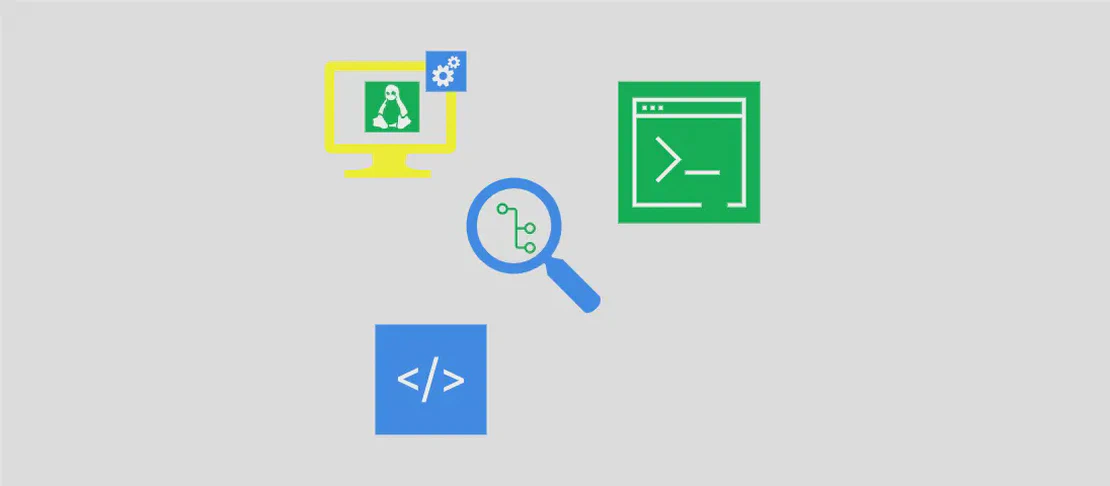
How to Use the Command 'gprof' (with Examples)
The ‘gprof’ command is a powerful performance analysis tool used to profile function executions within a program. This command is indispensable for developers seeking to optimize their code by understanding where the most time or resources are spent during execution. Gprof is a general-purpose profiler that generates reports indicating the frequency and duration of function calls in a program, allowing developers to identify bottlenecks and inefficient code paths.
To utilize ‘gprof’, a program must be compiled with specific flags that enable profiling. Once this is done, executing the program generates a profiling data file, which ‘gprof’ can then interpret to produce a detailed performance report. This tool supports many programming languages, with a primary focus on those compiled with GCC (GNU Compiler Collection).
Use Case 1: Compile binary with gprof information and run it to get gmon.out
Code:
gcc -pg program.c && ./a.out
Motivation for Using the Example:
Before you can analyze a program’s performance with ‘gprof’, you need to prepare by producing a special executable that will collect performance data during its run. This requires the program to be compiled with the -pg
flag, which instructs GCC to include extra profiling information in the executable. Running this executable generates a gmon.out
file that will contain the runtime statistics needed for later analysis with ‘gprof’.
Explanation for the Command Arguments:
gcc
: This is the GNU Compiler Collection command used to compile C programs. In this context, it is being used to compile ‘program.c’.-pg
: A flag provided to the GCC compiler that causes the produced binary to include profiling information, enabling the collection of runtime data.program.c
: The name of the C source file being compiled../a.out
: Executes the compiled binary, which then creates thegmon.out
file upon completion. This file contains the collected performance data necessary for profiling.
Example Output:
The compilation process will produce an executable named ‘a.out’, and upon execution, the command will produce an output similar to the normal running of the program. After program execution, a file named gmon.out
will appear in the directory, ready for the next step with ‘gprof’.
Use Case 2: Run gprof to obtain profile output
Code:
gprof
Motivation for Using the Example:
Once the required profiling data is collected into gmon.out
, you need to extract meaningful insights from it. Running ‘gprof’ without any arguments by default uses gmon.out
and the executable file to generate a human-readable performance summary. This report gives you insights into how often each function is called and how much time is spent in each execution.
Explanation for the Command Arguments:
gprof
: Invoked without any flags or parameters, it will automatically read from the defaultgmon.out
file and the executable used to produce it, generating a profiling report to standard output.
Example Output:
The output will be a verbose report showing statistics such as the number of calls to each function, how much time is spent in each function, and more, resembling:
Flat profile:
Each sample counts as 0.01 seconds.
...
Use Case 3: Suppress profile field’s description
Code:
gprof -b
Motivation for Using the Example:
The standard ‘gprof’ output includes explanatory text that describes each field in the report. While helpful, this can make the output cumbersome for experienced users who are already familiar with the profiling data format. By using the -b
option, the report is cleaner, focusing strictly on statistical information, which is beneficial for script processing or when clarity and brevity are needed.
Explanation for the Command Arguments:
gprof
: The command used to analyze the profiling data.-b
: This argument tells ‘gprof’ to suppress printing the description text for each field in the output, resulting in a cleaner and more concise report.
Example Output:
The output will primarily include just the raw data:
Flat profile:
...
The descriptive explanations accompanying each section will be omitted.
Use Case 4: Display routines that have zero usage
Code:
gprof -bz
Motivation for Using the Example:
Sometimes, it’s important to know which parts of a codebase are never executed. This is essential for code coverage analysis and can help identify dead code or functions that might be broken or misconfigured. The -bz
option in ‘gprof’ is particularly useful for this scenario as it allows developers to quickly spot code that is compiled but not run in practice.
Explanation for the Command Arguments:
gprof
: Engages the profiling analysis on the compiled binary using data fromgmon.out
.-b
: Suppresses the fields’ description, making the report more succinct.-z
: This option asks ‘gprof’ to display information about functions that have zero calls or usage, highlighting portions of code that aren’t being executed.
Example Output:
The output will include all functions, even those that were not invoked:
Call graph (explanation follows)
index % time self children called name
...
The report will list functions with a call count of zero, indicating areas to review for potential issues or unnecessary code.
Conclusion:
The ‘gprof’ command is an advanced profiling tool that, when used effectively, provides invaluable insights into program performance. Its versatility in analyzing function execution, ranging from highlighting unused code to generating a comprehensive time profile, makes it indispensable for developers targeting efficiency and performance optimization. Properly compiling and executing programs with ‘gprof’ enables a deeper understanding of application behavior and aids significantly in pinpointing areas for improvement.