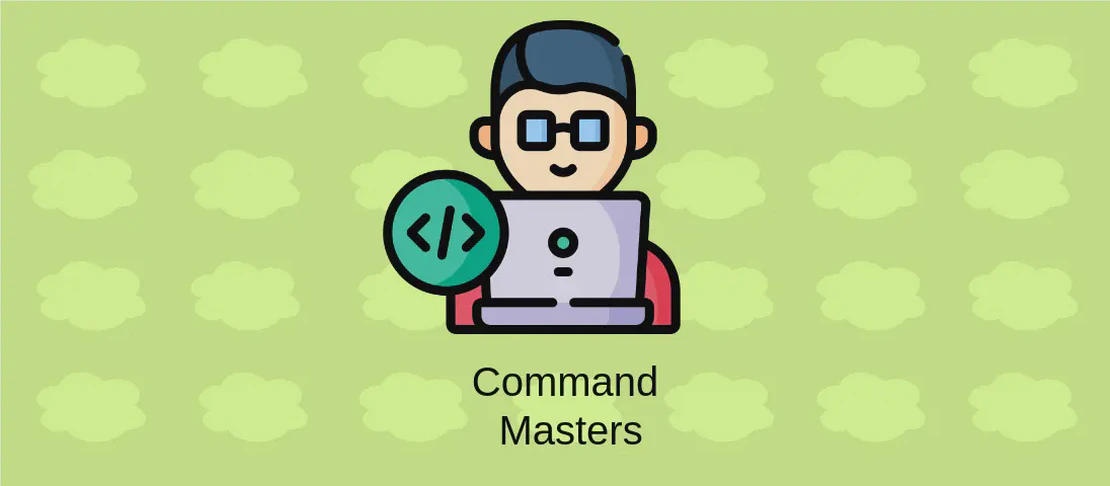
Efficient Haskell Code Improvement with 'hlint' (with examples)
- Linux
- December 17, 2024
‘hlint’ is a powerful command-line tool designed to assist Haskell developers in enhancing the quality of their code. This utility provides insightful suggestions for code improvements, checks code for potential issues, facilitates the automatic application of certain modifications, and even helps in generating personalized settings. With ‘hlint’, creating efficient, readable, and maintainable Haskell code becomes a more streamlined process. Below, we’ll explore various use cases in which ‘hlint’ can be utilized to its full potential.
Use case 1: Display suggestions for a given file
Code:
hlint path/to/file options
Motivation: As a Haskell developer, one often strives to ensure that their code is not only functioning correctly but is also readable and efficient. Hlint becomes invaluable by providing suggestions for improving Haskell code by identifying redundant patterns, recommending stylistic improvements, or pointing out potential errors. Applying hlint to a specific file allows developers to target their improvement efforts where it is needed most.
Explanation:
hlint
: This is the command which invokes the Hlint tool.path/to/file
: This argument specifies the path to the Haskell source file for which you want to receive suggestions. Replacepath/to/file
with the actual file path.options
: Optional arguments that you can include to customize the behavior of Hlint, such as specifying rules or excluding specific checks.
Example Output:
Suggestion: Use a better pattern for the case expression at line 10
Found: case foo of ...
Perhaps you should use: ...
Use case 2: Check all Haskell files and generate a report
Code:
hlint path/to/directory --report
Motivation: When working on a larger Haskell project, manually checking each file for code quality can be tedious and inefficient. This command automates the process, scanning all Haskell files within a specified directory, and compiles a comprehensive report. This report can be extremely useful for code reviews and maintaining overall project quality by addressing issues and implementing code improvements project-wide.
Explanation:
hlint
: The executable command to run the Hlint tool.path/to/directory
: Specifies the directory containing the Haskell files you want to analyze. Replacepath/to/directory
with the actual directory path.--report
: This flag orders Hlint to generate a detailed report, summarizing the findings and suggestions for all files, offering a macro view of the code’s health.
Example Output:
Report generated: 3 files analyzed
2 warnings found
1 suggestion available
See full report at: <URL>
Use case 3: Automatically apply most suggestions
Code:
hlint path/to/file --refactor
Motivation: While suggestions are helpful, implementing them manually can be a repetitive task, which is where automatic refactoring comes in handy. Using Hlint’s ability to apply most of its actionable suggestions directly, developers can rapidly elevate their code quality, saving time and ensuring consistency in code style.
Explanation:
hlint
: Command to start the Hlint tool.path/to/file
: Represents the specific Haskell file you wish to automatically refactor.--refactor
: This option triggers hlint to apply the suggestions it can to modify the file directly, streamlining the process of improvement.
Example Output:
Appended suggestions directly to file.hs
Resolved 2 issues with auto-refactor
Use case 4: Display additional options
Code:
hlint path/to/file --refactor-options
Motivation: For developers seeking more control over the refactoring process or needing to understand various refactor strategies available, this command provides a detailed list of options. Understanding these options can empower developers to customize their usage of hlint, allowing them to choose which suggestions to refactor.
Explanation:
hlint
: Initiates the Hlint tool.path/to/file
: Indicates the file for which you seek to display refactor options.--refactor-options
: A flag used to produce a list of additional options and customizable parameters available during refactoring processes.
Example Output:
Available refactor options:
--refactor-on-unused-imports
--refactor-on-simple-patterns
...
Use case 5: Generate a settings file ignoring all outstanding hints
Code:
hlint path/to/file --default > .hlint.yaml
Motivation: Sometimes, it’s necessary to generate a custom configuration that ignores current suggestions, especially when integrating with a specific codebase where current practices must be maintained initially. This command creates a settings file that outlines these exceptions, which can then be incrementally improved over time without overwhelming the developers with initial suggestions.
Explanation:
hlint
: Runs the tool to analyze the file.path/to/file
: Indicates the file for which you’d like to create default settings.--default
: Instructs hlint to produce a default settings file.> .hlint.yaml
: Redirects the output, which is the default settings, into a file named.hlint.yaml
.
Example Output:
.hlint.yaml settings file created to ignore current suggestions
Customize settings to adapt hlint rules
Conclusion
‘Hlint’ is an essential tool for Haskell developers aiming to maintain high-quality code through actionable insights and automation. By employing its various commands as demonstrated, developers can efficiently polish their code, ensuring both correctness and readability. Hlint not only facilitates immediate improvements but also aids in long-term code maintenance, making it an indispensable part of the Haskell development toolkit.