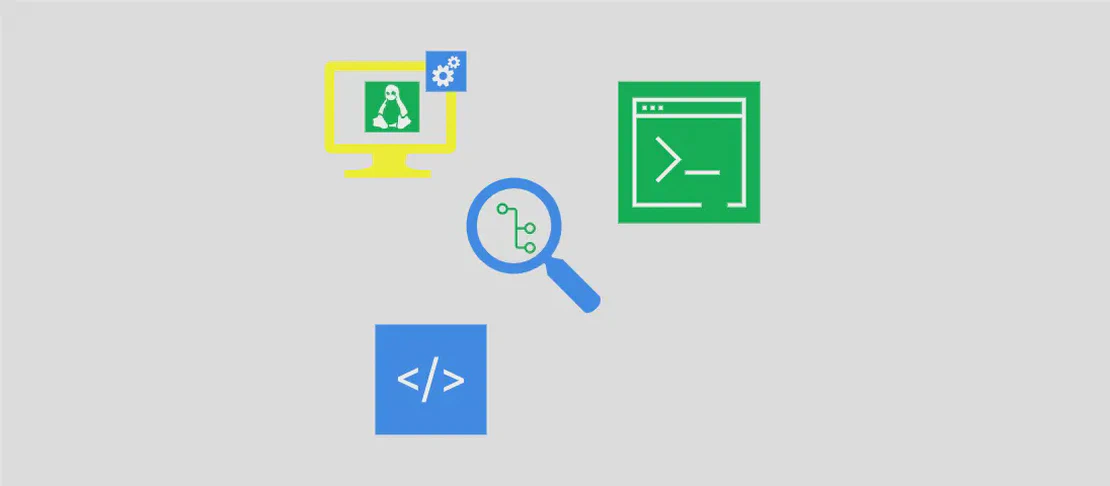
How to Use the Command 'golangci-lint' (with Examples)
Golangci-lint is a command-line tool designed to streamline the process of running multiple linters on Go (Golang) code. It operates in a parallelized manner to ensure quick and efficient analysis, making it an invaluable tool in the software development process. Additionally, it integrates seamlessly with all major Integrated Development Environments (IDEs) and allows for extensive customization through YAML configuration files. This tool aids developers in maintaining high code quality by identifying potential issues such as bugs, stylistic errors, and performance concerns early in the development lifecycle.
Use case 1: Run Linters in the Current Folder
Code:
golangci-lint run
Motivation:
Running linters in the current folder is a fundamental step in ensuring code quality. By executing this command, developers can quickly identify and address various issues in their Go code, ranging from syntax errors to more complex logical inconsistencies. This use case is particularly useful during active development when code changes are frequent, as it allows developers to maintain a continuous integration mindset by regularly checking their code against predefined quality standards.
Explanation:
golangci-lint
: This specifies the command line tool being used, which is designed for running multiple golang linters efficiently.run
: This subcommand directs golangci-lint to start its main action, which is running all the enabled linters on the Go code located within the current directory. By default, a wide variety of linters are run, each specializing in detecting different kinds of potential issues in the code.
Example Output:
foo.go:10:6: `bar` is unused (deadcode)
baz.go:15:10: expected type, found 'EOF' (varcheck)
Total issues: 2
This output shows the issues found including their location (file and line), type of problem, and the specific linter that identified the issue, helping developers quickly pinpoint areas that need attention.
Use case 2: List Enabled and Disabled Linters
Code:
golangci-lint linters
Motivation:
Sometimes a developer needs to know which linters are currently active in their configuration, as each linter typically searches for a distinct set of issues. By listing enabled and disabled linters, developers can ensure they are using the correct configuration or adjust it according to specific project needs. Adjusting linters allows teams to focus on certain quality aspects and maintain a consistent codebase based on their unique requirements.
Explanation:
golangci-lint
: This is the name of the tool being used.linters
: This subcommand lists all available linters, categorizing them into enabled and disabled based on the current configuration or defaults. It can be useful to ensure that the linter suite is comprehensive yet not redundant or overly aggressive.
Example Output:
Enabled linters:
deadcode
errcheck
gosimple
govet (vet)
Disabled linters:
goconst
gocyclo
golint
The output reveals which linters are participating in the analysis and which are excluded, enabling developers to tune their setup to maximize effectiveness and relevance.
Use case 3: Enable a Specific Linter for this Run
Code:
golangci-lint run --enable errcheck
Motivation:
In certain scenarios, a developer might want to test a specific aspect of their code by enabling a particular linter temporarily. This approach is beneficial when a specific issue is suspected, like unchecked errors, and the developer wants to perform a targeted check without altering the global configuration persistently. This flexibility is essential in a dynamic development process where quick iterations and testing of specific conditions can lead to more robust code.
Explanation:
golangci-lint
: This indicates the command-line tool in use.run
: As before, this tells the tool to perform its main task of running linters.--enable
: This flag instructs golangci-lint to activate a specific linter manually, even if it is not enabled by default in the current configuration.errcheck
: This is the name of the specific linter being activated for this execution. The ’errcheck’ linter, for instance, checks for unchecked errors, a common source of bugs in Go programs.
Example Output:
foo.go:22:9: unchecked error (errcheck)
Total issues: 1
The specific output highlights an unchecked error, providing line-specific feedback that alerts the developer to a potentially significant issue that needs addressing.
Conclusion
Golangci-lint stands out as an essential tool for Go developers aiming to maintain high standards of code quality. By leveraging its parallelized linter running capabilities and easy integration with various development environments, it helps developers quickly identify and address code issues. From comprehensive checks across all codebases to focused analysis using specific linters, golangci-lint provides versatile options to elevate the coding process and ensure the delivery of robust, clean, and maintainable software.