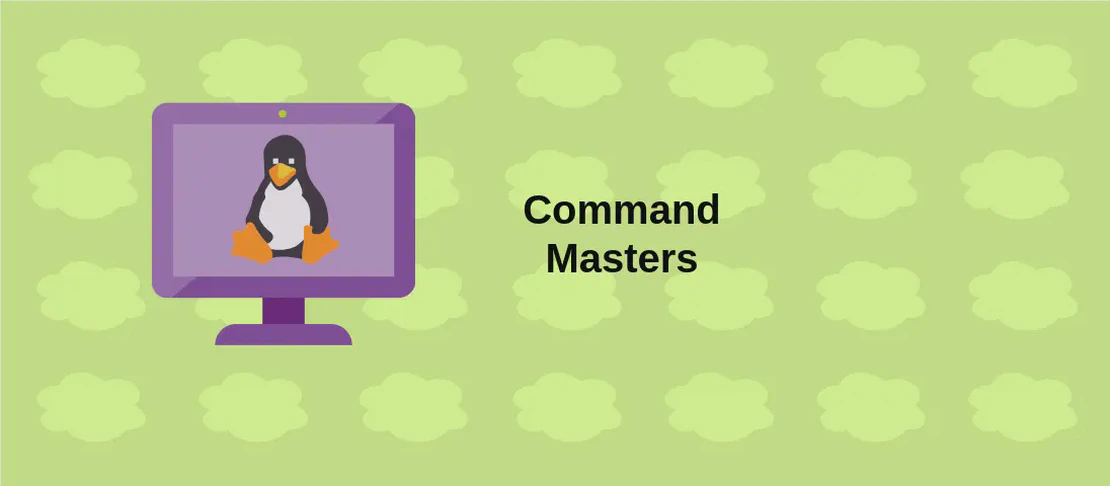
How to Use the Command 'gunicorn' (with Examples)
Gunicorn, short for ‘Green Unicorn’, is a Python WSGI (Web Server Gateway Interface) HTTP server. It’s a robust, scalable server primarily used to serve web applications written in Python. Gunicorn is compatible with various web frameworks, including Flask and Django, making it an essential tool for deploying production-ready web applications. The command line options allow you to fine-tune server behavior to suit specific requirements, such as binding to a particular port, enabling live reloads during development, managing worker processes and threads, and setting up HTTPS.
Run Python Web App
Code:
gunicorn import.path:app_object
Motivation:
Running a Python web application using Gunicorn is a common task for deploying applications in a robust environment. This command is utilized at the very basic level to initiate the Gunicorn server with the specified application object. Using this simple command allows for starting a web app without any additional customizations, perfect for local development or testing purposes.
Explanation:
gunicorn
: Calls the Gunicorn command to start the server.import.path:app_object
: Specifies the module path and the application object. “import.path” refers to the path where your Python app resides, and “app_object” is the callable WSGI application object within your app.
Example Output:
[INFO] Starting gunicorn 20.x.x
[INFO] Listening at: http://127.0.0.1:8000 (PID:XXXX)
[INFO] Worker processes on the job...
Listen on Port 8080 on Localhost
Code:
gunicorn --bind localhost:8080 import.path:app_object
Motivation:
Listening on a specific port is crucial when multiple applications are running on the same server. By binding Gunicorn to localhost on port 8080, you ensure that the application listens only to internal network interfaces, enhancing security and allowing other applications to use different ports.
Explanation:
--bind localhost:8080
: Instructs Gunicorn to bind the server to “localhost” on port “8080”.import.path:app_object
: Denotes the application as previously explained.
Example Output:
[INFO] Listening at: http://127.0.0.1:8080 (PID:XXXX)
Turn on Live Reload
Code:
gunicorn --reload import.path:app_object
Motivation:
During active development, code changes occur frequently. Enabling live reload ensures the server automatically restarts whenever a code change is detected. This saves developers the hassle of manually restarting the server, which can be both time-consuming and error-prone when managing numerous files.
Explanation:
--reload
: Activates auto-reloading of the server.import.path:app_object
: As before, specifies the target WSGI application.
Example Output:
[INFO] Detected code changes. Reloading...
[INFO] Listening at: http://127.0.0.1:8000 (PID:XXXX)
Use 4 Worker Processes for Handling Requests
Code:
gunicorn --workers 4 import.path:app_object
Motivation:
Utilizing multiple worker processes increases the capacity to handle simultaneous requests, improving performance and reliability. This is particularly useful for applications expecting a high number of concurrent users. Increasing the number of workers helps in leveraging multi-core systems more efficiently.
Explanation:
--workers 4
: Specifies the server should utilize 4 separate worker processes to handle incoming requests.import.path:app_object
: Continues to specify the WSGI application.
Example Output:
[INFO] Using 4 workers...
[INFO] Listening at: http://127.0.0.1:8000 (PID:XXXX)
Use 4 Worker Threads for Handling Requests
Code:
gunicorn --threads 4 import.path:app_object
Motivation:
While processes are entirely separate, threads share components like memory, which can be more efficient for certain workloads. By assigning multiple threads to a worker process, you can further tune applications for optimal performance where threading is more beneficial than multiprocessing.
Explanation:
--threads 4
: Directs that each worker will run with 4 threads, enabling it to handle multiple requests simultaneously.import.path:app_object
: Specifies the application target as usual.
Example Output:
[INFO] Using 4 threads per worker...
[INFO] Listening at: http://127.0.0.1:8000 (PID:XXXX)
Run App over HTTPS
Code:
gunicorn --certfile cert.pem --keyfile key.pem import.path:app_object
Motivation:
Running applications over HTTPS encrypts the data transferred between client and server, enhancing security. This is essential for any service dealing with sensitive information or requiring secure connections. Using SSL/TLS certificates ensures trust and confidentiality for end-users accessing the service.
Explanation:
--certfile cert.pem
: Path to the SSL certificate file.--keyfile key.pem
: Path to the corresponding SSL private key file.import.path:app_object
: Your application’s WSGI entry point.
Example Output:
[INFO] Listening at: https://127.0.0.1:8000 (PID:XXXX)
[INFO] Secure connection established.
Conclusion:
Gunicorn is a powerful WSGI server that offers many flexible configurations for deploying Python web applications. By understanding and using its various options and configurations, you can significantly enhance the performance, security, and responsiveness of your applications in both development and production environments. The examples provided illustrate basic use cases, and mastering these concepts can lay the groundwork for more advanced configurations tailored to specific application needs.