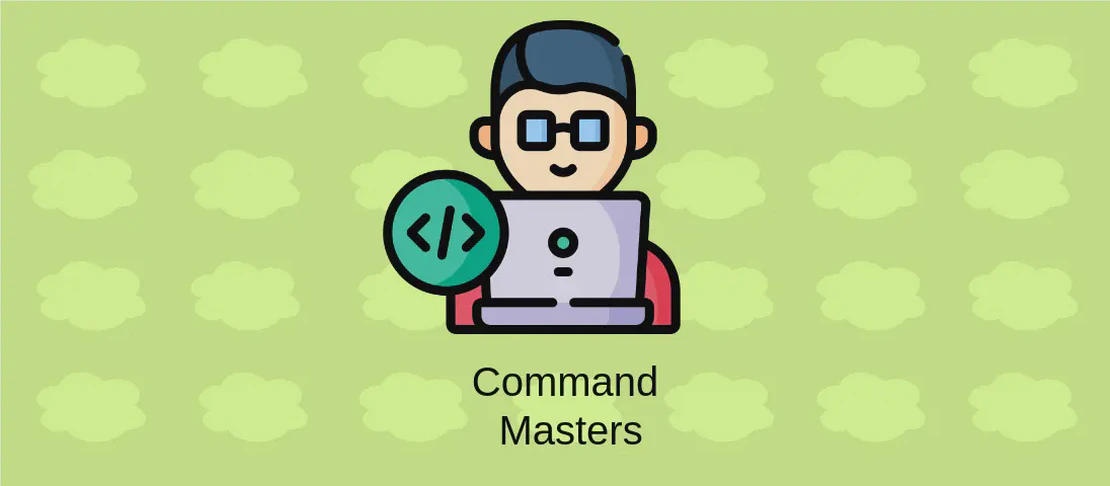
Utilizing 'gsutil' for Google Cloud Storage Management (with examples)
Google Cloud Storage is a powerful solution for storing and accessing your data reliably and securely. One of the primary tools to interact with Google Cloud Storage is the gsutil
command-line tool. gsutil
allows users to manage their storage buckets and objects efficiently. It is part of the Google Cloud SDK and provides a wide range of functionalities, including listing buckets, uploading and downloading objects, and managing storage configurations. By executing simple commands, users can perform complex storage management tasks, making it an essential tool for those looking to leverage Google Cloud’s capabilities.
Use case 1: List all buckets in a project you are logged into
Code:
gsutil ls
Motivation:
Listing all buckets in your project is a common task that helps you manage and evaluate your existing storage resources. It serves as a way to get a quick overview of what buckets you have created within your project. This can be especially useful in managing projects that involve numerous teams or departments, ensuring that no resources are left unmanaged.
Explanation:
gsutil
: The command-line tool for accessing Google Cloud Storage.ls
: The command that lists your cloud storage assets. When used without additional arguments, it searches and retrieves all buckets within the current project.
Example Output:
gs://example-bucket-1/
gs://example-bucket-2/
gs://example-bucket-3/
This output lists all the buckets available in your Google Cloud project.
Use case 2: List the objects in a bucket
Code:
gsutil ls -r 'gs://bucket_name/prefix**'
Motivation:
Listing objects within a bucket allows users to understand their existing data structure and organization. Particularly in cases where regular updates and adjustments are made, seeing the collection of objects provides a snapshot of what’s currently stored.
Explanation:
gsutil
: Google Cloud Storage command-line tool.ls
: Command to list objects and information.-r
: Recursive flag that lists all objects inside the specified bucket and its subdirectories.'gs://bucket_name/prefix**'
: The specified path where objects are stored. Theprefix**
allows users to target specific folders or file structures.
Example Output:
gs://bucket_name/folder1/
gs://bucket_name/folder1/file1.txt
gs://bucket_name/folder1/file2.txt
The above output shows the hierarchy of files and directories within the specified bucket.
Use case 3: Download an object from a bucket
Code:
gsutil cp gs://bucket_name/object_name path/to/save_location
Motivation:
Downloading objects from a cloud storage bucket is often required for data processing, analysis, or backup purposes. This action allows continuous integration practices or updates to use the latest data available.
Explanation:
gsutil
: Tool to interface with Google Cloud Storage.cp
: Command to copy the specified item from the source to a destination.gs://bucket_name/object_name
: The cloud location of the object you want to download.path/to/save_location
: The local file system path where you want to save the downloaded data.
Example Output:
Copying gs://bucket_name/object_name...
[100%] Download complete.
The object is successfully downloaded to the specified local path.
Use case 4: Upload an object to a bucket
Code:
gsutil cp object_location gs://destination_bucket_name/
Motivation:
Uploading objects to Google Cloud Storage is essential for creating backups, sharing data with other services or teams, or simply storing large datasets offsite for reliability and availability.
Explanation:
gsutil
: Command-line tool for Google Cloud Storage.cp
: Stands for ‘copy’, used to transfer files.object_location
: Path to the local file you wish to upload.gs://destination_bucket_name/
: Destination bucket path where the file will be stored.
Example Output:
Uploading object_location to gs://destination_bucket_name...
[100%] Upload complete.
Successful transfer and upload of the file to the cloud bucket.
Use case 5: Rename or move objects in a bucket
Code:
gsutil mv gs://bucket_name/old_object_name gs://bucket_name/new_object_name
Motivation:
Renaming or moving files within a bucket is vital for data organization and management. As project scopes and data structures evolve, certain data restructures are necessary to maintain a logical and efficient storage system.
Explanation:
gsutil
: The command-line tool reference.mv
: Command for moving or renaming an object.gs://bucket_name/old_object_name
: The current location and name of the file.gs://bucket_name/new_object_name
: The new location and/or name of the file.
Example Output:
Renaming gs://bucket_name/old_object_name to gs://bucket_name/new_object_name...
Success.
The object is moved to or renamed with the new specified identifier.
Use case 6: Create a new bucket in the project you are logged into
Code:
gsutil mb gs://bucket_name
Motivation:
Creating new buckets is fundamental for initiating projects or expanding existing storage options. Dedicated buckets can be organized for specific types of data or datasets to manage permissions and accessibility.
Explanation:
gsutil
: Google Cloud Storage command-line utility.mb
: Command that signifies ‘make bucket’.gs://bucket_name
: The intended name and path for the new bucket.
Example Output:
Creating gs://bucket_name/...
Bucket created successfully.
A new bucket is created in the current project environment.
Use case 7: Delete a bucket and remove all the objects in it
Code:
gsutil rm -r gs://bucket_name
Motivation:
Deleting buckets is necessary for managing costs, resources, and cleaning up after a project is completed or when certain data is no longer required under storage. It ensures the efficient use of cloud resources while maintaining organized storage.
Explanation:
gsutil
: The command used for interacting with Google Cloud Storage.rm
: The ‘remove’ command to delete files.-r
: Recursive flag that ensures all contents and subdirectories of the bucket are deleted.gs://bucket_name
: The specific bucket that is being permanently removed.
Example Output:
Removing gs://bucket_name/...
All contents deleted and bucket removed.
The bucket and all its contents are successfully removed from cloud storage.
Conclusion:
The gsutil
command-line tool provides essential services for managing Google Cloud Storage. From listing and organizing your buckets, uploading and downloading objects, to creating new resources and deleting old ones, gsutil
offers a comprehensive toolkit for your cloud data management needs. These examples illustrate how even the simplest commands can provide powerful and effective control over your data storage.