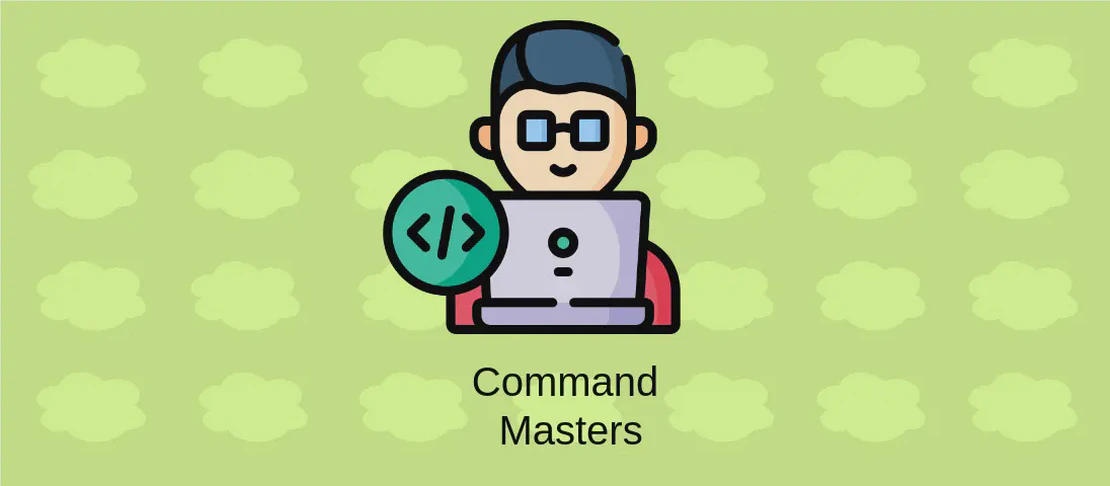
How to Use the Command 'goimports' (with examples)
The goimports
command is a powerful tool for Go developers that simplifies the management of import statements in Go source files. By automatically adding missing import statements and removing unreferenced ones, goimports
helps maintain a clean and efficient codebase. This tool is especially useful in large projects where managing imports manually becomes cumbersome. Here are some use cases that illustrate how goimports
can be employed effectively.
Use case 1: Display the Completed Import Source File
Code:
goimports path/to/file.go
Motivation for using this example:
When working on a Go project, it is common to import various packages that are used throughout the code. However, keeping track of all the necessary imports can be tedious, especially when refactoring or adding new code. Running goimports
allows developers to quickly verify the current state of imports and see which ones are required for the code to compile successfully. This is useful for initial checks and reviews to ensure that no unnecessary imports are left in the file.
Explanation for each argument:
path/to/file.go
: This is the path to the Go source file you want to process.goimports
will analyze this file to determine which imports are needed.
Example output:
When running this command, the terminal will display the contents of file.go
with the updated import statements. The unneeded imports will be removed, and missing ones will be added automatically.
package main
import (
"fmt"
"net/http"
)
func main() {
fmt.Println("Hello, World!")
http.ListenAndServe(":8080", nil)
}
Use case 2: Write the Result Back to the Source File Instead of stdout
Code:
goimports -w path/to/file.go
Motivation for using this example:
This use case is practical for developers who want to directly update their source files without manually copying the output from the terminal. By writing the results back to the file, developers can ensure that their code is always up to date with the correct import statements, thus reducing the overhead of manual edits and the risk of leaving outdated imports.
Explanation for each argument:
-w
: This flag tellsgoimports
to write the modified import statements back into the source file instead of outputting them tostdout
.path/to/file.go
: Specifies the Go file that needs import adjustments.
Example output:
Instead of displaying the changes, this command edits file.go
directly. The file is updated to reflect the necessary imports without producing any visible output.
Use case 3: Display Diffs and Write the Result Back to the Source File
Code:
goimports -w -d path/to/file.go
Motivation for using this example:
When making changes to a codebase, it’s often important to review what modifications have been applied. By displaying the diffs, developers can easily see which imports were added, removed, or rearranged, ensuring transparency and understanding of the changes occurring in the code. This use case is highly beneficial during code reviews and when working collaboratively with other developers.
Explanation for each argument:
-w
: Writes the changes to the original source file.-d
: Displays the differences between the current file and the updated content as a diff.path/to/file.go
: Indicates the file thatgoimports
will process.
Example output:
The terminal will show a diff of changes, highlighting additions and deletions to your import statements. The original file will also be updated.
diff -u old/path/to/file.go new/path/to/file.go
--- old/path/to/file.go
+++ new/path/to/file.go
@@ -1,5 +1,6 @@
package main
+import "fmt"
import "net/http"
func main() {
Use case 4: Set the Import Prefix String After 3rd-party Packages
Code:
goimports -local path/to/package1,path/to/package2,... path/to/file.go
Motivation for using this example:
In a large codebase, especially one that relies on many third-party packages, it’s helpful to organize imports into sections. By specifying local import prefixes, goimports
ensures that related packages are grouped together, following a consistent import order. This enhances readability and helps quickly identify where different dependencies come from.
Explanation for each argument:
-local
: A flag that specifies comma-separated import path prefixes which, when used, keep local imports grouped together in a distinct section within the import statement.path/to/package1,path/to/package2,...
: These are the prefixes for your local packages, helpinggoimports
understand how to group imports.path/to/file.go
: The Go file that will have its imports adjusted according to the specified prefixes.
Example output:
This command organizes your import statements based on the local package prefixes, ensuring your imports are structured for clarity.
package main
import (
"net/http"
"path/to/package1"
"path/to/package2"
)
Conclusion:
goimports
is an invaluable tool for Go developers who want to maintain clean and well-organized codebases. By automating the management of import statements, it not only increases productivity but also helps in maintaining consistency across the project. Whether you’re displaying, writing changes to files, or organizing imports with local prefixes, goimports
provides the flexibility needed for comprehensive import management.