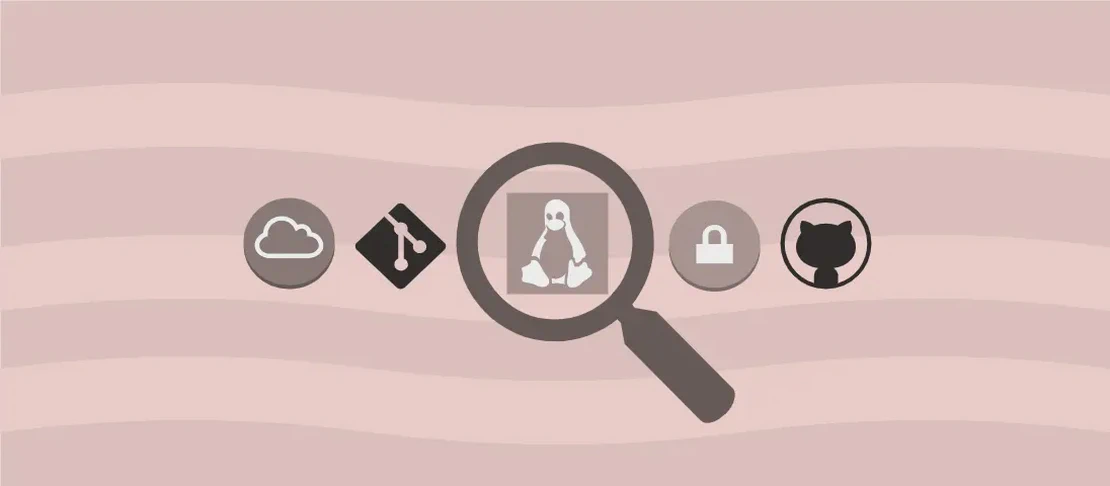
How to Use the Command 'gulp' (with examples)
Gulp is a JavaScript task runner and streaming build system that is widely used to automate time-consuming and repetitive tasks involved in web development workflows. It offers a simple platform for defining tasks, executing them in a streamlined manner, and managing project builds through a series of automation processes. Gulp tasks are defined within a gulpfile.js
, located at the root of the project directory. These tasks can range from simple file minification and image optimization to complex build pipelines.
Use case 1: Run the default task
Code:
gulp
Motivation:
Running the default task is perhaps the most common use case when using Gulp. By executing the simply typed command gulp
, you’re telling Gulp to carry out the predefined ‘default’ tasks in your gulpfile.js
. This is useful in scenarios where you want to initiate the initial build or perform the entire set of actions you have designated as base operations. Typically, the default task is configured to execute a sequence that establishes the foundation needed for further development or deployment.
Explanation:
gulp
: This command invokes Gulp in the terminal and, by default, runs the task designated as ‘default’ within yourgulpfile.js
. It does not require any additional arguments when you’re intending to execute the typical task sequence set for general operations.
Example output:
[13:12:49] Using gulpfile ~/your_project/gulpfile.js
[13:12:49] Starting 'default'...
[13:12:49] Starting 'clean'...
[13:12:49] Finished 'clean' after 16 ms
[13:12:49] Starting 'build-scripts'...
[13:12:50] Successfully built scripts
[13:12:50] Finished 'build-scripts' after 565 ms
[13:12:50] Starting 'compile-scss'...
[13:12:51] Successfully compiled SCSS
[13:12:51] Finished 'compile-scss' after 243 ms
[13:12:51] Finished 'default' after 829 ms
Use case 2: Run individual tasks
Code:
gulp task othertask
Motivation:
Often, there is a need to execute specific tasks rather than the entire list within the default workflow. For instance, you might want to regenerate CSS files or recompile JS only for sections that were recently changed, rather than running the entire stack of tasks. Running individual tasks with gulp task othertask
allows developers to save time and resources by focusing solely on components that need adjustment or testing, thereby enhancing efficiency and productivity.
Explanation:
gulp
: This calls upon the Gulp tool to interact with tasks found ingulpfile.js
.task
: This is a placeholder for any user-defined task name included in yourgulpfile.js
. It calls upon a specific task to be executed.othertask
: Similarly, this represents another task you may want to run concurrently or separately. By specifying one or more tasks, the command executes only these, following the sequence provided.
Example output:
[13:20:12] Using gulpfile ~/your_project/gulpfile.js
[13:20:12] Starting 'task'...
[13:20:12] Task has completed!
[13:20:12] Finished 'task' after 45 ms
[13:20:12] Starting 'othertask'...
[13:20:13] Othertask is in progress
[13:20:13] Finished 'othertask' after 80 ms
Use case 3: Print the task dependency tree for the loaded gulpfile
Code:
gulp --tasks
Motivation:
Understanding the dependency structure of your project’s tasks is crucial for efficient workflow management and troubleshooting. By displaying a task dependency tree using gulp --tasks
, developers can obtain a clear picture of task sequences and interdependencies as defined within the gulpfile.js
. This is particularly useful for new team members, debugging tasks dependencies issues, or when extending existing workflows.
Explanation:
gulp
: Invokes the Gulp command line interface.--tasks
: This option requests Gulp to output a hierarchical representation of the tasks and their dependencies without actually running them. It provides insight into the task orchestration at a glance.
Example output:
[13:25:30] Using gulpfile ~/your_project/gulpfile.js
[13:25:30] Tasks for ~/your_project/gulpfile.js
[13:25:30] ├── default
[13:25:30] ├── build-scripts
[13:25:30] ├── compile-scss
[13:25:30] ├── watch
[13:25:30] ├── clean
Conclusion:
Understanding how to leverage Gulp effectively can significantly streamline the development process and foster more efficient workflows. By utilizing the default task, selectively running individual tasks, or mapping out the task dependency tree, developers can tailor their builds precisely to their project needs, reducing overhead and focusing resources where they’re needed most. Through these use cases, we see how Gulp provides not only a powerful automation tool but also a flexible framework for managing complex build systems with ease.