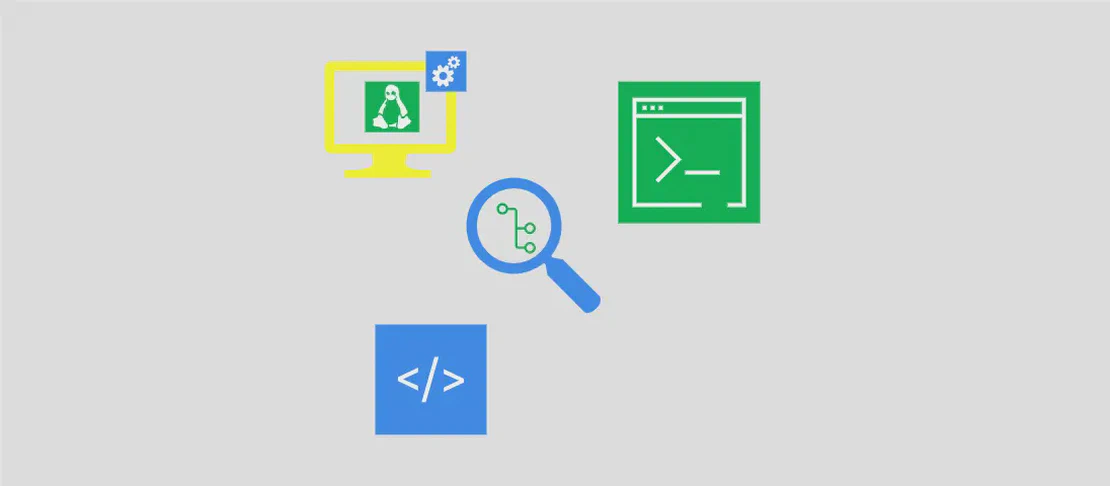
How to use the command 'grex' (with examples)
The ‘grex’ command is a tool that generates regular expressions based on given input. It is especially useful for users who are not familiar with regular expressions or find it time-consuming to create complex patterns manually. ‘grex’ simplifies the process by automatically generating regular expressions tailored to specific use cases.
Use case 1: Generate a simple regular expression
Code:
grex space_separated_strings
Motivation: Generating a regular expression for a specific pattern can be challenging and time-consuming. With the ‘grex’ command, users can quickly generate a regular expression based on the given input strings. This can be useful when trying to match or tokenize specific patterns in text or data.
Explanation: The ‘space_separated_strings’ argument represents the input strings from which the regular expression will be generated. ‘grex’ analyzes the input strings and creates a regular expression pattern that matches those strings.
Example output:
(space separated strings)
Explanation: The generated regular expression matches the exact string “space separated strings”.
Use case 2: Generate a case-insensitive regular expression
Code:
grex -i space_separated_strings
Motivation: Sometimes, it is necessary to match patterns in a case-insensitive manner. For example, when searching for specific words or phrases in text, it may be useful to find matches regardless of uppercase or lowercase variations. By using the ‘-i’ flag with the ‘grex’ command, users can generate a regular expression that is case-insensitive.
Explanation: The ‘-i’ flag indicates that the generated regular expression should be case-insensitive. This means that the regular expression pattern will match both uppercase and lowercase characters.
Example output:
(?:space\s+separated\s+strings)
Explanation: The generated regular expression matches the string “space separated strings” while allowing for multiple spaces between each word.
Use case 3: Replace digits with ‘\d’
Code:
grex -d space_separated_strings
Motivation: In some cases, it is useful to match or replace digits in a string. The ‘\d’ expression is commonly used in regular expressions to represent any digit character. By using the ‘-d’ flag with the ‘grex’ command, users can generate a regular expression that specifically targets digits in the input strings.
Explanation: The ‘-d’ flag instructs ‘grex’ to replace any occurrence of a digit in the input strings with ‘\d’. This allows the generated regular expression to match any digit character.
Example output:
(?:space\s+separated\s+strings)
Explanation: The generated regular expression remains the same as in the previous use case. As the input strings do not contain any digit characters, the ‘\d’ replacement does not have any effect.
Use case 4: Replace Unicode word character with ‘\w’
Code:
grex -w space_separated_strings
Motivation: When working with text data, it can be useful to match or replace word characters. Word characters typically include alphanumeric characters and underscores. The ‘\w’ expression in regular expressions represents any word character. By using the ‘-w’ flag with the ‘grex’ command, users can generate a regular expression that specifically targets word characters in the input strings.
Explanation: The ‘-w’ flag instructs ‘grex’ to replace any occurrence of a Unicode word character in the input strings with ‘\w’. This allows the generated regular expression to match any word character.
Example output:
(?:space\s+separated\s+strings)
Explanation: The generated regular expression remains the same as in the previous use cases. As the input strings do not contain any Unicode word characters, the ‘\w’ replacement does not have any effect.
Use case 5: Replace spaces with ‘\s’
Code:
grex -s space_separated_strings
Motivation: In some cases, it is necessary to match or replace spaces in text or data. The ‘\s’ expression in regular expressions represents any whitespace character, including spaces, tabs, and newlines. By using the ‘-s’ flag with the ‘grex’ command, users can generate a regular expression that specifically targets spaces in the input strings.
Explanation: The ‘-s’ flag instructs ‘grex’ to replace any occurrence of a space character in the input strings with ‘\s’. This allows the generated regular expression to match any whitespace character.
Example output:
space\s+separated\s+strings
Explanation: The generated regular expression matches the string “space separated strings” while allowing for multiple spaces between each word.
Use case 6: Add {min, max} quantifier representation for repeating sub-strings
Code:
grex -r space_separated_strings
Motivation: In regular expressions, quantifiers are used to specify the number of occurrences of a sub-pattern. By default, ‘grex’ generates regular expressions with the ‘+’ quantifier, which matches one or more occurrences of the previous sub-pattern. However, it can be useful to specify a range of minimum and maximum occurrences. By using the ‘-r’ flag with the ‘grex’ command, users can add the {min, max} representation to quantify repeating sub-strings.
Explanation: The ‘-r’ flag instructs ‘grex’ to add the {min, max} representation to quantify repeating sub-strings in the generated regular expression. The ‘space_separated_strings’ argument represents the input strings.
Example output:
space\s+separated\s+strings
Explanation: The generated regular expression remains the same as in the previous use cases. As the input strings do not contain any repeating sub-strings, the {min, max} representation does not have any effect.
Conclusion:
The ‘grex’ command is a powerful tool for automatically generating regular expressions based on given input strings. It simplifies the process of creating complex regular expression patterns and can be utilized in various use cases. The examples provided demonstrate different aspects of ‘grex’, such as generating case-insensitive expressions, replacing specific character types, and quantifying repeating sub-strings. By understanding and utilizing the various options and flags available with ‘grex’, users can save time and effort in creating regular expressions for their specific needs.