being passed at a lower level without actually running the command. It provides a dry-run capability similar to using whereis
or a verbose mode in other command-line tools.
Explanation:
-n
: This flag tells thego tool
to print the command it would have executed rather than executing it. It effectively acts as a dry run.command
: The specific tool or command you wish to inspect.arguments
: Placeholder for any arguments you might pass to the command.
Example output:
/usr/local/go/pkg/tool/linux_amd64/compile -o ./main.o main.go
Use case 4: View documentation for a specified tool
Code:
go tool command --help
Motivation:
Having easy access to documentation is invaluable when using command-line tools. Viewing the help documentation for a specific Go tool provides users with information about the available flags, usage examples, and potential arguments that can be used with that tool. This is crucial for effective utilization of the tools, especially for more complex commands where various options can have substantial effects.
Explanation:
command
: The specific Go tool command for which you want to view documentation.--help
: A common flag that prints out the help information for the command, describing its usage and options.
Example output (for go vet
):
Usage of vet:
-printf
Check printf-style functions
-shadow
Check for possible unintended shadowing (experimental; see doc)
...
Use case 5: List all available cross-compilation targets
Code:
go tool dist list
Motivation:
Cross-compiling allows Go applications to be built for different operating systems and architectures from a single codebase and build environment. Listing all available cross-compilation targets gives developers a clear indication of the platforms they can target directly from their current setup. This is particularly useful when developing applications that need to be deployed across diverse environments.
Explanation:
dist
: Represents the Go distribution tool used for managing installations and environments.list
: A subcommand that allows you to see all available targets for cross-compilation.
Example output:
android/386
android/amd64
android/arm
android/arm64
darwin/amd64
darwin/arm64
...
Conclusion:
The go tool
command is a versatile and powerful utility necessary for any Go developer’s toolkit. Whether you’re linking compiled files, exploring available tools, or listing cross-compilation targets, understanding how to leverage this command effectively can greatly enhance both your development efficiency and the versatility of your Go applications.