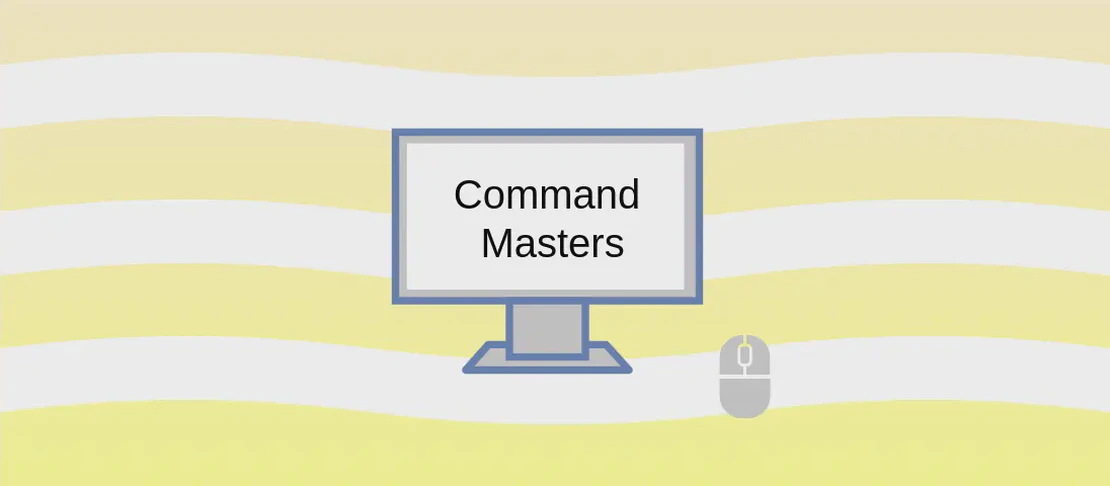
How to Use the Command 'go vet' (with Examples)
The go vet
command is a crucial tool for Go developers, designed to check Go source code and report any suspicious constructs. It effectively acts as a linting tool, pinpointing potential issues that could lead to bugs or unexpected behavior in your code. If any problems are found, go vet
returns a non-zero exit code, and conversely, a zero exit code if no problems are detected. This tool enhances code quality by ensuring that source files adhere to Go’s best practices and conventions.
Use case 1: Check the Go package in the current directory
Code:
go vet
Motivation:
Running go vet
without any additional arguments checks the Go package located in the current directory. This is particularly useful for developers who want to ensure that the code they are actively working on is free of subtle errors before committing or deploying it. By routinely using go vet
in the development process, programmers can efficiently identify and correct issues early, enhancing the stability and reliability of the application.
Explanation:
go vet
: By executing this command, you initiate a comprehensive scan of all Go source files in the current working directory. The tool will perform various checks aimed at identifying coding errors, such as incorrect print formatting, or usage of built-in functions that might lead to runtime failures.
Example output:
# command-line-arguments
./main.go:10:2: assignment count mismatch: 2 = 3;
./util.go:5:6: exported function Foo should have comment or be unexported
Use case 2: Check the Go package in the specified path
Code:
go vet path/to/file_or_directory
Motivation:
Specifying a path allows developers to target a specific package or directory for vetting, which is beneficial when working with larger codebases. This focused approach means you can vet sections of the project independently, streamlining the review process especially in multi-package projects where different teams or developers work on separate modules.
Explanation:
path/to/file_or_directory
: By specifying this argument, you directgo vet
to analyze a particular file or directory instead of the default current directory. This offers flexibility, letting you focus the analysis on parts of code that have recently changed or are under active development.
Example output:
# example/package/path
example/package/path/foo.go:23:15: Printf format %d reads arg #1, but call has only 0 args
Use case 3: List available checks that can be run with go vet
Code:
go tool vet help
Motivation:
Understanding the specifics of what go vet
checks can be extremely useful for developers aiming to apply specific tests or to expand the range of checks performed. Knowing all available options ensures that developers can leverage the full capabilities of go vet
, tailoring tests to suit the requirements of different projects or coding standards.
Explanation:
go tool vet help
: This command provides a detailed list of all available checks thatgo vet
can perform. By acquainting yourself with these options, you can make informed decisions about which exact checks are most pertinent to your project’s needs.
Example output:
usage: vet [flags] [directory | files.go ...]
Composite literals checks for unkeyed composite literals (composites).
...
Use case 4: View details and flags for a particular check
Code:
go tool vet help check_name
Motivation:
When a specific issue is identified by go vet
, developers may wish to delve deeper into the particular check responsible for reporting the warning or error. This approach allows developers to understand the rationale and mechanism of specific checks, facilitating better compliance with intended coding guidelines and informing more effective code correction strategies.
Explanation:
check_name
: Replace this with the name of the specific check you are interested in. The command will yield detailed information about that particular test including its purpose, how it operates, and any flags you can use to customize its operation.
Example output:
The printf check validates the format arguments of Printf-like functions. It checks for mismatches between format strings and arguments.
Use case 5: Display offending lines plus N lines of surrounding context
Code:
go vet -c=N
Motivation:
When working with large files, pinpointing exactly where an error occurs can be challenging. By including a few lines of context around the offending line, developers can gain better insights into the surrounding code, thus making it easier to comprehend the scope of the issue and how it might interact with other parts of the code.
Explanation:
-c=N
: The-c
flag is followed by a numerical valueN
, indicating how many lines of context should be displayed around the offending lines. This helps in understanding the issue in the context of surrounding code, contributing to a more informed and effective debugging process.
Example output:
main.go:8:2: for loop must specify range
6 for i : 1 to 3 {
7 fmt.Println(i)
> 8 }
main.go:10:1: print format %d mismatched with argument string
Use case 6: Output analysis and errors in JSON format
Code:
go vet -json
Motivation:
Outputting results in JSON format can be incredibly useful for integrating go vet
into automated systems or for developers who prefer to parse and process output using scripts or other tools. JSON’s structured and standardized nature makes it ideal for automation, allowing easy extraction and analysis of information.
Explanation:
-json
: This flag instructsgo vet
to present its output in JSON format. The structured data format is particularly advantageous for developers looking to incorporatego vet
into a larger toolchain or CI/CD pipeline, facilitating smoother integration and better data handling.
Example output:
[
{
"file": "main.go",
"line": 10,
"column": 2,
"message": "assignment count mismatch: 2 = 3",
"severity": "error"
}
]
Conclusion:
The go vet
command is an invaluable part of the Go developer’s toolkit, providing a robust set of features for identifying potential issues in Go code. By using go vet
as part of a regular development and pre-deployment workflow, teams can significantly improve code quality, reduce bugs, and ensure adherence to best practices. The examples provided illustrate various ways to leverage go vet
for optimal code review and maintenance, aiding developers in writing clean, efficient, and secure Go programs.