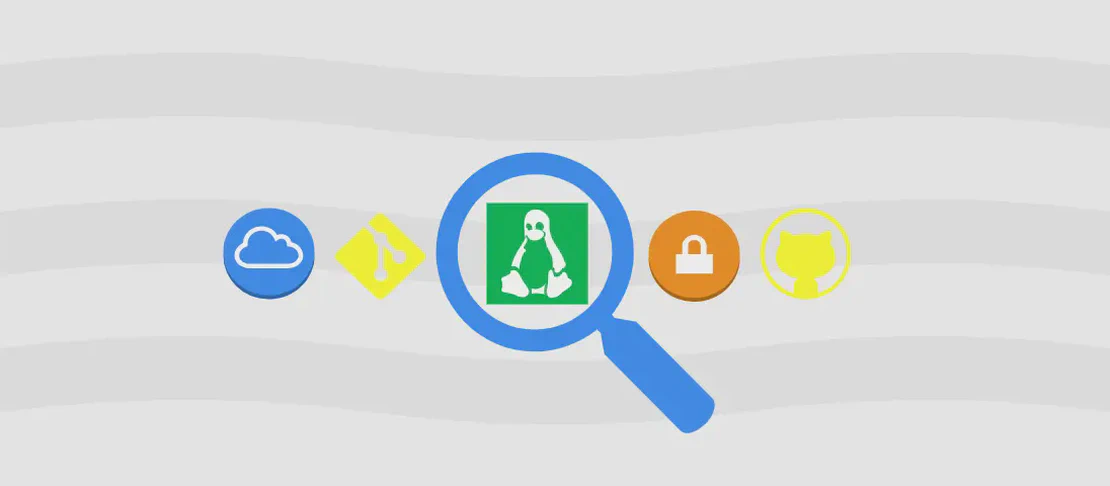
How to Use the Command 'grpcurl' (with Examples)
grpcurl
is a powerful command-line tool designed for interacting with gRPC servers, akin to how curl
is used for HTTP/HTTPS-based servers. It allows developers to send requests to gRPC servers and capture their responses without needing to write explicit client code in a specific programming language. This flexibility comes in handy during development, debugging, and testing of gRPC-based APIs. The tool can automatically discover available services and methods on gRPC servers, simplifying the process of interaction.
Use Case 1: Send an Empty Request
Code:
grpcurl grpc.server.com:443 my.custom.server.Service/Method
Motivation:
Sending an empty request is a basic yet valuable operation when first testing connectivity to a service. Whether you’re verifying that your network can communicate with the server or quickly running a default endpoint without any parameters, testing an empty request can serve as a foundational step in working with gRPC APIs.
Explanation:
grpcurl
: This is the command-line tool being used to interact with the gRPC server.grpc.server.com:443
: Specifies the server address and port number thatgrpcurl
should connect to. In this case, it defaults to the common secure port 443 for gRPC over TLS/SSL.my.custom.server.Service/Method
: Denotes the specific service and method on the gRPC server you wish to call. This follows the patternService/Method
, targeting a specific RPC endpoint.
Example Output:
{
"responseField": "responseValue"
}
This response indicates a successful call to the method, with the server providing a default or empty response.
Use Case 2: Send a Request with a Header and a Body
Code:
grpcurl -H "Authorization: Bearer $token" -d '{"foo": "bar"}' grpc.server.com:443 my.custom.server.Service/Method
Motivation:
Sending a request with both headers and a body is vital when dealing with secured APIs or those requiring specific data inputs. Authorization headers, such as Bearer tokens, are commonly necessary for authenticated endpoints, while the data body allows the user to provide detailed input parameters to the service method.
Explanation:
-H "Authorization: Bearer $token"
: Adds a header to the request. Here,Authorization: Bearer $token
is used to pass authentication credentials, with$token
typically representing a dynamically assigned string or an environmental variable containing the security token.-d '{"foo": "bar"}'
: Specifies the JSON format data payload sent in the body of the request. In this case,{ "foo": "bar" }
represents a sample input object.grpc.server.com:443 my.custom.server.Service/Method
: As previously explained, this addresses the specific server and endpoint you are accessing.
Example Output:
{
"result": "success",
"details": "Data processed successfully"
}
A response like this highlights that the request was received and processed correctly, indicating that the input data was accepted and utilized by the server.
Use Case 3: List All Services Exposed by a Server
Code:
grpcurl grpc.server.com:443 list
Motivation:
Listing all services on a gRPC server is an exploratory task, hugely beneficial when familiarizing yourself with the available endpoints on a given server. This is particularly useful for developers who might not have detailed documentation at hand or need to quickly audit the server capabilities.
Explanation:
list
: This command flag indicates togrpcurl
that the user desires a listing of all available services exposed by the server.grpc.server.com:443
: Directsgrpcurl
to connect to the specified server on port 443 to retrieve the service list.
Example Output:
my.custom.server.Service
another.custom.server.Service
yet.another.server.Service
The output provides a concise list of all services, aiding users in planning further interactions.
Use Case 4: List All Methods in a Particular Service
Code:
grpcurl grpc.server.com:443 list my.custom.server.Service
Motivation:
Discovering all methods within a specific service helps users understand the functionalities offered by that service, assisting in both exploration and learning phases. This can be essential for determining the exact method needed for a particular integration or feature development task.
Explanation:
list my.custom.server.Service
: Enhances the list command by specifying interest in a certain service, promptinggrpcurl
to display all available methods tied to this service.grpc.server.com:443
: Specifies which gRPC server hosts the service you want to inspect.
Example Output:
my.custom.server.Service.MethodOne
my.custom.server.Service.MethodTwo
my.custom.server.Service.MethodThree
The result is a detailed list of method endpoints available within the specified service, showing users what actions or data requests they can perform.
Conclusion:
grpcurl
proves to be an indispensable tool for developers working with gRPC servers, offering a variety of options for sending requests and exploring available services and methods. By using the command’s capabilities as demonstrated, users can efficiently interact with APIs, whether testing connectivity, sending secured requests, or conducting discovery operations.