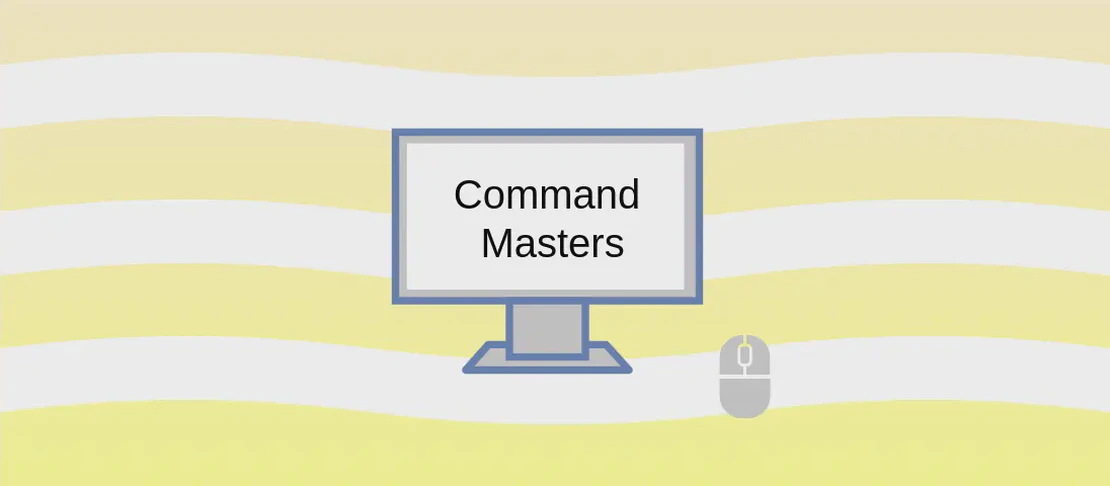
Mastering gofmt for Go Code Formatting (with Examples)
Gofmt is a valuable tool for Go developers that facilitates automatic formatting of Go source code. It is widely used in the Go community to ensure that code is stylistically consistent and adheres to best practices. The command can reformat and simplify code, making it easier to read, understand, and maintain. This article will demonstrate several use cases for the gofmt
command, providing examples and explanations for each.
Use Case 1: Format a File and Display the Result to the Console
Code:
gofmt source.go
Motivation: When working on Go projects, developers often need to quickly check the formatting of their code without making permanent changes. Displaying the changes in the console allows developers to review the adjustments that gofmt
would apply to ensure consistent and readable code formatting.
Explanation:
gofmt
is the command invoked to format the Go source code.source.go
is the name of the file to be formatted. This file contains the Go code you wish to check for necessary formatting changes. The command reads the input file, applies the necessary formatting rules, and outputs the formatted version to the standard output, typically the terminal.
Example Output: Assuming source.go
contains Go code with irregular spacing and misaligned brackets, using gofmt
will display a version of the code with corrected indentation and alignment.
package main
import (
"fmt"
)
func main() {
fmt.Println("Hello, World!")
}
Use Case 2: Format a File, Overwriting the Original File In-Place
Code:
gofmt -w source.go
Motivation: Developers often choose to format their source files directly to save time and ensure consistency. This command is particularly useful for teams adhering to strict style guides, as it standardizes the format of the codebase automatically.
Explanation:
gofmt -w
extends the basicgofmt
functionality.-w
stands for “write,” and it tellsgofmt
to overwrite the original file with the formatted content.source.go
is the target file that will be directly modified to include the formatting changes.
Example Output: After executing the command, source.go
is rewritten with consistent spacing and indentation. Opening source.go
again will reveal that it now follows Go’s formatting rules.
Use Case 3: Format a File, and Then Simplify the Code, Overwriting the Original File
Code:
gofmt -s -w source.go
Motivation: Beyond basic formatting, simplifying the code can further enhance readability by removing clutter or redundant constructs. By also applying simplifications, developers can refactor their code for improved clarity and performance in one step.
Explanation:
gofmt -s -w
involves two flags:-s
and-w
.-s
stands for “simplify,” and it instructsgofmt
to perform code simplifications that adhere to idiomatic Go practices.-w
ensures that the changes made by both formatting and simplification are saved directly tosource.go
.
Example Output: The original content of source.go
not only reflects formatting improvements but also includes simplified expressions. For instance, redundant type conversions might be removed, leading to cleaner code.
Use Case 4: Print All (Including Spurious) Errors
Code:
gofmt -e source.go
Motivation: During development, encountering errors in code is inevitable. Displaying all errors, including non-essential ones, can assist developers in pinpointing areas that require attention, potentially highlighting issues that would otherwise be overlooked.
Explanation:
gofmt -e
incorporates the-e
flag alongside the maingofmt
command.-e
stands for “errors,” enabling the detailed display of all errors, including minor or non-critical ones, in the formatting ofsource.go
.
Example Output: Running this command might produce output that highlights errors such as misplaced brackets, incorrect indentation, or other common formatting mistakes. Although these are usually stylistic, addressing them can lead to more maintainable code.
-- source.go --
Line 3, Syntax error (missing semicolon)
Conclusion:
The gofmt
command is an essential utility for any Go developer focused on maintaining clean, readable, and idiomatically correct code. By automatically formatting Go files, simplifying code, and identifying potential errors, gofmt
enhances productivity and ensures compliance with Go’s conventions. These examples demonstrate how gofmt
can be leveraged to streamline Go development processes, enforce style consistency, and ultimately contribute to a more efficient and collaborative coding environment.