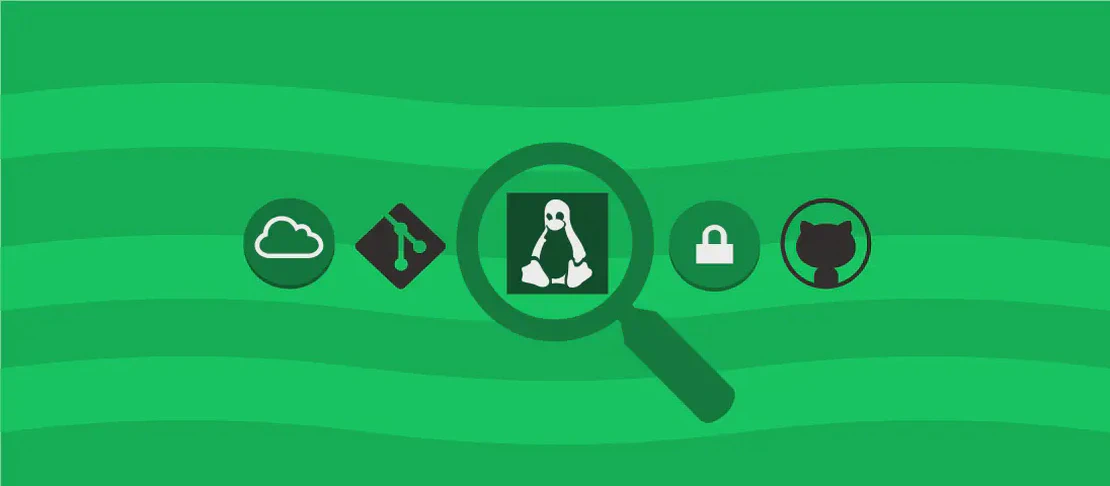
Redirecting Output Using Bash's Greater Than Command (with examples)
In Unix-based systems, the ability to redirect output is a crucial feature that allows users to manage where their program’s output is sent. This can be particularly useful for organizing scripts, logging important events, or simply directing information away from the terminal. The greater than (>
) symbol in Bash is a common method for redirecting output from the terminal to a file or other destinations. Below, we delve into various use cases of this command, exploring how it can be utilized to streamline your command-line operations.
Use case 1: Redirect stdout
to a file
Code:
command > path/to/file
Motivation:
Redirecting the standard output (stdout
) of a command to a file is particularly useful when you need to save the output for later analysis or documentation purposes. Rather than displaying output directly on the terminal, you can store it in a file where it can be reviewed at a more convenient time.
Explanation:
command
: This represents the command whose output you wish to redirect. It could be anything from a simpleecho
to a complex script.>
: The greater than symbol is the redirection operator, signaling that the output should be directed away from the terminal.path/to/file
: This specifies the file’s path where you want to save the output. If the file already exists, its contents will be overwritten.
Example output:
Suppose you run echo "Hello, World!" > output.txt
, the text “Hello, World!” will be saved in output.txt
.
Use case 2: Append to a file
Code:
command >> path/to/file
Motivation: Appending output to a file rather than overwriting it is extremely useful for creating logs or maintaining a cumulative record without losing previous data. This is particularly beneficial when you want to record incremental outputs over time.
Explanation:
command
: This can be any executable command that produces output.>>
: This is the append redirection operator. It appends the output of the command to the file atpath/to/file
.path/to/file
: This is the file to which the output will be appended. The existing contents of the file will remain unchanged, and new content is added to the end.
Example output:
If you run echo "New Log Entry" >> log.txt
, the phrase “New Log Entry” will be appended to the log.txt
file.
Use case 3: Redirect both stdout
and stderr
to a file
Code:
command &> path/to/file
Motivation: There are scenarios where a command might generate both standard output and error messages. Redirecting these to a single file helps in debugging and auditing, as it combines regular output and error diagnostics in one place for easier access and analysis.
Explanation:
command
: Represents the command producing output and potential error messages.&>
: This symbol is used to redirect bothstdout
(file descriptor 1) andstderr
(file descriptor 2) to the same destination.path/to/file
: The file where both output streams will be stored, overwriting existing content.
Example output:
Executing rm non_existent_file &> error_log.txt
will capture both the error message generated and any other output from the command in error_log.txt
.
Use case 4: Redirect both stdout
and stderr
to /dev/null
to keep the terminal output clean
Code:
command &> /dev/null
Motivation:
Sometimes, the output and error messages of a command might be unnecessary or clutter your terminal, especially if you’re executing multiple commands or running scripts in the background. Redirecting all output to /dev/null
effectively silences the command and keeps your terminal output clean.
Explanation:
command
: This is the command you wish to silence.&>
: Redirects both standard and error output streams./dev/null
: This is a special file that discards all data written to it. Redirecting output to/dev/null
ensures that it is completely ignored.
Example output:
Running find / -name "secret.txt" &> /dev/null
will suppress any output or error messages, making them invisible on the terminal.
Use case 5: Clear the file contents or create a new empty file
Code:
> path/to/file
Motivation: Clearing the contents of a file or creating a new empty file is a straightforward way to reset logs or prepare a new file for data entry. This can be particularly useful when scripts need to start with a clean state before logging new data.
Explanation:
>
: This redirection symbol, when used without specifying a command, effectively resets the contents of the specified file.path/to/file
: This is the file that will be cleared. If the file does not exist, it will be created anew as an empty file.
Example output:
Upon executing > report.log
, report.log
will either become empty if it already exists, or be created as an empty file if it didn’t exist beforehand.
Conclusion:
Understanding how to use the greater than (>
) redirection operator in Bash provides powerful tools for managing output, organizing logs, suppressing unnecessary terminal clutter, and more. Each use case enables efficient workflows and helps maintain clean and structured environments, be it for single commands or complex scripts.