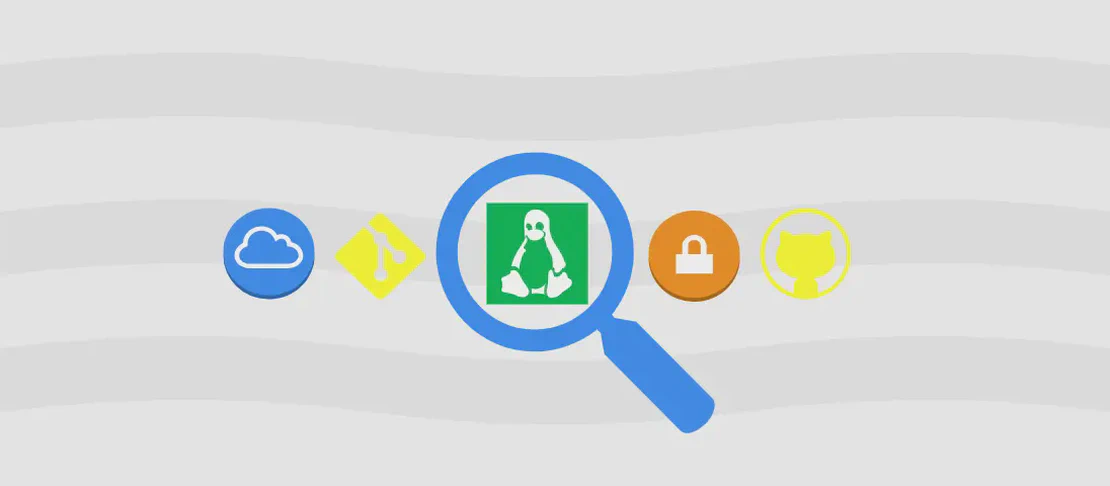
How to Use the Command 'gradle' (with Examples)
Gradle is an open-source build automation system designed to support the building, testing, deploying, and packaging lifecycle of applications. As a versatile tool, Gradle is extensively used in environments like Java projects and Android developments, providing consistent, reproducible builds with incremental improvement capabilities. It automates tasks often integrated into software development cycles, helping developers efficiently manage dependencies and workflows. This article will walk you through various use cases of the Gradle command, offering clear examples for each scenario.
Compile a Package
Code:
gradle build
Motivation: The gradle build
command is foundational for software development, particularly in compiling the source code and creating a deployable artifact. It’s designed to automate the consistent creation of software builds. Every time modifications are made, a fresh build can be triggered using this command, ensuring that updates are correctly implemented and new features are appropriately integrated.
Explanation: The primary command here is build
. It tells Gradle to execute the default lifecycle tasks necessary for building the project. This typically includes compiling the source code, processing resources, and packaging binaries.
Example Output:
BUILD SUCCESSFUL in 45s
12 actionable tasks: 12 executed
Exclude Test Task
Code:
gradle build -x test
Motivation: Testing can sometimes be time-consuming, especially when making minor changes that do not affect the code under test. By excluding tests with the -x test
option, you speed up the compilation process during active development, allowing developers to focus on other tasks by skipping time-intensive test execution.
Explanation: The -x
flag is introduced in this command, which means exclude. It is followed by the task you wish to skip, test
in this case, which tells Gradle not to run the test suite during this build process.
Example Output:
BUILD SUCCESSFUL in 30s
11 actionable tasks: 11 executed, 1 up-to-date
Run in Offline Mode
Code:
gradle build --offline
Motivation: Running in offline mode is crucial when operating in a development environment with limited or no network connectivity. Gradle caches previously downloaded dependencies, allowing further builds to proceed without relying on network access, thus enhancing efficiency and reliability in constrained scenarios.
Explanation: The --offline
flag informs Gradle to utilize the cached copies of required dependencies, avoiding any network calls. This is vital for performance when the network is unreliable.
Example Output:
BUILD SUCCESSFUL in 40s
12 actionable tasks: 12 executed
Clear the Build Directory
Code:
gradle clean
Motivation: Cleaning the build directory is an essential step when dealing with build errors or unforeseen artifacts from previous builds. By executing this command, you ensure that all generated outputs (compiled codes, bytecode, etc.) are removed, allowing for a clean slate to build again, which helps in diagnosing and resolving build-related issues.
Explanation: The clean
task removes any directories and files generated by previous builds from the output directory. It wipes out all build artifacts giving a fresh environment for subsequent builds.
Example Output:
BUILD SUCCESSFUL in 1s
1 actionable task: 1 executed
Build an Android Package (APK) in Release Mode
Code:
gradle assembleRelease
Motivation: Building an APK in release mode is an integral part of preparing an Android application for distribution. This command assembles the binaries intended for release targets, optimizing and securing them with appropriate configurations, ready for deployment to app stores.
Explanation: The term assembleRelease
specifies that Gradle should create a release-ready APK. This version will have optimizations like code minification and resource shrinking applied, preparing the application for production distribution.
Example Output:
BUILD SUCCESSFUL in 2m 30s
35 actionable tasks: 35 executed
List the Main Tasks
Code:
gradle tasks
Motivation: Discovering the tasks defined within a Gradle project is beneficial in understanding and invoking relevant build processes. This insight is especially useful when trying to identify main tasks quickly or when onboarding new developers who need clarity on what tasks are available.
Explanation: The command tasks
lists common Gradle tasks applied to the project without an exhaustive breakdown. This provides a high-level overview of the typical tasks that one might frequently utilize.
Example Output:
Welcome to Gradle 7.0.1.
To run a build, run gradle <task> ...
Tasks runnable from root project 'exampleProject'
Build tasks
-----------
assemble - Assembles the outputs of this project.
Help tasks
----------
dependencies - Displays all dependencies declared in root project 'exampleProject'.
List All the Tasks
Code:
gradle tasks --all
Motivation: For detailed introspection, listing all tasks associated with a Gradle project helps in understanding every possible action the Gradle build script can perform. This is crucial for advanced users who may want to refine build processes or optimize task executions by knowing every hook at their disposal.
Explanation: The tasks --all
option extends beyond regular task listings to include all tasks exposed by the project’s plugins and configurations, thereby providing complete visibility into the Gradle scripts’ full capabilities.
Example Output:
Tasks runnable from root project 'exampleProject'
Build tasks
-----------
build - Assembles and tests this project.
...
Other tasks
-----------
clean - Deletes the build directory.
test - Runs the unit tests.
...
Conclusion:
The Gradle command-line interface (CLI) is fervently designed to foster robust software development practices by automating and managing multifaceted processes within the build lifecycle. This article highlights a range of Gradle use cases, elucidating both their importance and the mechanics behind them, thereby equipping developers with the acumen to more effectively utilize this powerful tool in their projects. Whether compiling, building APKs, or understanding every task within a build script, Gradle efficiently manages critical stages to facilitate efficient and error-free software development.