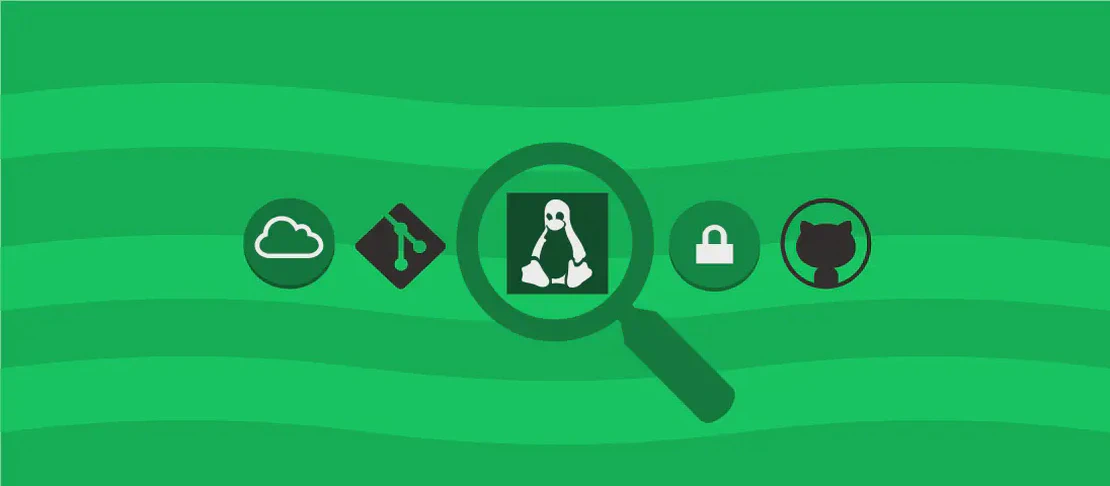
Managing Golang Versions with goenv (with examples)
The goenv
tool is a command-line utility designed to simplify the management of different versions of the Go programming language, commonly known as Golang. This tool streamlines the process of installing, uninstalling, and switching between multiple Go versions, making it invaluable for developers working on projects that require varied Go environments. Hosted on GitHub, users can access detailed instructions and further information to enhance their use of goenv
by visiting: GoEnv GitHub
.
Use case 1: List all available goenv commands
Code:
goenv commands
Motivation:
This use case allows developers to familiarize themselves with the comprehensive functionality that goenv
offers. By listing all available commands, users can understand the capabilities and options at their disposal, potentially discovering features that they were previously unaware of.
Explanation:
goenv
: This is the base command for interacting with the Go version manager.commands
: This argument requests a list of all commands available withingoenv
, serving as a helpful guide for users.
Example Output:
Available goenv commands:
install Install a specific version of Go
uninstall Uninstall a specific version of Go
local Set a specific Go version for the current project
global Set the global Go version
versions List all installed Go versions
exec Run commands with a specific Go version
Use case 2: Install a specific version of Golang
Code:
goenv install 1.16.15
Motivation:
At times, a project may require a specific version of Golang, either due to dependency compatibility or testing needs. Using goenv install
, developers can effortlessly install any necessary Golang version, ensuring their development environment matches project requirements.
Explanation:
goenv
: The command initiates the Go version manager.install
: This argument specifies the intention to install a new version of Go.1.16.15
: Represents the version of Golang that the user wishes to install.
Example Output:
Downloading Go 1.16.15...
Installing Go 1.16.15...
Installation complete.
Use case 3: Use a specific version of Golang in the current project
Code:
goenv local 1.16.15
Motivation:
Different projects may depend on different versions of Golang. The goenv local
command enables developers to set a specific Go version for a particular project directory, ensuring that any Go-related tasks in that project utilize the correct version.
Explanation:
goenv
: Invokes the Go version manager.local
: Defines the Go version to be used in the local context or current project directory.1.16.15
: This is the version of Go that will be set for the current project.
Example Output:
Local Go version set to 1.16.15.
Use case 4: Set the default Golang version
Code:
goenv global 1.16.15
Motivation:
Consistency in development environments is crucial; setting a global default version of Golang using goenv
ensures that all projects default to a particular Go version unless specifically overridden. This sets a stable foundation for Go development across new projects.
Explanation:
goenv
: Utilizes the Go version manager.global
: This command sets the global Go version, which acts as the default for all operations unless a local version is specified.1.16.15
: Indicating the Go version to be used globally across all projects.
Example Output:
Global Go version set to 1.16.15.
Use case 5: List all available Golang versions and highlight the default one
Code:
goenv versions
Motivation:
For efficient version management, developers must be aware of all Go versions installed on their system. This use case shows how goenv
can list all available versions and identify which version is set as default, aiding in version tracking and project planning.
Explanation:
goenv
: The base command for Go version management.versions
: This argument requests a list of all installed Go versions, highlighting the default version.
Example Output:
1.15.12
1.16.15
* 1.16.15 (set by /path/to/.goenv/version)
1.18.10
Use case 6: Uninstall a given Go version
Code:
goenv uninstall 1.16.15
Motivation:
As projects evolve, certain versions of Go may become obsolete. Using goenv uninstall
, developers can efficiently remove outdated Go versions, decluttering their system and freeing up resources while maintaining a tidy development environment.
Explanation:
goenv
: Activates the Go version management tool.uninstall
: Specifies that a Go version will be removed from the system.1.16.15
: Denotes the version of Go to be uninstalled.
Example Output:
Uninstalling Go 1.16.15...
Go 1.16.15 successfully uninstalled.
Use case 7: Run an executable with the selected Go version
Code:
goenv exec 1.16.15 go run main.go
Motivation:
To test an application across multiple Go versions, developers may need to execute code with a specific Go version. This use case demonstrates how goenv exec
allows developers to run an executable using any installed Go version without altering the globally set version.
Explanation:
goenv
: Triggers the Go environment manager.exec
: Indicates that an executable command should be run with a specified Go version.1.16.15
: Specifies the Go version to use for executing the command.go run main.go
: The executable command to run, which starts execution of the Go program located inmain.go
.
Example Output:
Running Go 1.16.15: Starting main...
Output from the Go application
Conclusion
The goenv
tool is an indispensable utility for developers working with multiple versions of Golang. By streamlining version management, goenv
ensures that developers can easily install, uninstall, and switch between different Go environments. Whether setting a global default or specifying a local project version, goenv
facilitates a more organized and efficient development process.