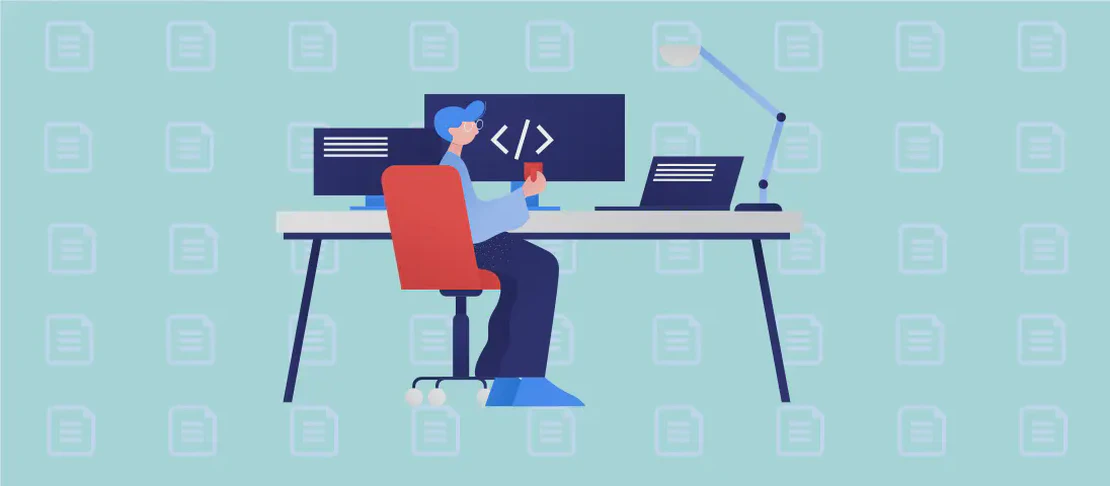
How to use the command 'help' (with examples)
- Linux
- December 25, 2023
The ‘help’ command is a built-in command in Bash that displays information about other built-in commands. It is a helpful tool for users who need assistance with understanding how to use specific commands or who want to explore all the available built-in commands.
Use case 1: Display the full list of builtin commands
Code:
help
Motivation: This use case is useful when users want to have a comprehensive list of all the built-in commands available in Bash. It allows users to quickly reference and explore the various commands.
Explanation: The ‘help’ command without any arguments will display the full list of builtin commands. It provides a brief description of each command along with the syntax and options if any.
Example output:
GNU bash, version 5.0.17(1)-release (x86_64-pc-linux-gnu)
These shell commands are defined internally. Type `help' to see this list.
Type `help name' to find out more about the function `name'.
Use `info bash' to find out more about the shell in general.
Use `man -k' or `info' to find out more about commands not in this list.
A star (*) next to a name means that the command is disabled.
job_spec [&] history [-c] [-d offset] [n] or history -anrw [filename] or history -ps arg [arg...]
(( expression )) if COMMANDS; then COMMANDS; [ elif COMMANDS; then COMMANDS; ]... [ else COMMANDS; ] fi
. filename [arguments] jobs [-lnprs] [jobspec ...] or jobs -x command [args]
: kill [-s sigspec | -n signum | -sigspec] pid | jobspec ... or kill -l [sigspec]
[ arg... ] let arg [arg ...]
[[ expression ]] local [option] name[=value] ...
alias [-p] [name[=value] ... ] logout [n]
bg [job_spec ...] mapfile [-d delim] [-n count] [-O origin] [-s count] [-t] [-u fd] [-C callback] [-c quantum] [array]
bind [-lpsvPSVX] [-m keymap] [-f filename] [-q name] [-r keyseq] [-x keyseq:shell-command] [keyseq:readline-func]
break [n] popd [-n] [+N | -N]
builtin [shell-builtin [arg ...]] printf [-v var] format [arguments]
…
Use case 2: Print instructions on how to use the while
loop construct
Code:
help while
Motivation: This use case is helpful when users want to understand how to use the while
loop construct in Bash. It provides information on the syntax and options available for the while
loop, making it easier for users to write and understand loop constructs.
Explanation: The ‘help while’ command will display instructions on how to use the while
loop construct in Bash. It explains the syntax and options available, such as condition evaluation and loop control.
Example output:
while: while COMMANDS; do COMMANDS; done
Expand and execute COMMANDS as long as the final command in the
`while' COMMANDS has an exit status of zero.
Exit Status:
Returns the status of the last command executed.
Use case 3: Print instructions on how to use the for
loop construct
Code:
help for
Motivation: When users are unfamiliar with the syntax and options of the for
loop construct in Bash, this use case is beneficial. It provides a clear overview of how to use the for
loop, allowing users to iterate over lists of items efficiently.
Explanation: The ‘help for’ command will provide instructions on how to use the for
loop construct in Bash. It explains the syntax, options, and control flow for iterating over values or elements in a list.
Example output:
for: for NAME [in WORDS ... ] ; do COMMANDS; done
Expand WORDS, and execute COMMANDS once for each member in the
resultant list, with NAME bound to the current member. If WORDS
is empty, then COMMANDS are executed once, if NAME is not bound,
then the `for' loop variable is set to each member of the list in
turn, and COMMANDS are executed. The return value is the exit
status of the last command that executes. If the `for' loop is
executed immediately after a `while' or `until' loop whose test
condition evaluates to false, then COMMANDS are not executed.
Exit Status:
The return status is the exit status of the last command executed,
or zero if no condition tested true.
Use case 4: Print instructions on how to use [[ ]]
for conditional commands
Code:
help [[ ]]
Motivation: This use case is useful when users want to understand the use of conditional commands in Bash. The ‘[[ ]]’ construct enables users to perform more complex conditional evaluations than the traditional ‘if’ statement, adding flexibility to their scripts.
Explanation: The ‘help [[ ]]’ command provides instructions on how to use the ‘[[ ]]’ construct for conditional commands in Bash. It explains the syntax and options available for performing conditional evaluations, including string comparisons, file tests, and logical operations.
Example output:
[[ expression ]]
Return a status of 0 or 1 depending on the evaluation of the
conditional expression expression. Expressions are composed of
the primaries described below under CONDITIONAL EXPRESSIONS. Word
splitting and pathname expansion are not performed on the words
between the [[ and ]].
Conditional Operators:
When used with [[, the < and > operators sort lexicographically
using the current locale.
Use case 5: Print instruction on how to use (( ))
to evaluate arithmetic expressions
Code:
help \( \)
Motivation: When users need to perform arithmetic evaluations within Bash, using the (( ))
construct is beneficial. This use case provides instructions on how to utilize the construct effectively, enabling users to perform arithmetic calculations and comparisons.
Explanation: The ‘help ( )’ command offers instructions on how to use the (( ))
construct to evaluate arithmetic expressions in Bash. It explains the supported arithmetic operators and provides guidance on performing mathematical calculations and logical comparisons.
Example output:
(( expression ))
The expression is evaluated according to the rules described below
under ARITHMETIC EVALUATION. If the value of the expression is
non-zero, the return status is 0; otherwise, the return status is
1. This is exactly equivalent to let "expression".
Exit Status:
Returns the exit status of the last command executed.
Use case 6: Print instructions on how to use the cd
command
Code:
help cd
Motivation: Understanding how to use the cd
command is crucial for navigating and switching directories within a Bash shell environment. This use case provides instructions on how to effectively use the cd
command, allowing users to easily change directories.
Explanation: The ‘help cd’ command displays instructions on how to use the cd
command in Bash. It provides the syntax, options, and examples of using the command to change directories, including relative and absolute paths.
Example output:
cd: cd [-L|[-P [-e]] [-@]] [dir]
Change the shell working directory.
Change the current directory to DIR. The default DIR is the value of
the HOME shell variable.
The variable CDPATH defines the search path for the directory containing
DIR. Alternative directory names in CDPATH are separated by a colon
(:). A null directory name is the same as the current directory,
i.e., `.'. If DIR begins with a slash (/), then CDPATH is not used.
If the directory is not found, and the shell option `cdable_vars' is set,
the word is assumed to be a variable name. If that variable has a value, its value is used for DIR.
Options:
-L force symbolic links to be followed: resolve symbolic
links in DIR after processing instances of `..'
-P use the physical directory structure without following
symbolic links: resolve symbolic links in DIR before
processing instances of `..'
-e if the -P option is supplied, and the current working directory
cannot be determined successfully, exit with a non-zero status
-@ on systems that support it, present a file with extended
attributes as a directory containing the file attributes
The default is to follow symbolic links, as if `-L' were specified.
`..' is processed by removing the immediately previous pathname component
back to a slash or the beginning of DIR.
Exit Status:
Returns 0 if the directory is changed, and if $PWD is set successfully when
-P is used; non-zero otherwise.
Conclusion:
The ‘help’ command is a valuable tool in Bash for obtaining information about built-in commands. It allows users to explore the available commands, understand their usage, and access detailed explanations for each command. Whether it’s obtaining a list of built-in commands, learning about loop constructs, understanding conditional evaluations, evaluating arithmetic expressions, or navigating directories, the ‘help’ command provides the necessary information to enhance users’ understanding of Bash commands.